Python Full Stack Developer Course Syllabus
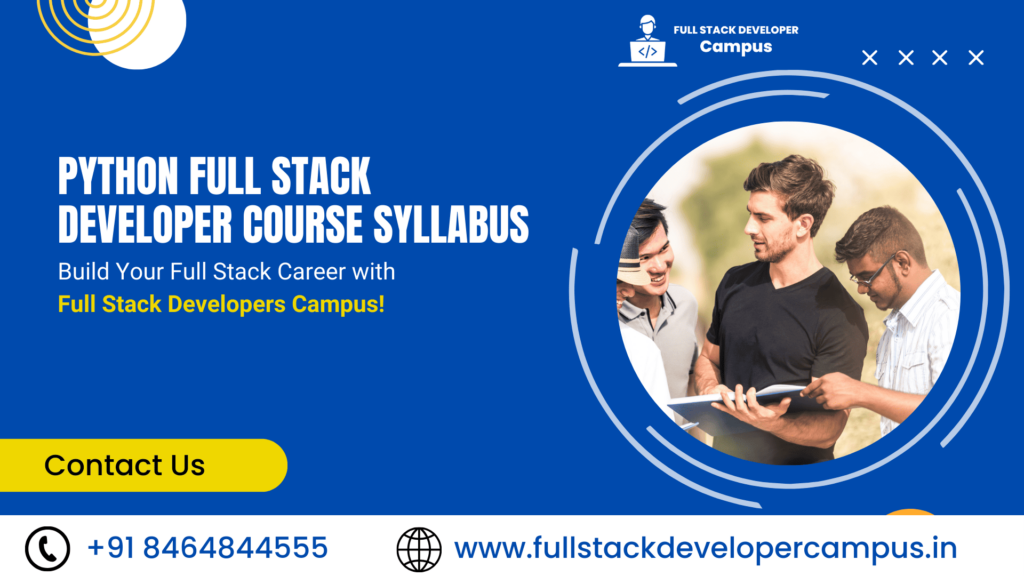
1. Python Full Stack Developer Course Syllabus
Definition: Full Stack Development covers both the front-end and back-end aspects of web development.
Role of a Full Stack Developer: A developer skilled in handling both client-side and server-side development.
Technologies Involved: Front-end technologies like HTML, CSS, JavaScript, and back-end technologies like Python.
Importance of Full Stack Developers: They can manage all layers of development, making them versatile and valuable in tech projects.
Python’s Role: Python is primarily used in back-end development, handling data processing, user authentication, and server-side logic.
Web Stack Overview: The stack refers to the combination of front-end and back-end technologies.
2. Understanding Web Development
Front-End vs. Back-End: Front-end deals with what users interact with, while back-end handles data processing and storage.
Web Development Layers:
Client-side: HTML, CSS, JavaScript, React, and Angular.
Server-side: Python (Django, Flask), Node.js, Ruby.
Databases: SQL (PostgreSQL, MySQL) and NoSQL (MongoDB).
Web Servers: Responsible for serving web pages and handling user requests.
Browser and Server Interaction: Understanding how data flows from the server to the user’s browser is key.
Front-End Technologies: Focuses on making websites interactive, responsive, and user-friendly.
Back-End Technologies: Deals with business logic, APIs, and managing databases.
End-to-End Development: Full Stack Developers are proficient in both ends, making them key players in building complete web applications.
3. Setting Up Your Development Environment
- Installing Python: Learn how to install and configure Python on your system for development.
- Choosing an IDE: Use IDEs like PyCharm, Visual Studio Code, or Sublime Text for better coding productivity.
- Version Control: Install Git to manage code versions and track changes effectively.
- Setting Up Virtual Environments: Use tools like virtualenv or venv to isolate project dependencies.
- Essential Tools: Text editors, terminal commands, and package managers like pip to manage libraries.
- Framework Installation: Install Python web frameworks like Django or Flask.
- Configuration for Front-End: Set up tools like Node.js, npm, and front-end libraries or frameworks.
