Mern Stack Developer Roadmap
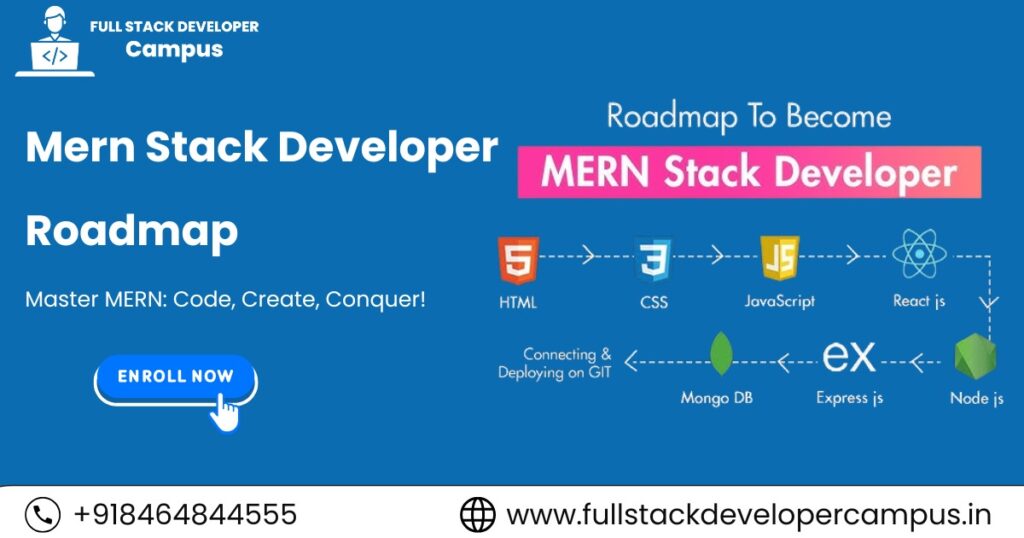
1. What is the MERN Stack Developer Roadmap
The MERN stack is one of the most popular technology stacks for web development, consisting of MongoDB, Express.js, React.js, and Node.js. Here’s a breakdown of what you should learn and the steps to take on your journey to becoming a proficient MERN Stack Developer:
The MERN stack is composed of four primary technologies:
MongoDB: A NoSQL database that stores data in JSON-like format, which is ideal for handling large volumes of unstructured or semi-structured data. Unlike traditional relational databases, MongoDB uses collections of documents instead of tables and rows. MongoDB’s flexibility allows you to store data in a format that is easy to scale as your application grows.
Key Concepts: Collections, Documents, CRUD operations, Aggregation Pipeline, and Data Indexing.
Express.js: Express is a fast, minimalistic, and flexible web application framework for Node.js. It simplifies server-side development by providing a wide range of features such as routing, middleware integration, request handling, and more.
Key Concepts: Routing, Middleware, HTTP Methods, Error Handling, Request and Response objects.
React: React is a declarative JavaScript library for building user interfaces, developed by Facebook. It allows developers to create interactive UIs with reusable components. React also provides virtual DOM that optimizes rendering and improves performance.
Key Concepts: Components, State, Props, JSX, Virtual DOM, Hooks (useState, useEffect), Component Lifecycle, React Router.
Node.js: Node.js is a server-side JavaScript runtime built on Chrome’s V8 JavaScript engine. It allows developers to use JavaScript for building fast and scalable applications. Node.js is known for its non-blocking, event-driven architecture, which makes it well-suited for building real-time applications.
Key Concepts: Event Loop, Asynchronous I/O, npm, Express Integration, Node.js Streams.
Together, the MERN stack allows developers to seamlessly build and deploy full-stack applications, enabling quick iteration cycles and smooth development workflows.
2 Requirement Before You Start
Before diving into MERN development, it is essential to have a solid understanding of the following foundational technologies:
HTML, CSS, and JavaScript Basics:
HTML provides the basic structure for web pages, CSS is used for styling, and JavaScript adds interactivity. A good understanding of these languages is essential before you move on to advanced topics.
Learn about semantic HTML, CSS Flexbox/Grid for layout design, and modern JavaScript features like ES6 (e.g., arrow functions, destructuring, classes, and promises).
Git and GitHub:
Git is a version control system that tracks changes to code over time. GitHub provides an online platform to host and collaborate on Git repositories.
Essential commands to learn: git init, git clone, git commit, git push, git pull, and git branch.
Command Line Basics:
Familiarity with basic terminal commands like cd (change directory), ls (list files), mkdir (make directory), touch (create a file), and npm (Node Package Manager) is important for managing your projects.
