Java Beginner Projects
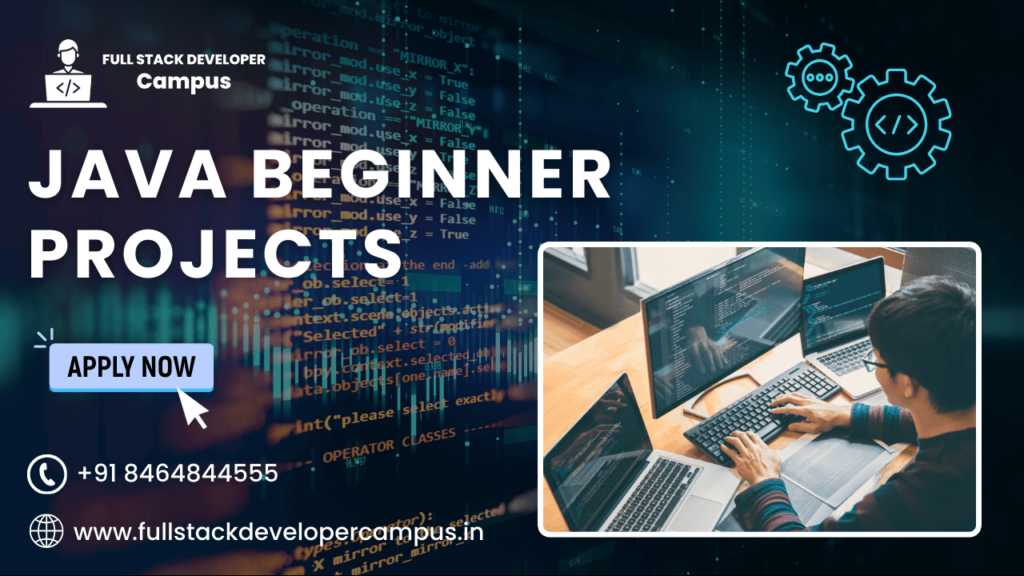
Java is one of the most popular programming languages used by developers worldwide, particularly for beginners due to its readability, scalability, and wide use in various domains. As a beginner in Java, understanding the theoretical concepts is crucial, but hands-on practice through projects is where real learning happens. Projects help solidify the core concepts, provide a sense of achievement, and give you the necessary skills to build your portfolio.
Starting with small and manageable Java projects is the key to building confidence in your programming abilities. These projects allow you to implement what you’ve learned in a practical manner, giving you real-world experience. They can range from simple applications to more complex ones, and each project will enhance your problem-solving skills, deepen your understanding of Java’s syntax, and improve your ability to write efficient code.
By choosing the right beginner-level projects, you’ll be able to gradually grow your knowledge and tackle more advanced concepts as you progress. As a Java beginner, working on projects will help you:
- Understand Java’s Object-Oriented Programming (OOP) principles.
- Improve problem-solving abilities by applying theoretical concepts to practical scenarios.
- Learn how to use IDEs (Integrated Development Environments) effectively.
- Gain experience in debugging and testing code.
- Build a portfolio to showcase your skills to potential employers or collaborators.
Mandatory Concepts for Java Beginners"
Before diving into Java projects, it’s important to have a solid understanding of key Java concepts that form the foundation of programming in this language. These concepts are critical not only for building projects but also for writing clean, efficient, and maintainable code. Here are the essential concepts every Java beginner should master:
- Variables and Data Types
Java is a statically typed language, meaning every variable must be declared with a specific data type (e.g., int, String, boolean). Understanding how variables work and when to use each data type is fundamental. You’ll also need to grasp the concepts of type casting and type conversion as you work with different data types. - Control Structures
Java provides several control structures to manage the flow of your program. These include if, else, switch, and looping constructs like for, while, and do-while. Mastering these will allow you to make decisions and repeat tasks in your programs, which is crucial for building interactive and dynamic applications. - Functions and Methods
Methods in Java define the behavior of your objects and functions. Understanding how to define methods, pass parameters, and return values is essential. Additionally, the concept of method overloading—where you can define multiple methods with the same name but different parameters—can help you optimize your code. - Object-Oriented Programming (OOP)
Java is an Object-Oriented Programming language, so mastering OOP principles is crucial. This includes concepts like classes, objects, inheritance, polymorphism, encapsulation, and abstraction. These principles will help you structure your code in a way that is modular, reusable, and easier to maintain. - Arrays and Collections
Arrays are used to store multiple values in a single variable. However, when you need more flexibility (such as adding or removing elements dynamically), Java provides powerful collection classes like ArrayList, HashMap, and HashSet. Understanding these will allow you to work with data more effectively. - Exception Handling
In real-world applications, things don’t always go as planned. Java’s exception handling mechanism allows you to handle errors gracefully using try, catch, and finally blocks. This is important for building robust applications that can recover from unexpected issues without crashing. - File Handling
Being able to read and write data to and from files is an essential skill for many Java projects. Understanding Java’s file I/O classes (like FileReader, BufferedReader, and FileWriter) will enable you to work with text files and store data persistently. - Basic Algorithms and Problem-Solving
Before starting projects, it’s beneficial to learn basic algorithms and how to solve problems logically. Concepts like sorting, searching, and recursion will improve your ability to write efficient code and tackle more complex projects.
