Python Programming Projects for Beginners
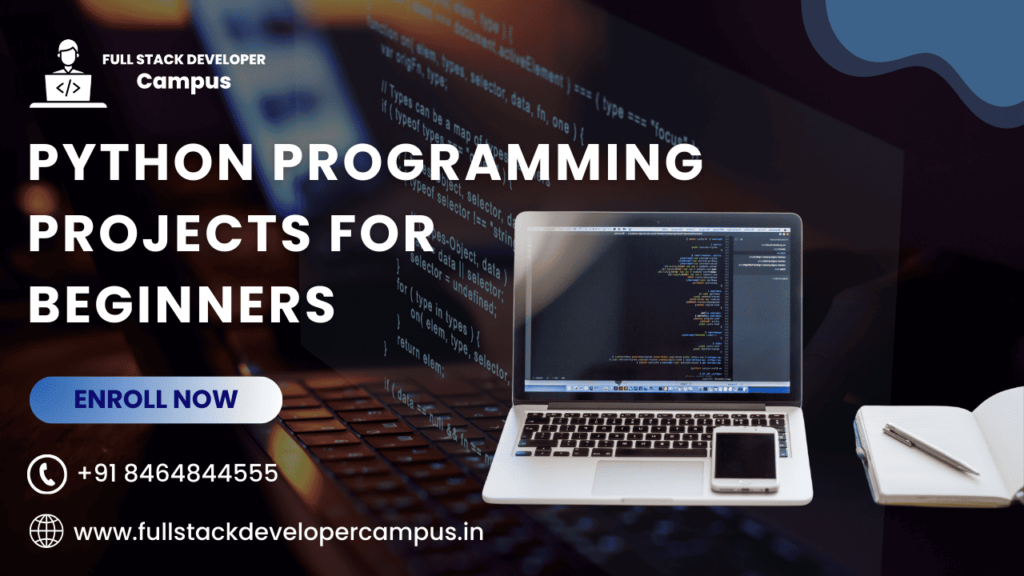
Project 1. Build a Fun Number Guessing Game with Randomized Hints and Feedback
Project Overview
The Number Guessing Game is a simple yet engaging Python project that tests a player’s ability to guess a randomly generated number. The game provides hints such as “Too High” or “Too Low” after each attempt, helping the player refine their guesses. The game continues until the correct number is found, making it a great way to practice Python Programming Projects for Beginners basics.
Key Skills Required
Python Basics – Variables, loops, conditionals
User Input Handling – Using
input()
for interactive gameplayRandom Number Generation – Utilizing the
random
moduleError Handling – Preventing crashes from invalid inputs
Steps to Build the Game
1. Setting Up the Environment
Install Python if not already installed
Use any text editor or IDE like VSCode, PyCharm, or IDLE
2. Generating a Random Number
Use
random.randint(start, end)
to create a random number within a defined range
3. Taking User Input
Prompt the player to enter a guess using
input()
4. Providing Hints
Compare the guessed number with the actual number and display:
“Too High” if the guess is greater
“Too Low” if the guess is smaller
5. Using Loops for Multiple Attempts
Implement a
while
loop to allow repeated guesses until the player finds the correct number
6. Handling Errors
Use
try-except
to manage non-numeric inputs and prevent Python Programming Projects for Beginners crashes
7. Displaying Results
Once the correct number is guessed, display a success message
with the number of attempts
Enhancements
Add difficulty levels with different number ranges
Keep track of the best score based on the fewest attempts
Project 2. Design a Feature-Rich To-Do List Application for Task Management
1. User-Friendly Interface
Intuitive design with a clean and responsive layout
Dark and light mode for better accessibility
2. Task Creation and Categorization
Add, edit, and delete tasks easily
Categorize tasks by priority (High, Medium, Low)
Organize tasks into lists such as Work, Personal, and Shopping
3. Deadline and Reminders
Set due dates for each task
Receive notifications and reminders via email or mobile alerts
4. Recurring Tasks and Automations
Schedule daily, weekly, or monthly recurring tasks
Auto-reschedule missed tasks
5. Collaboration and Sharing
Share tasks with team members
Assign tasks and track progress collaboratively
6. Task Completion and Progress Tracking
Mark tasks as completed and track progress
Visual indicators like progress bars or percentage completion
7. Integration with Other Tools
Sync with Google Calendar, Outlook, and Slack
Export tasks as PDFs or CSV files
8. Data Security and Cloud Sync
Secure cloud backup for easy access across devices
End-to-end encryption for data privacy
Project 3. Develop a User-Friendly Calculator for Basic Arithmetic Operations
1. Intuitive User Interface
Clean and minimalistic design for ease of use
Responsive layout for both desktop and mobile devices
Large, well-spaced buttons for seamless input
2. Basic Arithmetic Operations
Addition (+) – Sum of two or more numbers
Subtraction (-) – Difference between numbers
Multiplication (*) – Product of numbers
Division (/) – Quotient of numbers with error handling for division by zero
3. Error Handling and Validation
Prevents invalid inputs like letters or special characters
Displays clear error messages for incorrect operations
Handles division by zero gracefully
4. Memory and History Functionality
Stores recent calculations for quick reference
Option to clear history or keep track of multiple calculations
5. Accessibility and Customization
Dark and light mode options for user preference
Keyboard shortcuts for quick calculations
Adjustable button sizes for better usability
Project 4. Create a Python-Based Password Generator for Strong Passwords
Random Password Generation
Uses the random and secrets modules to generate unpredictable passwords
Includes uppercase, lowercase letters, numbers, and special characters.