HTTP and REST APIs:
Understanding how the web works (requests, responses, status codes, headers, etc.) is key to backend development. Learn about the principles of RESTful APIs and how to design APIs that handle CRUD operations (Create, Read, Update, Delete).
Familiarize yourself with tools like Postman for testing APIs.
3. Core Concepts to Master
In this section, we’ll dive into the core technologies of the MERN Stack Developer Roadmap. Each of the four components comes with a unique set of features that must be mastered to build a well-functioning application.
MongoDB
CRUD Operations: Learn how to create, read, update, and delete documents in a MongoDB collection. MongoDB uses its own querying language, but it is based on JSON syntax, making it easier for JavaScript developers to grasp.
Mongoose: Use Mongoose for object data modeling (ODM) in Node.js. It allows you to define schemas, validate data, and provide methods to interact with MongoDB.
Indexes and Aggregation: Learn how to create indexes to speed up queries and use the aggregation pipeline to perform advanced data processing.
Express.js
Routing: Understand how to define routes for different HTTP methods (GET, POST, PUT, DELETE) and how to handle URL parameters and query strings.
Middleware: Middleware functions in Express are pieces of code that run between receiving a request and sending a response. They are used for tasks like logging, authentication, error handling, and request parsing.
Building RESTful APIs: Express simplifies the creation of RESTful APIs, where you can define endpoints for performing CRUD operations on resources (e.g., user data, blog posts).
React
Components: Everything in React is a component. Components are reusable pieces of code that manage their own state and render user interface elements. They can be functional or class-based.
State and Props: Learn how data flows in React using props (inputs from parent to child components) and state (internal data for a component).
Hooks: React hooks like useState, useEffect, and useContext make functional components more powerful, allowing them to have local state and side effects.
React Router: Use React Router to handle client-side routing in your single-page application (SPA). It allows you to navigate between different views without reloading the page.
Node.js
Asynchronous Programming: Node.js uses asynchronous, non-blocking I/O, which means that tasks like reading files or querying a database don’t block the execution of the rest of the program. Learn how to use callbacks, Promises, and async/await to handle asynchronous operations.
npm: Use npm to install dependencies and manage libraries. Learn about common libraries like express, mongoose, and dotenv.
Building RESTful Backends: Understand how to set up a Node.js server with Express, and handle API requests (GET, POST, PUT, DELETE) for a full-fledged back-end.
4. Setting Up the MERN Stack Development Environment
To begin building with MERN, you need to set up the development environment:
Node.js and npm: Install Node.js from the official website, which comes with npm (Node Package Manager).
MongoDB: You can either install MongoDB locally or use MongoDB Atlas, a cloud-based service for managing MongoDB databases.
Text Editor: Use VS Code (Visual Studio Code), a lightweight and feature-rich editor for web development.
Terminal: Learn how to run npm commands and use the command line to manage your development server.
Once the environment is set up, you can start building your MERN application.
5. structure Your First MERN Stack Application
Create a simple To-Do List Application or a CRUD App that will help you put everything together:
Backend: Use Express.js to create routes for creating, updating, and deleting to-do items. Use MongoDB to store the to-do list items.
Frontend: Use React to create a dynamic user interface where you can add, view, and delete items. Use Axios or fetch to make HTTP requests to the Express API.
Connecting Backend and Frontend: The React app will make API calls to the Express backend, which will interact with MongoDB to store and retrieve the data.
Authentication (Optional): You can add user authentication using JWT or sessions, where users can sign up, log in, and have their own private to-do lists.
6. Advanced Topics to Explore
Once you’re comfortable with the basics, you can dive into more advanced topics to enhance your MERN stack knowledge:
Authentication & Authorization: Implement JWT (JSON Web Tokens) or OAuth to secure user accounts and manage sessions.
Real-Time Applications: Use WebSockets with Socket.io to build real-time chat applications or live notifications.
GraphQL: Learn how to use GraphQL as an alternative to REST APIs. GraphQL allows clients to query exactly the data they need, reducing over-fetching.
Testing: Write unit tests using Jest for front-end components and Mocha/Chai for back-end logic.
Deployment: Learn how to deploy MERN applications on platforms like Heroku, Netlify, or AWS for production-ready apps.
7. Best Practices for MERN Stack Developers
To build scalable, maintainable, and secure MERN applications:
Code Organization: Keep a clean and modular structure. Divide your code into smaller files and components for easier maintenance and collaboration.
Security: Implement security best practices like input validation, password hashing (bcrypt), and using helmet.js for securing HTTP headers.