These core concepts serve as the foundation for more advanced programming topics, so take the time to understand them thoroughly. By mastering these basics, you’ll be prepared to take on any Java beginner project and continue building your skills as you go.
Top 5 Java Projects for Beginners to Practice
Once you have a grasp of Java’s basic concepts, it’s time to put your knowledge into practice. Starting with simple projects helps reinforce the skills you’ve learned and builds confidence. Below are five excellent Java projects for beginners that are both educational and enjoyable to work on:
- Simple Calculator Application
A calculator is one of the most common beginner Java projects. This project will teach you the basics of handling user input, performing mathematical operations, and displaying results on the screen. You can start by building a basic calculator that handles addition, subtraction, multiplication, and division. Once you’re comfortable, try expanding the functionality to include more complex operations like square roots or trigonometric functions.
Key Skills Learned:
- Handling user input with Scanner class.
- Using conditional statements (if, else) and loops.
- Implementing methods to structure your program.
- To-Do List Application
A to-do list app is another great project for beginners. It involves creating a simple interface where users can add, remove, and view tasks. This project will help you understand how to work with data structures such as lists or arrays to store and manipulate user tasks. You can also add functionality to store tasks in a file, allowing users to save and load their to-do list.
Key Skills Learned:
- Working with arrays or ArrayList collections.
- File handling for saving and loading data.
- Understanding the use of loops for iterating over tasks.
- Number Guessing Game
A number guessing game involves the program selecting a random number, and the user has to guess it. This simple game teaches you how to use random number generation and basic user input validation. You can also add additional features such as tracking the number of attempts or setting a time limit for the user to guess.
Key Skills Learned:
- Generating random numbers using Math.random() or Random class.
- Validating user input.
- Using loops and conditionals for game logic.
- Basic Banking System
A basic banking system allows users to create an account, deposit and withdraw money, and check their balance. This project introduces the concept of object-oriented programming (OOP) as you can model the system using classes like Account, Transaction, etc. It also helps you understand how to implement methods that manipulate object data.
Key Skills Learned:
- Using OOP principles like classes and objects.
- Implementing methods for account operations.
- Managing simple user input and output.
- Tic-Tac-Toe Game
Tic-Tac-Toe is a classic project that involves creating a game board and allowing two players to take turns marking the spaces with X or O. This project challenges you to think logically about how to represent the game state (such as using a 2D array for the board) and implement game logic that determines when the game is won or tied.
Key Skills Learned:
- Using multi-dimensional arrays to store the game board.
- Implementing logic to check for win conditions.
- Handling user turns and displaying results.
These projects provide an excellent starting point for beginners to practice their Java skills. As you complete each one, you’ll become more comfortable with the language and better equipped to tackle more complex projects in the future.
Choosing the Right Java Project for Your Skill Level
When you’re just starting out with Java, choosing the right project is crucial for maintaining motivation and ensuring that you’re learning in a way that challenges you without overwhelming you. Here’s how you can assess your current skill level and pick projects that will help you grow as a Java developer.