Debugging Tools: Learn how to use debugging tools for both front-end and back-end issues.
4. Python Basics for Web Development
Python Syntax: Learn Python syntax, variables, data types, and control structures.
Functions and Modules: Understand functions, parameters, return values, and how to structure Python code into modules.
Error Handling: Learn how to handle errors using try-except blocks in Python.
Data Structures: Master Python lists, dictionaries, sets, and tuples.
Loops and Iteration: Understand for and while loops for repeated tasks.
Working with Libraries: Learn how to import and utilize libraries like requests, json, and math for various functionalities.
File Handling: Learn how to read from and write to files in Python.
Introduction to Python for Web: Learn how to use Python for web-specific tasks like form processing, routing, and database integration.
5. Object-Oriented Programming (OOP) in Python
- Classes and Objects: Understand the concepts of classes and objects in Python.
- Encapsulation: Learn how to hide internal object details and expose only necessary features.
- Inheritance: Create new classes based on existing ones, inheriting their properties and methods.
- Polymorphism: Implement methods in different ways, allowing for flexibility in your code.
- Abstraction: Understand how to simplify complex systems by hiding unnecessary details.
- OOP Principles: Master the four main principles of OOP: Encapsulation, Abstraction, Inheritance, and Polymorphism.
- Real-World Examples: Apply OOP concepts to model real-world scenarios like customer accounts or product catalogs in web applications.
- OOP for Web Development: Learn how to structure your Python web apps using OOP principles for scalability and reusability.
6. Web Frameworks: Introduction to Django and Flask
- Frameworks Overview: Web frameworks help simplify the development process by providing reusable components and tools. They are especially helpful in large-scale applications.
- Django:
- High-level framework: Django is known for its “batteries-included” approach, offering a lot of built-in tools like authentication, ORM, and admin interface.
- Model-View-Template (MVT) Architecture: Django follows this design pattern, helping developers separate concerns and streamline the codebase.
- Security: Django includes robust security features like CSRF protection and secure cookie handling.
- Best for large-scale apps: Django’s features make it ideal for building large, complex web applications.
- Flask:
- Lightweight framework: Flask is more minimalistic compared to Django, giving developers the freedom to choose their tools and libraries.
- Microframework: Flask offers a simple core with extensions to add features like authentication and database management.
- Flexibility: Flask is great for small to medium applications, offering more control over how the app is structured.
- Jinja2 Templating: Flask uses Jinja2 for creating dynamic web pages, making it a powerful tool for rendering HTML with Python.
- Choosing Between Django and Flask: Learn how to choose between the two based on project needs. Django is better for rapid development and complex applications, while Flask offers flexibility for small-scale projects.
7. Frontend Development Basics
- HTML (HyperText Markup Language):
- Structure of a web page: HTML defines the structure of your web page, using elements like headings, paragraphs, and links.
- Semantics: Use proper HTML tags like <article>, <section>, and <footer> for semantic web development.
- CSS (Cascading Style Sheets):
- Styling the page: CSS is used to style and layout web pages, including colors, fonts, margins, and positioning.
- Responsive Design: Learn how to use media queries to create responsive designs that work on different devices (mobile, tablet, desktop).
- Flexbox and Grid Layout: Understand modern layout techniques for creating complex, flexible layouts.
- JavaScript:
- Interactivity: JavaScript enables interactivity, such as form validation, DOM manipulation, and event handling.
- DOM (Document Object Model): Learn how JavaScript interacts with the HTML structure of the page, allowing you to dynamically change content.
- ES6 Features: Master modern JavaScript features like arrow functions, promises, async/await, and destructuring for better code efficiency.
- Front-End Libraries/Frameworks:
- React: Learn React, a popular front-end library for building dynamic user interfaces with reusable components.
- Vue.js: Explore Vue.js, a lightweight and flexible JavaScript framework for building single-page applications.
- Angular: Understand Angular, a powerful framework by Google for building enterprise-level applications.