Optimization: Optimize performance by enabling lazy loading in React, and caching frequent database queries in MongoDB.
Error Handling: Implement global error handling in Express and React to prevent your application from breaking unexpectedly.
8. Debugging and Error Handling
As your application grows in complexity, debugging and handling errors effectively becomes crucial. Here’s what you need to know:
Node.js Debugging: Learn how to debug Node.js applications using node –inspect or integrated debuggers in code editors like VS Code. Setting breakpoints and stepping through your code will help you find issues in the back-end code efficiently.
React Debugging: Use React Developer Tools in the browser to inspect React components, their state, and props. This tool helps you track state changes and pinpoint issues in your UI.
Error Handling in Express: Implement centralized error handling by creating custom error-handling middleware in Express. This ensures that your app responds gracefully to unexpected errors and provides meaningful error messages to the user.
Logging: Use winston or morgan for logging in Node.js to track requests, errors, and other useful information in production environments. Proper logging will help you diagnose problems more effectively.
9. Version Control and Collaboration
Effective use of version control and collaboration is key to developing scalable projects in a team or individually. Here’s how you can master it:
Git Workflow: Learn the fundamentals of version control with Git. Use commands like git commit, git push, git pull, and git merge to track changes, revert to previous versions, and collaborate with others. Adopt a branching strategy (such as Gitflow) for better management of features, bug fixes, and releases.
Pull Requests and Code Reviews: In team environments, use pull requests (PRs) to propose and review changes before merging them into the main codebase. Conduct thorough code reviews to ensure code quality, readability, and maintainability.
GitHub for Collaboration: Host your code repositories on platforms like GitHub to collaborate with others, contribute to open-source projects, and showcase your work. Learn to manage issues, track bugs, and use GitHub Actions for Continuous Integration (CI) and Continuous Deployment (CD).
10. Performance Optimization and Scalability
Building applications that can scale and perform well under heavy load is crucial for production apps. Here are some techniques:
React Performance Optimization:
Memoization: Use React’s React.memo and useMemo hooks to prevent unnecessary re-renders.
Lazy Loading: Implement lazy loading for components using React.lazy and Suspense to load components only when they’re needed.
Code Splitting: Split your React app into smaller chunks using dynamic imports to optimize loading times.
Server-Side Optimization:
Caching: Use caching strategies like Redis to store frequently accessed data and reduce database load.
Database Indexing: Ensure your MongoDB collections are properly indexed to optimize read and write performance.
Load Balancing: If your app needs to scale, use load balancing techniques (e.g., with NGINX) to distribute traffic across multiple servers.
Front-End Optimization:
Minimize and bundle your JavaScript and CSS files to reduce loading time.
Use image optimization techniques and Content Delivery Networks (CDNs) for faster static content delivery.
Horizontal Scaling: Design your application to handle more traffic by scaling out (adding more servers) rather than scaling up (increasing server size).
Summary of Added Sections:
Debugging and Error Handling: Learning to efficiently debug your MERN stack application and implement error handling will ensure your application is stable and error-free.
Version Control and Collaboration: Mastering Git and GitHub workflows will enable you to collaborate effectively with teams and manage code versions efficiently.
Performance Optimization and Scalability: Understanding optimization techniques will help you build high-performing applications capable of scaling smoothly as user demand increases.
11. Authentication and Authorization
Implementing proper user authentication and authorization ensures that your app is secure and user data is protected. Here’s how to handle it effectively:
User Authentication: Use JWT (JSON Web Tokens) or OAuth for secure authentication. JWT is widely used to authenticate users in MERN apps by creating a token after the user logs in. The token is then used to authorize subsequent requests.
Password Hashing: Never store passwords in plain text. Use bcrypt to hash passwords before storing them in MongoDB. This ensures that even if the database is compromised, user passwords remain secure.
Role-based Authorization: Implement role-based access control (RBAC) in your app to manage user permissions based on their roles (e.g., admin, user). This is crucial for protecting routes and data in your application.
Session Management: For apps with persistent login sessions, store session data in cookies or localStorage (for React) and manage expiration of sessions properly.
12. Testing and Test Automation
Testing ensures that your app works as expected and helps catch bugs early. Learn how to automate and write tests for your MERN stack applications:
Unit Testing: Write unit tests for individual functions, components, and methods using libraries like Jest (for React) and Mocha/Chai (for Express). Ensure that each unit of your application behaves correctly in isolation.
Integration Testing: Test the interaction between different parts of your application. For instance, verify that the front-end React components are correctly interacting with the back-end Express API and MongoDB.