- Start with Simple Projects
As a beginner, you should begin with projects that focus on understanding basic Java concepts such as variables, loops, and conditionals. A simple project, like a number guessing game or a basic calculator, helps you get comfortable with syntax and basic logic without needing to worry about complex topics like object-oriented programming or data structures. - Build Up Gradually
As you gain confidence, you can start working on slightly more complex projects that require using more advanced Java features. A project like a to-do list application or a simple banking system involves working with data structures (like arrays or ArrayLists), handling files, and using methods. These projects will push you to go beyond basic syntax and start structuring your code in a more modular way. - Focus on OOP Concepts
Once you’re comfortable with the basics, start working on projects that introduce object-oriented programming (OOP). A Tic-Tac-Toe game or a simple chat application can help you get hands-on experience with classes, objects, inheritance, and other OOP principles. These projects will teach you how to model real-world problems in code, which is an essential skill for building more advanced applications. - Work on Projects with User Interaction
Projects that require user interaction, like a text-based adventure game or a small inventory system, will help you become more familiar with input handling and program flow. These types of projects often involve additional considerations, such as how to manage user sessions and validate input, which are important aspects of software development. - Consider Long-Term Projects
As you progress in your learning, consider taking on a long-term project that you can build upon over time. A personal blog system, a weather app, or a movie database system are all examples of projects that grow in complexity as you add features. These projects will give you the chance to apply your learning in a real-world context, and by continually improving and refactoring your code, you’ll learn best practices in software development. - Don’t Be Afraid to Experiment
Sometimes, the best way to learn is by experimenting. If you find a project idea that excites you but feels just a little bit beyond your current skill level, don’t hesitate to dive in. You might not know how to complete every step, but the process of troubleshooting and researching solutions is an essential part of growing as a developer.
Remember, the key is to find projects that are challenging but not frustrating. If you’re constantly working on projects that are too easy, you won’t grow. But if you’re tackling projects that are too difficult, you might feel overwhelmed. Find a balance that keeps you engaged and learning, and you’ll see rapid improvement.
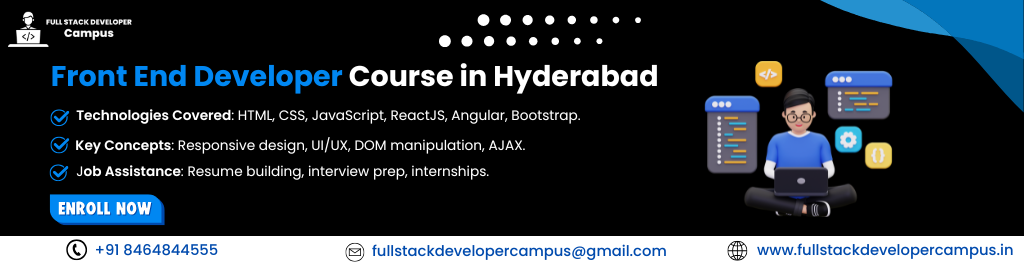
Easy Guide to Building Your First Java Project
Building your first Java project is an exciting learning experience. Here’s a simple, non-technical guide to get you started.
Step 1: Install Java Development Kit (JDK)
To start working with Java, you need to install the Java Development Kit (JDK), which provides tools for developing Java applications. Download and install the latest JDK from Oracle or an open-source version like OpenJDK. After installation, make sure the environment variables are set up correctly.
Step 2: Choose Your Development Environment
While you can write Java code in any text editor, using an Integrated Development Environment (IDE) is recommended. IDEs like IntelliJ IDEA, Eclipse, or NetBeans provide helpful features like code suggestions, debugging tools, and project organization. Choose one that suits you best and install it.
Step 3: Create a New Java Project
Once your IDE is set up, create a new Java project. Most IDEs allow you to set up a project with just a few clicks, automatically creating necessary files and folders to organize your code.
Step 4: Write Your First Program
In your new project, create a Java class with a main method. This is the entry point of your program. A simple program might print a message to the console. This is a great way to test if everything is set up correctly.
Step 5: Run Your Program
Use the IDE’s built-in tools to run your Java program. After running, you should see the output in the console, such as a greeting or any message you’ve written. This confirms that your development environment and Java installation are working.
Step 6: Learn Basic Java Concepts
As you continue building your project, explore basic Java concepts like variables, conditionals, loops, and methods. These building blocks will help you develop more complex programs.