8. Working with Templates in Django and Flask
- What Are Templates?: Templates allow you to generate dynamic HTML content by embedding Python code within HTML files.
- Django Template Engine:
- Template Syntax: Learn Django’s template language, which allows for variables, loops, and conditionals within HTML.
- Template Inheritance: Use template inheritance to avoid repeating common structures like headers and footers across pages.
- Rendering Templates: Understand how to pass data from views to templates to render dynamic web pages.
- Flask Template Engine (Jinja2):
- Jinja2 Syntax: Jinja2 is Flask’s templating engine, enabling dynamic HTML generation using variables and logic.
- Control Structures: Learn how to use for loops, if statements, and filters in Jinja2 for dynamic content.
- Inheritance: Use template inheritance in Flask to structure your templates efficiently and avoid redundancy.
- Common Use Cases: Both Django and Flask templates are used to display dynamic data, such as user profiles, product listings, or blog posts.
9. Backend Development with Python
- Introduction to Server-Side Development: The back end is responsible for processing requests, managing databases, and ensuring the logic behind user actions is executed properly.
- Python Web Frameworks for Backend:
- Django: Provides an easy-to-use ORM (Object-Relational Mapping) to interact with databases and a built-in admin panel.
- Flask: Offers more control over your back-end setup, allowing you to choose third-party libraries for tasks like authentication and database management.
- Routing:
- URL Mapping: Learn how to map URLs to Python functions (views) that process the request and return a response.
- Dynamic URLs: Use dynamic URL patterns with variables in Django and Flask to handle user-specific data (e.g., user profiles).
- Handling HTTP Requests:
- GET vs. POST: Understand the difference between GET (retrieving data) and POST (sending data) HTTP methods.
- Handling Form Data: Learn how to handle user input from forms and process it in your back-end Python code.
- Interacting with Databases:
- Django ORM: Learn how to use Django’s ORM to interact with databases using Python code, eliminating the need for raw SQL.
- Flask with SQLAlchemy: Use SQLAlchemy in Flask for object-relational mapping, allowing you to query and update databases using Python classes.
10. Databases and Data Modeling
- Relational Databases: Learn how to use relational databases like PostgreSQL and MySQL to store structured data.
- Tables: Data is stored in tables with rows and columns, each column representing an attribute and each row representing an entry.
- SQL Queries: Understand how to write SQL queries to select, insert, update, and delete data in your database.
- Database Design: Understand how to design databases with relationships between tables, including primary and foreign keys.
- Normalization: Learn how to organize data to avoid redundancy and improve data integrity.
- Indexes: Create indexes to optimize query performance.
- Database Models in Django:
- Django ORM: Use Django’s ORM to define models, which are Python classes that map to database tables.
- Migrations: Learn how to manage changes to your database schema using Django’s migration system.
- Database Models in Flask:
- SQLAlchemy: Understand how SQLAlchemy is used to define database models and interact with databases in Flask.
- Flask Migrations: Use Flask-Migrate to handle database migrations in Flask projects.
11. APIs and RESTful Services
- What is an API?: An API (Application Programming Interface) allows different software systems to communicate with each other. It defines the methods and data formats that applications can use to interact.
- REST (Representational State Transfer): REST is an architectural style for building APIs. It uses standard HTTP methods like GET, POST, PUT, DELETE, and PATCH to interact with resources.
- Why RESTful APIs?:
- Stateless Communication: Each request from a client to the server must contain all necessary information (no session state is stored on the server).
- HTTP Methods: Use GET to retrieve data, POST to create data, PUT/PATCH to update data, and DELETE to remove data.
- Resource-Based: Resources (such as user accounts, products, or posts) are identified by URLs.
- Django Rest Framework (DRF):
- Serializers: DRF provides serializers to convert complex data types like Django models into JSON, which can be sent over the network.
- ViewSets and Routers: These allow you to quickly build APIs without manually writing view functions for each endpoint.
- Authentication and Permissions: Use DRF’s built-in tools to implement authentication (e.g., token-based authentication) and control access with permissions.