End-to-End Testing: Use tools like Cypress or Puppeteer for end-to-end testing to simulate real user behavior. These tests ensure that the entire application stack functions as intended when users interact with the app.
Test Automation: Integrate automated testing in your CI/CD pipeline to ensure continuous testing and prevent regressions as new code is added.
13. Working with APIs (RESTful and GraphQL)
Modern web applications often rely on third-party or internal APIs. Understanding how to work with APIs is critical for MERN developers:
RESTful APIs: Learn to design and build REST APIs that follow proper conventions such as CRUD operations (Create, Read, Update, Delete). Use Express.js for defining routes and handling requests with HTTP methods like GET, POST, PUT, and DELETE.
GraphQL: Learn GraphQL, an alternative to REST, which allows clients to request exactly the data they need, avoiding over-fetching. With Apollo Server for the back-end and Apollo Client for the front-end, you can integrate GraphQL seamlessly into your MERN app.
Consuming External APIs: Learn how to consume third-party APIs (like social media APIs, weather data, or payment processing APIs). Use Axios or fetch in React to make API requests and display the results to users.
Error Handling for APIs: Properly handle errors such as 404 (not found), 500 (server error), and network issues when working with APIs to improve the user experience.
14. DevOps and Continuous Integration/Continuous Deployment (CI/CD)
DevOps practices help automate workflows and streamline deployment pipelines, enabling faster development cycles and reliable deployments. Here’s what you need to know:
Version Control with Git: As mentioned earlier, Git is central to CI/CD workflows. Using GitHub Actions, CircleCI, or Travis CI, you can automatically trigger tests, builds, and deployment pipelines every time you push code to your repository.
Automated Testing: Integrate automated tests (unit tests, integration tests, etc.) into your CI/CD pipeline to ensure that your code is thoroughly tested every time changes are made.
Containerization with Docker: Learn how to containerize your MERN stack application using Docker. Docker allows you to package your application along with its dependencies into a container, making it easier to deploy across different environments (e.g., development, staging, and production).
Deployment Automation: Automate the deployment process using services like Heroku, Netlify, or AWS. Use deployment scripts to automatically deploy your application when tests pass, ensuring faster and more reliable updates.
15. State Management in React
Managing state in React can be tricky, especially as your application grows. Mastering state management tools ensures scalability and maintainability:
React’s Built-in State Management: Learn how to use useState and useReducer hooks to manage local state in your React components. Understand when to lift state up to parent components for shared state management.
Context API: For passing state across multiple components without prop drilling, use React’s Context API. This is ideal for global state management in smaller to mid-sized applications.
Redux: For larger, more complex applications with multiple components requiring shared state, learn Redux. Redux provides a centralized state container and works well with React. Learn core concepts like actions, reducers, and store.
Redux Toolkit: Use the Redux Toolkit to simplify Redux development. It includes pre-configured tools and best practices to manage state in a more efficient and scalable way.
State Management Libraries: Explore other libraries like Recoil or MobX for alternative state management solutions, depending on your project’s needs.
16. Mobile Development with React Native
If you want to extend your MERN stack skills to mobile app development, React Native is a great choice. It allows you to use the same React principles to build cross-platform mobile apps:
React Native Basics: Learn the fundamental differences between web and mobile development in React. React Native uses native components like View, Text, and Button instead of HTML tags.
Building Mobile Apps: Create mobile applications using React Native CLI or Expo, which provides an easier setup for development and testing. Learn to manage navigation, states, and data fetching in mobile apps.
Connecting to MERN Backend: Use your existing MERN stack API (built with Express and MongoDB) to interact with the mobile app. Use Axios or Fetch in React Native to send requests to your Express server and retrieve data.
Deployment and Testing for Mobile: Understand how to test and deploy your mobile apps to the App Store and Google Play Store using Xcode (for iOS) and Android Studio (for Android).
Summary of Additional Sections:
Authentication and Authorization: Master user authentication, password hashing, and role-based access to secure your application.
Testing and Test Automation: Implement unit, integration, and end-to-end testing to ensure your app works correctly and catches bugs early.
Working with APIs (RESTful and GraphQL): Learn to build, consume, and handle APIs (RESTful or GraphQL) to integrate your app with external services.
DevOps and CI/CD: Automate your workflow, testing, and deployment using continuous integration and continuous deployment tools.
State Management in React: Manage application state using React’s built-in tools, Context API, and advanced solutions like Redux or Redux Toolkit.
Mobile Development with React Native: Extend your MERN skills to mobile development by learning how to build cross-platform mobile apps using React Native.