Step 7: Organize Your Project
As your project grows, you may want to organize it into packages. Packages help keep your code structured and easier to manage. It’s also important to use proper naming conventions for your files and classes.
Step 8: Add External Libraries (Optional)
For more complex projects, you may need to include additional libraries. Tools like Maven or Gradle can manage dependencies for you, making it easier to integrate external libraries into your project.
Step 9: Test Your Code
Testing your code is a crucial step in software development. Write simple test cases to verify that your program behaves as expected. Most IDEs provide built-in testing tools to help you with this.
Step 10: Package and Distribute Your Project
Once your project is complete, you can package it as a JAR (Java Archive) file. This allows you to distribute your program to others, who can run it on any machine with Java installed.
Common Java Beginner Challenges & Solutions
When starting with Java, beginner projects often encounter challenges that can feel overwhelming. However, understanding these obstacles and knowing how to tackle them can make your learning journey smoother and more enjoyable. Here are some of the most common challenges and strategies to overcome them:
1. Understanding Syntax and Error Messages
Java’s syntax can be intimidating for beginners, especially when encountering errors like missing semicolons, incorrect curly braces, or misunderstood error messages.
How to Overcome:
- Practice writing small code snippets to familiarize yourself with Java’s syntax.
- Use an Integrated Development Environment (IDE) like IntelliJ IDEA or Eclipse. These tools provide real-time feedback and highlight syntax errors.
- Carefully read error messages; they often point to the exact line of code and issue.
2. Difficulty Grasping Object-Oriented Programming (OOP) Concepts
Java is built around OOP principles like classes, objects, inheritance, and polymorphism, which can be difficult to grasp initially.
How to Overcome:
- Start by building simple classes and objects to see how they work together.
- Use real-world analogies to understand concepts (e.g., a class is like a blueprint, and an object is an instance of that blueprint).
- Work on small projects that focus on one OOP principle at a time, such as creating a class for a “Car” with attributes and behaviors.
3. Struggling with Debugging and Logical Errors
Logic errors, where the program runs but doesn’t produce the expected results, are a common frustration for beginners.
How to Overcome:
- Debug systematically by using print statements (System.out.println) to track the values of variables.
- Learn to use the debugging tools in your IDE to step through your code line by line.
- Write unit tests for your methods to check if they work correctly with different inputs.
4. Managing Data Structures and Algorithms
Understanding how to use arrays, ArrayList, or maps and implementing algorithms like sorting or searching can be challenging.
How to Overcome:
- Start with simple data structures like arrays and gradually move to collections like ArrayList or HashMap.
- Solve beginner-friendly problems on coding platforms like LeetCode, HackerRank, or Codeforces.
- Watch tutorials or read articles on specific algorithms and practice implementing them.
5. Overwhelmed by Advanced Features and Libraries
Java’s vast ecosystem of libraries and frameworks can be overwhelming, leading beginners to feel lost about what to focus on.
How to Overcome:
- Focus on core Java concepts before diving into libraries or frameworks.
- Learn to read documentation for libraries and use only those relevant to your project.
- Gradually explore popular libraries like JavaFX for GUI applications or Apache POI for working with files.
6. Lack of Patience with Project Bugs
Encountering bugs in your projects is inevitable, but beginners might feel discouraged when they can’t fix issues quickly.
How to Overcome:
- Break your problem into smaller pieces and debug one section at a time.
- Seek help from online communities like Stack Overflow or Java forums by sharing your specific issue.
- Cultivate patience and treat debugging as a learning experience.
7. Staying Consistent with Learning
Consistency is a common challenge for many beginners, especially when progress feels slow or obstacles arise.
How to Overcome:
- Set small, achievable goals, like coding for 30 minutes daily.
- Track your progress by maintaining a journal or checklist of completed projects and concepts.
- Join online communities or study groups to stay motivated and accountable.
By recognizing these challenges and proactively addressing them, you’ll build resilience and improve as a Java developer. Remember, every programmer faces obstacles—it’s how you tackle them that defines your growth.