- Building APIs with Flask:
- Flask-RESTful: A lightweight library for Flask that simplifies building REST APIs.
- Flask-SQLAlchemy: Integrates SQLAlchemy with Flask to provide database access in your API.
- Flask-JWT: Implement JSON Web Tokens (JWT) for securing your API and managing user authentication.
- Testing APIs: Learn how to test your APIs using tools like Postman or Insomnia to ensure that endpoints are working as expected.
12. Authentication and Authorization
- Authentication vs Authorization:
- Authentication: Verifies who the user is (e.g., username/password, token-based login).
- Authorization: Determines what a user can do (e.g., read, write, delete based on user roles).
- User Authentication in Django:
- Django Authentication System: Django has built-in tools for user login, registration, and session management.
- Password Hashing: Django automatically hashes passwords to ensure secure storage in the database.
- Django Login and Logout: Implement secure login/logout functionality using Django’s authentication views.
- Email Verification: Set up email confirmation links to verify user email addresses.
- User Authentication in Flask:
- Flask-Login: Use Flask-Login for managing user sessions, including handling login, logout, and user sessions.
- Flask-Security: Integrate Flask-Security for handling authentication, authorization, roles, and permissions.
- OAuth: Learn how to implement third-party login systems, such as Google or Facebook login, using OAuth.
- Role-Based Access Control (RBAC):
- User Roles: Assign different roles to users (e.g., admin, user, guest) to control what parts of the application they can access.
- Permissions: Define permissions for each role, such as allowing admins to access the admin dashboard but restricting regular users.
- Token-Based Authentication:
- JWT (JSON Web Tokens): Securely authenticate users using JWT tokens that are passed in HTTP headers.
- Refresh Tokens: Implement refresh tokens to keep users logged in without requiring them to constantly re-authenticate.
13. Version Control with Git and GitHub
- What is Git?: Git is a distributed version control system that allows multiple developers to collaborate on a codebase. It tracks changes to files and helps you manage project history.
- Basic Git Commands:
- git init: Initializes a new Git repository.
- git clone: Clones an existing repository to your local machine.
- git add: Adds changes to the staging area.
- git commit: Commits changes to the repository with a message.
- git push: Pushes local commits to a remote repository (like GitHub).
- git pull: Pulls the latest changes from the remote repository to your local machine.
- Branching:
- git branch: Creates a new branch to work on features or fixes separately.
- git checkout: Switches between branches.
- Merging: Combine changes from different branches into the main branch.
- Handling Merge Conflicts: Learn how to resolve conflicts that occur when changes to the same lines of code are made on different branches.
- GitHub:
- Creating Repositories: Learn how to create and manage repositories on GitHub.
- Pull Requests (PRs): Use PRs to propose changes and review code before merging it into the main project.
- Collaborating with Teams: Share code with other developers, track issues, and work on projects collaboratively using GitHub’s issues and project boards.
- Git Best Practices:
- Commit Often, Commit Early: Keep commits small and focused on specific changes.
- Write Descriptive Commit Messages: Always provide clear and concise commit messages to explain what was changed and why.
- Use Branches: Use branches for features, fixes, and releases to keep the main branch stable.
14. Testing Your Code
- Why Testing Is Important: Testing ensures that your application works as expected and helps prevent bugs. It is vital for creating maintainable and reliable applications.
- Types of Testing:
- Unit Testing: Test individual components of your code in isolation. For example, check if a function correctly handles input and returns the expected output.
- Integration Testing: Test the interaction between different parts of the application, such as checking if the database and views work together.
- Functional Testing: Test the overall functionality of the application, including features like user registration, login, and form submission.
- End-to-End Testing: Test the entire application flow, simulating real user interactions from start to finish.
- Testing Frameworks:
- Django’s Test Framework: Use Django’s built-in testing tools to write unit and integration tests. Django automatically sets up a test database for isolated testing.
- PyTest: A powerful testing framework for Python that works well with Django and Flask for unit and integration testing.