Conclusion
Becoming proficient in the MERN stack is a highly rewarding journey that opens up many opportunities for building modern, scalable, and dynamic web applications.
With MongoDB, Express.js, React, and Node.js, you have a powerful set of tools that can help you create full-stack applications entirely in JavaScript.
By following this roadmap, you have learned the fundamentals of each technology in the stack, starting from the basics to more advanced concepts like state management, testing, performance optimization, and mobile development. You’ve also explored key practices such as version control, debugging, and integrating CI/CD pipelines, which are essential for maintaining high-quality, production-ready code.
As you continue your learning, remember that building real-world projects is crucial. By working on personal or open-source projects, contributing to the developer community, and staying up-to-date with the latest trends, you’ll be able to grow your skills and become a well-rounded MERN stack developer.
Finally, always strive to learn and iterate. The world of web development is constantly evolving, and the best way to stay ahead is through continuous learning, experimenting, and building. Whether you’re developing complex enterprise-level applications or simple personal projects, the MERN stack provides the flexibility and scalability needed to succeed in today’s web development landscape.
FAQs
1. What is the MERN stack?
The MERN stack is a collection of JavaScript-based technologies used for building full-stack web applications. It consists of MongoDB (database), Express.js (web framework), React (front-end library), and Node.js (runtime environment).
2. Why should I learn the MERN stack?
Learning the MERN stack allows you to build full-stack web applications using a single programming language, JavaScript, for both the client and server sides. It’s highly scalable, efficient, and popular for developing modern web applications.
3. Do I need to learn all four technologies in the MERN stack?
While it’s not mandatory, learning all four technologies will give you a well-rounded understanding of how full-stack JavaScript applications work. It will also help you become a more versatile developer.
4. Can I use the MERN stack for mobile app development?
Yes, you can use React Native, a framework built on React, to develop mobile apps. The back-end of your mobile app can still be powered by the MERN stack using Node.js and Express, while MongoDB serves as your database.
5. What is the difference between MongoDB and traditional SQL databases?
MongoDB is a NoSQL database that stores data in a flexible, document-based format (BSON), making it ideal for handling unstructured data. SQL databases, on the other hand, use structured data and predefined schemas in tables.
6. How does React work in the MERN stack?
React is used for building the front-end of the application. It allows developers to create reusable UI components and manage the application’s state efficiently, often with the help of libraries like Redux or Context API.
7. Is Express.js necessary for the MERN stack?
Yes, Express.js acts as the back-end framework of the MERN stack. It simplifies handling HTTP requests, routing, middleware, and integrates seamlessly with Node.js for building RESTful APIs.
8. What is Node.js used for in the MERN stack?
Node.js serves as the runtime environment for executing JavaScript code on the server side. It allows you to build scalable back-end applications and APIs with asynchronous I/O, making it fast and efficient.
9. Can I use other databases with the MERN stack?
Although MongoDB is the primary database in the MERN stack, you can replace it with other databases like PostgreSQL, MySQL, or SQLite. However, this would technically make the stack something like MEAN (MongoDB/Express/Angular/Node.js) or similar.
10. What is the role of middleware in Express.js?
Middleware in Express.js is a function that processes requests and responses before the final handler. It is used for tasks such as error handling, logging, authentication, and modifying request/response objects.
11. How does authentication work in the MERN stack?
Authentication in MERN stack apps is often handled using JWT (JSON Web Tokens) or OAuth. The server validates the user’s credentials and issues a token that must be included in subsequent requests to protected routes.
12. How do I deploy a MERN stack application?
You can deploy MERN stack applications using platforms like Heroku, Netlify, or AWS. The front-end React app can be deployed on Netlify, while the back-end API (Node/Express) can be deployed on Heroku or AWS EC2.
13. What are React hooks, and how are they used in the MERN stack?
React hooks are functions like useState and useEffect that let you use state and lifecycle methods in functional components. They simplify component logic and state management without needing class components.
14. How does state management work in React?
State management in React can be done through useState and useReducer hooks for simple cases. For more complex applications, you can use Redux, Context API, or third-party libraries like Recoil to handle global state across components.
15. What are some common challenges when working with the MERN stack?
Some common challenges include:
- Asynchronous programming: Handling asynchronous code in JavaScript (with callbacks, promises, and async/await) can be tricky.
- State management: Managing complex state in React, especially across multiple components.
- Database design: Structuring your MongoDB database efficiently for scalability and performance.
- API integration: Handling communication between the front-end (React) and back-end (Node/Express) via APIs can be complex.
- Debugging and error handling: Tracing and fixing bugs in full-stack JavaScript applications requires good debugging skills across the entire stack.