Best Practices for Clean and structured Java Code
Writing clean and efficient code is essential for building robust and maintainable Java projects. As a beginner, developing good coding habits early on will help you avoid common pitfalls and ensure your projects are easy to debug, extend, and share with others. Here are some best practices to follow:
Follow Consistent Naming Conventions
Using meaningful and consistent names for variables, methods, and classes makes your code easier to understand.
- Variables and methods: Use camelCase (e.g., totalScore, calculateSum).
- Classes: Use PascalCase (e.g., StudentRecord, BankAccount).
- Constants: Use UPPER_SNAKE_CASE (e.g., MAX_SCORE, PI_VALUE).
Why It Matters: Readable and descriptive names reduce confusion and make the purpose of your code clear.
- Write Modular Code
Break your code into smaller, reusable methods or classes. Each method should perform a single, well-defined task.
- Example: Instead of writing one long method to calculate grades, split it into smaller methods like calculateAverage() and determineGrade().
Why It Matters: Modular code is easier to read, debug, and maintain.
- Comment Your Code Sparingly but Effectively
Add comments to explain the purpose of complex logic or critical sections of your code. Avoid over-commenting obvious parts, such as:
int sum = a + b; // Adding a and b (Unnecessary comment)
Why It Matters: Useful comments help others (and your future self) understand your thought process without cluttering the code.
- Avoid Hardcoding Values
Use constants or variables instead of hardcoding values directly in your code.
- Example: Instead of if (age > 18), use if (age > LEGAL_DRIVING_AGE).
Why It Matters: This improves flexibility and makes it easier to update values later without changing the entire codebase.
- Use Proper Indentation and Formatting
Consistently format your code for better readability. Most IDEs can automatically format your code (e.g., Ctrl+Shift+F in Eclipse).
- Use 4 spaces for indentation.
- Place curly braces on the same line for methods and classes.
Why It Matters: Proper formatting makes your code easier to navigate, especially in large projects.
- Handle Exceptions Gracefully
Always include proper exception handling using try, catch, and finally blocks.
- Example: When working with file I/O, handle errors like file not found:
try {
BufferedReader reader = new BufferedReader(new FileReader(“data.txt”));
// Read file
} catch (FileNotFoundException e) {
System.out.println(“File not found: ” + e.getMessage());
} finally {
reader.close();
}
Why It Matters: This ensures your program doesn’t crash unexpectedly and provides meaningful feedback to the user.
- Optimize for Performance
Avoid unnecessary computations or memory usage.
- Example: Instead of recalculating the same value repeatedly, store it in a variable.
- Use efficient data structures like HashMap over ArrayList when fast lookups are required.
Why It Matters: Optimized code runs faster and consumes fewer resources.
- Test Your Code Thoroughly
Test each method individually (unit testing) and the entire program for different input scenarios.
- Use tools like JUnit for systematic testing.
- Test edge cases and unexpected inputs to ensure robustness.
Why It Matters: Testing helps catch errors early and ensures your application behaves as expected.
- Refactor Regularly
Revisit your code to simplify or improve it.
- Look for repetitive patterns and extract them into reusable methods.
- Remove unused code or variables.
Why It Matters: Refactoring ensures your codebase remains clean, efficient, and easy to maintain.
- Keep Learning and Adapting
Programming languages evolve, and so should your skills. Stay updated with new Java features (like the enhanced switch statement in Java 14) and adopt modern practices as they emerge.
Why It Matters: Staying current helps you write better, more efficient code and keeps you competitive in the industry.
By adhering to these best practices, you’ll not only create high-quality Java projects but also develop a professional approach to coding that will serve you well throughout your career.
How Java Projects Build Real-World Skills
Java beginner projects are more than just practice exercises—they serve as a foundation for real-world applications. The skills and concepts you learn while building beginner projects can be directly applied to professional environments, giving you the confidence and knowledge to tackle more complex challenges. Here’s how these projects help prepare you:
- Learning Problem-Solving Skills
Every project starts with a problem you need to solve. For example, creating a calculator project requires breaking down mathematical operations into steps. This teaches you how to approach problems systematically, which is a critical skill for real-world development.
- Real-World Application: Identifying client requirements and breaking them into manageable features.
- Familiarity with Programming Concepts
Beginner projects solidify your understanding of Java fundamentals like loops, conditionals, and methods. These core concepts are the building blocks for any Java application.