- Mocking: Learn how to mock external services (like APIs or databases) during testing to isolate code functionality.
- Test-Driven Development (TDD): Adopt TDD practices by writing tests before writing code to ensure your functions are testable and behave as expected.
- Continuous Integration: Set up CI tools like GitHub Actions or Jenkins to automatically run tests whenever you push code changes.
15. Deployment of Full Stack Applications
- Why Deployment subject: Deployment makes your application available to users over the internet. It involves setting up servers, configuring databases, and handling security.
- Cloud Deployment Services:
- Heroku: A platform-as-a-service (PaaS) that makes it easy to deploy Django and Flask applications. Learn how to set up your application on Heroku using Git.
- AWS (Amazon Web Services): A cloud service offering more control over server configuration and scalability. Learn how to deploy using EC2 instances and RDS databases.
- DigitalOcean: A cloud hosting provider ideal for smaller projects and simple app deployments. Use DigitalOcean droplets for scalable server instances.
- Setting Up a Web Server:
- Gunicorn: A Python WSGI server used to run Python web apps in production. Learn how to configure Gunicorn with Django or Flask.
- Nginx: Use Nginx as a reverse proxy to serve your application and handle static files.
- SSL/TLS: Set up SSL certificates (HTTPS) to secure your application with encryption.
- Continuous Deployment: Automate your deployment process using CI/CD pipelines, where new code is automatically tested and deployed to your live environment.
- Environment Variables: Learn how to securely store and manage sensitive data (like API keys and database credentials) using environment variables in production.
- Monitoring and Logging: Set up tools like Loggly or Sentry to monitor app performance and log errors for easy debugging.
16. Handling Errors and Debugging
- Why Error Handling Is Important: Effective error handling ensures your application remains stable and user-friendly, even when something goes wrong. It also helps you track down bugs early in the development process.
- Common Error Types:
- Syntax Errors: Issues in the structure of your code (e.g., missing parentheses or typos).
- Runtime Errors: Errors that occur when your program is running (e.g., dividing by zero or accessing an undefined variable).
- Logic Errors: When the code runs but doesn’t produce the expected results due to a flaw in logic (e.g., incorrect calculations).
- Debugging Techniques:
- Print Statements: A simple yet effective method to check variable values and flow of execution.
- Python’s Debugger (pdb): Use Python’s built-in pdb module to step through your code, inspect variables, and find where things are going wrong.
- IDEs with Debugging Tools: IDEs like PyCharm and Visual Studio Code offer powerful debugging tools that allow you to set breakpoints and inspect variables visually.
- Error Handling in Python:
- try-except Blocks: Python’s try-except blocks allow you to catch exceptions and handle them gracefully, ensuring your application doesn’t crash unexpectedly.
- Custom Exception Classes: You can create custom exceptions to raise specific error messages when certain conditions occur.
- Logging:
- Python Logging Module: Use Python’s logging module to log critical events in your application, making it easier to trace issues that occur in production.
- Logging Levels: Learn how to use different logging levels, like DEBUG, INFO, WARNING, ERROR, and CRITICAL, to categorize the importance of logs.
- Handling Errors in Web Frameworks:
- Django Error Handling: Django provides error views and custom error templates (e.g., 404, 500) that you can modify to provide better user experience during errors.
- Flask Error Handling: Flask allows you to set custom error handlers using decorators to return specific messages or pages for different error codes.
17. Optimizing Web Applications
- Why Performance subject matter: Optimizing your web application is essential for providing a fast, responsive user experience and reducing server load.
- Front-End Optimization:
- Image Optimization: Compress images using formats like WebP and SVG to reduce load times while maintaining quality.
- Minification: Minify CSS, JavaScript, and HTML files to reduce file size and improve page load times.
- Lazy Loading: Load images and other content only when they are visible on the user’s screen, reducing initial load time.
- Browser Caching: Use browser caching to store resources like images and scripts locally on users’ devices, speeding up repeated visits.
- Back-End Optimization:
- Database Indexing: Index frequently queried database columns to speed up search operations.