- Real-World Application: Most enterprise applications rely on these basic constructs, making them essential for writing scalable and maintainable code.
- Introduction to Object-Oriented Programming (OOP)
Projects like a banking system or a student management application introduce you to OOP principles. You learn how to design classes, create objects, and use concepts like inheritance and polymorphism.
- Real-World Application: In professional development, OOP is used extensively to model complex systems (e.g., inventory management or financial systems).
- Working with Data Structures and Algorithms
Beginner projects often require you to store and manipulate data using arrays, lists, or maps. You’ll also use algorithms to perform tasks like sorting or searching.
- Real-World Application: Applications like e-commerce platforms or search engines rely heavily on efficient data management and algorithms.
- Exposure to Input Validation and Error Handling
Building beginner projects teaches you how to handle user input and manage errors gracefully. For instance, validating inputs in a number guessing game helps you understand how to prevent crashes caused by invalid data.
- Real-World Application: Validating user inputs is critical in any application, especially in web forms or database queries, to prevent errors or security vulnerabilities.
- Understanding File Handling
Projects like a to-do list with save and load functionality introduce you to file handling in Java. You learn how to read from and write to files, which is a common requirement in real-world applications.
- Real-World Application: File handling is essential in creating applications like log management systems or data export tools.
- Hands-On Experience with Debugging
While working on beginner projects, you’ll inevitably encounter bugs. Debugging helps you understand how to identify and resolve issues in your code.
- Real-World Application: Debugging is a daily task for developers to ensure that applications run smoothly and meet user expectations.
- Preparing for Collaborative Development
Though beginner projects are often done individually, they teach you habits like writing clean code, using meaningful variable names, and adding comments. These practices are essential when working in a team.
- Real-World Application: Collaboration in a team setting often involves shared codebases where readability and maintainability are crucial.
- Gaining Confidence with Tools and Frameworks
As you progress, beginner projects can introduce you to tools like Git for version control or libraries like Swing for graphical interfaces. This prepares you for using more advanced tools and frameworks in professional development.
- Real-World Application: Most software companies use version control systems and frameworks extensively in their development processes.
- Building a Portfolio to Showcase Your Skills
Completing beginner projects gives you tangible proof of your skills, which you can include in your portfolio or share on platforms like GitHub. A well-documented project demonstrates your ability to learn, build, and solve problems.
- Real-World Application: Employers and clients often look for portfolios to evaluate a developer’s potential.
By working on Java beginner projects, you’re not just learning to code—you’re laying the groundwork for a successful career in software development. These projects simulate the kinds of tasks you’ll face in the real world, ensuring you’re well-prepared for the challenges ahead.
improve Your Java Beginner Projects
Once you’ve built your first few Java beginner projects, the next step is to enhance them. Adding new features or improving the structure of your projects not only deepens your understanding of Java but also makes your work more impressive and useful. Here are some practical tips to take your projects to the next level:
- Add More Features
Enhancing the functionality of your project can make it more engaging and practical. For instance:
- Calculator Project: Add scientific operations like square root, power, or trigonometric functions.
- To-Do List: Allow users to set deadlines and mark tasks as completed.
Why It Helps: Adding features introduces you to more Java classes and libraries, expanding your skill set.
- Improve the User Interface (UI)
Many beginner projects rely on simple console input and output. Upgrading the UI can make your project more user-friendly:
- Use JavaFX or Swing to create graphical interfaces for your projects.
- Experiment with buttons, sliders, and text fields for a polished look.
Why It Helps: Working on UI development prepares you for building professional desktop applications.
- Implement Error Handling and Input Validation
Ensure your project handles incorrect or unexpected input gracefully. For example:
- Validate user input to avoid runtime errors (e.g., ensure a number is entered where required).
- Use try-catch blocks to handle exceptions like division by zero or invalid file paths.
Why It Helps: Robust error handling is a critical skill for building reliable software.
- Optimize Performance
Refactor your code to improve efficiency:
- Replace nested loops with more efficient algorithms where possible.
- Use appropriate data structures, such as HashMap for fast lookups instead of iterating through a list.
Why It Helps: Optimizing code teaches you to write scalable applications suitable for real-world use cases.
- Add a Data Persistence Layer
Introduce functionality to save and retrieve data:
- Use file handling to store data locally.
- Learn basic database operations using JDBC (Java Database Connectivity). For instance, a to-do list app could save tasks in an SQLite database.
Why It Helps: Data persistence is a core component of many real-world applications, such as e-commerce systems or banking software.
- Make Your Code Modular and Reusable
Refactor your code into smaller, reusable components:
- Extract repeated logic into methods or utility classes.
- Use interfaces and abstract classes to make your code extensible.
Why It Helps: Modular code is easier to debug, extend, and maintain, mimicking professional development practices.
- Add Documentation
Create clear and comprehensive documentation for your project:
- Use Javadoc to generate documentation for your classes and methods.
- Include a README file in your project that explains how to set it up and use it.
Why It Helps: Good documentation makes your projects more accessible to others, especially if you’re showcasing them in a portfolio.
- Integrate Testing
Introduce testing to ensure your project behaves as expected:
- Write unit tests using frameworks like JUnit to test individual components.
- Simulate edge cases to test your application’s robustness.
Why It Helps: Testing is a critical part of professional software development and demonstrates attention to detail.
- Version Control Your Project
Use Git to track changes to your code:
- Commit changes regularly with meaningful messages.
- Push your project to a GitHub repository to showcase your work.
Why It Helps: Version control is a standard practice in software development, and hosting projects online builds your professional presence.
- Seek Feedback and Iterate
Share your project with peers, mentors, or online communities like Stack Overflow or GitHub:
- Ask for feedback on your code’s readability and functionality.
- Incorporate suggestions to improve your project iteratively.
Why It Helps: Feedback provides new perspectives and helps you grow as a developer.
Enhancing your beginner projects not only improves your technical skills but also showcases your commitment to learning and growth. By continually refining your projects, you’ll build a portfolio that stands out to employers and clients.
How to Present Your Java Projects to Employers
Building Java beginner projects is only the first step. Showcasing your work effectively can open doors to internships, jobs, or freelance opportunities. Employers value practical skills, and a well-presented portfolio of projects demonstrates your capabilities. Here’s how you can showcase your Java projects to make a lasting impression:
- Organize Your Projects into a Portfolio
Create a dedicated portfolio that highlights your best Java projects:
- Use platforms like GitHub, GitLab, or Bitbucket to host your code.
- Include a README file for each project that describes its purpose, features, and how to run it.
- Add screenshots or videos showcasing the functionality of your projects.
Why It Helps: A structured portfolio gives employers an immediate understanding of your skills and effort.
- Write Detailed Documentation
Comprehensive documentation helps others understand your project:
- Include a summary of the project’s goals and key features.
- Add instructions for setting up and running the project.
- Use Javadoc to explain your classes and methods.
Why It Helps: Detailed documentation demonstrates professionalism and makes your project accessible to a broader audience.
- Create an Online Presence
Use online platforms to share your projects:
- GitHub: Upload your projects and maintain active repositories.
- LinkedIn: Write posts about your learning journey and share links to your projects.
- Personal Website: If you have one, create a portfolio page showcasing your projects.
Why It Helps: An online presence boosts your visibility and shows potential employers your passion for programming.
- Build Interactive Demonstrations
Set up live demos to showcase your projects:
- Use platforms like Replit or Glitch to host small projects online.
- Record a video walkthrough explaining your code and demonstrating its features.
Why It Helps: Interactive demos allow employers to experience your project firsthand, creating a lasting impression.
- Highlight the Skills You Used
For each project, list the technical and problem-solving skills you demonstrated:
- Mention the tools, libraries, or frameworks you used (e.g., JavaFX, JDBC).
- Explain the challenges you faced and how you overcame them.
Why It Helps: Highlighting skills shows your understanding of Java and your ability to apply it practically.
- Tailor Your Projects for Job Roles
Focus on projects that align with the job or internship you’re applying for:
- For a web development role, showcase Java projects using Spring Boot.
- For a data analysis role, include projects that involve file handling or APIs.
Why It Helps: Tailored portfolios show employers you have relevant experience for their requirements.
- Network with Developers and Recruiters
Share your projects with professionals in the industry:
- Join Java-focused groups on LinkedIn, Discord, or Reddit.