- Database Query Optimization: Optimize database queries by reducing the number of joins, using SELECT statements for only required fields, and using proper pagination.
- Caching: Implement caching mechanisms like Redis or Memcached to store frequently accessed data in memory, reducing database load and improving response time.
- Code Profiling: Use Python’s cProfile or third-party tools like Py-Spy to identify performance bottlenecks in your application’s code.
- Load Testing: Use tools like Apache JMeter or Locust to simulate traffic and identify potential performance issues under load.
- Asynchronous Programming:
- Asyncio: Python’s asyncio module enables asynchronous programming, allowing non-blocking I/O operations and improving the performance of web applications handling many requests.
- Celery: For heavy background tasks (like sending emails), use Celery to handle them asynchronously, keeping the main thread responsive.
18. Security in Full Stack Development
- Importance of Web Security: As cyber threats become more sophisticated, securing your web applications is crucial to protect sensitive user data and maintain trust.
- Common Security Threats:
- SQL Injection: Attackers can manipulate SQL queries to access or modify your database. Mitigate this risk by using parameterized queries and Django ORM.
- Cross-Site Scripting (XSS): Malicious scripts can be injected into your app, potentially stealing user data. Protect against XSS by escaping user input and using safe rendering techniques in Django and Flask.
- Cross-Site Request Forgery (CSRF): CSRF attacks trick users into performing actions without their knowledge. Use Django’s CSRF middleware or Flask extensions to protect your forms from CSRF attacks.
- Session Hijacking: Attackers can steal session cookies and impersonate users. Use secure cookie flags and session expiration to mitigate this risk.
- Data Encryption:
- SSL/TLS: Secure your website with HTTPS using SSL/TLS certificates to encrypt data between the server and the client.
- Password Hashing: Use hashing algorithms like bcrypt or Argon2 to store passwords securely, ensuring they are not saved as plain text.
- Authentication Security:
- Multi-Factor Authentication (MFA): Add an extra layer of security by requiring users to verify their identity through multiple means (e.g., SMS, email, or app-generated codes).
- JWT Security: When using JWT tokens for authentication, ensure proper token expiration, secure storage, and signing.
- Django Security Features:
- Security Middleware: Django comes with built-in security middleware to prevent common vulnerabilities like clickjacking, cross-site scripting, and insecure password storage.
- Secure Headers: Implement security headers like Content Security Policy (CSP), HTTP Strict Transport Security (HSTS), and X-Content-Type-Options to protect against attacks.
- Flask Security:
- Flask-Security: Use Flask-Security for secure user authentication, password hashing, and role-based access control.
- Flask-SSLify: Force HTTPS connections to ensure secure communication.
19. Working with Real-Time Data
- What is Real-Time Data?: Real-time data refers to information that is delivered immediately after collection without significant delay, essential for features like messaging, notifications, or live feeds.
- WebSockets:
- WebSocket Basics: WebSockets provide full-duplex communication channels over a single, long-lived connection. They’re ideal for real-time applications like chat apps, live notifications, or collaborative tools.
- Django Channels: Use Django Channels to extend Django’s capabilities to handle WebSockets and asynchronous tasks.
- Flask-SocketIO: Flask-SocketIO enables real-time communication in Flask applications using WebSockets.
- Pusher: A service that allows you to implement real-time features like push notifications, live updates, and activity streams in your web apps with minimal effort.
- Polling vs. WebSockets:
- Polling: Regularly checking for updates from the server at fixed intervals. It’s easier to implement but less efficient compared to WebSockets.
- WebSockets: A more efficient, persistent connection that allows immediate data transfer between client and server.
- Use Cases for Real-Time Data:
- Live Chat: Implement a real-time messaging system where users can send and receive messages instantly.
- Real-Time Notifications: Push notifications to users when events occur (e.g., new comments, new messages).
- Live Updates: Display live updates, such as stock prices or sports scores, without needing the user to refresh the page.
20. Structure a Capstone Project
- Project Overview: The capstone project is your opportunity to apply everything you’ve learned throughout the course. It should be a comprehensive, full-stack web application that includes both front-end and back-end components.
- Project Planning:
- Define Your Idea: Choose an interesting project idea that showcases your skills. This could be anything from an e-commerce site, a social media platform, or a task manager.
- Break It Down: Break your project into smaller tasks, including front-end design, back-end logic, database design, and deployment.
- Designing the Application:
- Wireframes: Create wireframes to map out the design and flow of your web application.
- Database Schema: Plan your database schema, including tables, relationships, and fields.
- Implementation:
- Frontend: Use HTML, CSS, JavaScript, and your chosen front-end framework (e.g., React) to create a responsive, user-friendly interface.
- Backend: Use Django or Flask to handle routing, logic, and database management.
- API Integration: If needed, integrate third-party APIs (e.g., payment gateways, weather services).
- Testing and Debugging: Thoroughly test your application, fixing bugs and improving performance where needed.
- Deployment: Deploy your application to platforms like Heroku, AWS, or DigitalOcean, making it accessible online.
- Documentation: Write clear documentation for your project, explaining its functionality, setup, and usage.
Conclusion
This Python Full Stack Developer course will teach you how to build both the front and back parts of websites. Here’s what you’ll learn:
- Python basics: The foundation for creating the logic behind web apps.
- Web development with Django and Flask: Tools for building the back-end (server side) of websites.
- Frontend skills: Learn HTML, CSS, and JavaScript to create interactive web pages.
- Database management: Use SQL and Django ORM to store and retrieve data in your app.
- Version control with Git: Track changes in your code and work with others.
- Security practices: Protect your app from common online threats.
- API development: Connect your app to other services and add real-time features.
- Deployment: Learn to make your app available online with platforms like Heroku and AWS.
By working on real projects, you’ll practice Structure, testing, and launching your own web apps. At the end of the course, you’ll be ready to create full-stack web applications.
As a Python Full Stack Developer, you’ll have many job opportunities because companies need developers who can manage both the front-end and back-end of websites. This course will help you grow your technical skills, problem-solving abilities, and creativity.
FAQs
What is a Python Full Stack Developer?
- A Python Full Stack Developer is someone who can create both the front-end (what users see) and back-end (the server-side) of a website using Python.
What will I learn in this course?
- You’ll learn Python for back-end development, HTML, CSS, and JavaScript for the front-end, and how to work with databases.
Do I need to know coding before taking this course?
- It’s helpful to have basic knowledge, but the course can teach you everything you need, even if you’re new to coding.
What are the basic requirements to take this course?
- You should have an interest in learning programming, and some basic knowledge of how websites work would be useful.
How long does it take to finish the course?
- The course usually takes around 3 to 6 months, depending on whether it’s full-time or part-time.
What will I learn for front-end development?
- You’ll learn to design websites using HTML, CSS, and JavaScript, and make them interactive with tools like React or Angular.
What will I learn for back-end development?
- You’ll learn to create server-side applications using Python with Django or Flask and connect to databases.
Will I learn about databases?
- Yes, you’ll learn to work with databases like MySQL or MongoDB to store and manage data.
What is Django?
- Django is a tool in Python that helps build web applications quickly and securely. It’s mainly used for back-end development.
What is Flask?
- Flask is another Python tool for web development, but it is simpler and gives you more control over how your website works.
What tools will I use during the course?
- You’ll use coding software like Visual Studio Code and tools like Git for version control and Heroku for deploying websites.
Do I need to know JavaScript?
- Yes, JavaScript is needed for front-end development, as it helps make websites interactive.
What is a RESTful API, and will I learn to build one?
- Yes, you will learn how to create APIs that let different apps talk to each other.
Do I get a certificate after completing the course?
- Yes, most courses give a certificate that you can add to your resume.
Can I get a job after finishing this course?
- Yes, after completing the course and building a good portfolio, you can apply for jobs as a Python Full Stack Developer.