- Participate in hackathons and coding challenges to connect with like-minded peers.
- Ask for feedback and engage in constructive discussions.
Why It Helps: Networking can lead to job opportunities and valuable feedback on your work.
- Include Your Projects on Your Resume
Highlight your most impressive Java projects in the projects section of your resume:
- Provide a brief description, the technologies used, and a link to the project.
- Quantify your impact if possible (e.g., “Designed a library management system to handle up to 500 book records”).
Why It Helps: Including projects demonstrates your practical experience and sets you apart from other candidates.
- Prepare for Interviews with Your Projects
Be ready to discuss your projects in job interviews:
- Explain the purpose and functionality of each project.
- Highlight the problems you solved and the skills you gained.
- Be prepared to dive into specific code sections if asked.
Why It Helps: Discussing your projects confidently shows employers your expertise and enthusiasm.
- Keep Your Portfolio Updated
As you build more advanced projects, update your portfolio to showcase your growth:
- Replace older or simpler projects with more complex ones.
- Add new technologies or methodologies you’ve learned.
Why It Helps: An up-to-date portfolio reflects your ongoing learning journey and commitment to improvement.
By effectively showcasing your Java beginner projects, you’ll demonstrate not only your technical skills but also your dedication, creativity, and problem-solving abilities. A strong portfolio can set you apart in the competitive tech job market and help you land your dream role.
Conclusion
Java beginner projects are a great way to establish a solid foundation in programming. They allow you to apply key concepts in real-world scenarios, from object-oriented programming to practical applications. These projects help build technical skills, showcase your learning, and demonstrate your commitment to growth. Whether you’re looking to build a portfolio, land a job, or deepen your understanding, starting with beginner projects is an effective way to advance as a developer. Remember, these projects are just the beginning—keep pushing forward with more complex challenges and collaborative experiences to continue evolving your skills.
FAQs
What is a beginner Java project?
Beginner Java projects are small-scale programs designed to help learners practice and understand Java concepts like loops, conditionals, and object-oriented programming.
What skills should I have before starting Java projects?
Basic understanding of Java syntax, variables, loops, conditionals, arrays, and object-oriented programming concepts like classes and methods.
Why should I work on Java beginner projects?
Working on projects helps solidify your understanding of Java, improves problem-solving skills, and adds practical experience to your portfolio.
How do I choose a suitable Java project as a beginner?
Choose a project that aligns with your current knowledge and gradually increases in complexity. Start with simple projects like calculators or to-do apps.
What tools do I need to start Java projects?
You need a Java Development Kit (JDK), an Integrated Development Environment (IDE) like IntelliJ IDEA or Eclipse, and version control tools like Git.
What are some popular beginner Java project ideas?
- Calculator
- To-do list application
- Number guessing game
- Simple banking system
- Student management system
How long does it take to complete a beginner Java project?
It depends on the complexity of the project and your skill level. Simple projects might take a few hours to a couple of days.
Can I use libraries and frameworks in beginner projects?
Yes, but focus on understanding core Java first. Later, you can explore libraries like Swing for GUIs or frameworks like Spring for advanced projects.
Can Java beginner projects help me get a job?
Yes, showcasing well-documented beginner projects in your portfolio demonstrates your practical skills to potential employers.
Where can I find inspiration for Java projects?
Online platforms like GitHub, coding challenge websites like LeetCode or HackerRank, and community forums like Reddit or Stack Overflow.
What is the best way to document a Java project?
Use comments in your code, write README files explaining the project, and include usage instructions and screenshots.
How can I make my Java projects more interactive?
Incorporate user inputs, create GUIs using libraries like JavaFX or Swing, and add features like real-time updates.
What should I do if I get stuck while building a project?
Refer to Java documentation, search for solutions online, ask for help in programming forums, or revisit Java concepts.
What’s the next step after completing beginner Java projects?
Move on to intermediate projects, learn about advanced Java topics like multithreading and networking, and explore frameworks like Spring or Hibernate.
What are the common challenges faced in Java beginner projects?
Some common challenges include understanding error messages, managing project structure, implementing object-oriented principles effectively, and debugging runtime errors. Regular practice and seeking help from the community can overcome these hurdles.