2. Customizable Password Length
Allows users to define password length (e.g., 8, 12, 16, or more characters)
Option to exclude specific characters for user preference
3. Strength Assessment
Checks password strength based on length and character diversity
Displays suggestions for improving weak passwords
4. Secure Storage and Copy to Clipboard
Option to copy generated passwords directly to the clipboard
Securely store passwords in a local encrypted file
5. User-Friendly Interface
Simple command-line or GUI-based version using Tkinter
Easy-to-use interface for quick password generation
Steps to Build the Project
Import Required Modules – Use random, secrets, and string for secure password generation
Define Password Criteria – Set minimum requirements for strong passwords
Generate a Secure Password – Randomly select characters from predefined character sets
Display and Copy Password – Provide an option to copy the password to the clipboard
Enhance with GUI – Use Tkinter for a graphical version
Project 5. Build a Weather App Using Python and Real-Time API Data
Random Password Generation
Uses the random and secrets modules to generate unpredictable passwords
Includes uppercase, lowercase letters, numbers, and special characters.
2. Customizable Password Length
Allows users to define password length (e.g., 8, 12, 16, or more characters)
Option to exclude specific characters for user preference
3. Strength Assessment
Checks password strength based on length and character diversity
Displays suggestions for improving weak passwords
4. Secure Storage and Copy to Clipboard
Option to copy generated passwords directly to the clipboard
Securely store passwords in a local encrypted file
5. User-Friendly Interface
Simple command-line or GUI-based version using Tkinter
Easy-to-use interface for quick password generation
Steps to Build the Project
Import Required Modules – Use random, secrets, and string for secure password generation
Define Password Criteria – Set minimum requirements for strong passwords
Generate a Secure Password – Randomly select characters from predefined character sets
Display and Copy Password – Provide an option to copy the password to the clipboard
Enhance with GUI – Use Tkinter for a graphical version
Project 6. Design a Web Scraper to Extract Data Using BeautifulSoup and Python
Understanding Web Scraping
Web scraping involves fetching web page content, parsing its structure, and extracting relevant data. The process typically includes:
Sending HTTP requests to access web pages
Parsing the HTML structure to identify and extract desired elements
Storing the extracted data in a structured format such as CSV, JSON, or databases
2. Key Features of a Web Scraper
A. Fetching and Parsing Web Content
A web scraper retrieves HTML content from a website using libraries like requests. The BeautifulSoup library then parses this content, allowing easy navigation and data extraction.
B. Targeted Data Extraction
Using BeautifulSoup, specific elements like headings, tables, images, and metadata can be extracted based on their HTML tags (<h1>, <p>, <div>, etc.) and attributes (class, ID).
C. Handling Dynamic Content
Static websites can be easily scraped using BeautifulSoup, but JavaScript-based dynamic content may require Selenium or API-based approaches.
D. Data Cleaning and Storage
After extraction, the data is cleaned to remove unnecessary characters and formatting. It can then be saved in CSV, JSON, or relational databases for further analysis.
E. Error Handling and Optimization
A well-designed scraper should handle errors like HTTP failures, timeouts, and missing data gracefully. Rate limiting and user-agent headers prevent websites from blocking requests.
3. Best Practices for Ethical Scraping
Check the website’s robots.txt file to ensure scraping is allowed.
Respect website policies and avoid excessive requests that may overload servers.
Use official APIs when available for structured data access.
Avoid scraping personal or copyrighted content without permission.
4. Applications of Web Scraping
E-commerce: Price tracking and competitor analysis
News Aggregation: Collecting articles from multiple sources
Job Portals: Extracting job listings for recruitment insights
Data Research: Gathering structured data for analytics
Project 7. Develop an Interactive Multiple-Choice Quiz Application with Scoring
Project Description:
The Interactive Multiple-Choice Quiz Application is a Python program that allows users to take a quiz with multiple-choice questions. Each question has a set of possible answers, and the user selects the correct answer. The app keeps track of the user’s score, provides immediate feedback for correct or incorrect answers, and displays the total score at the end of the quiz. This project demonstrates how to use Python to create a simple yet engaging interactive application for users.
Skills Required:
Python basics (variables, loops, conditionals).
Functions for modular programming.
Data structures (lists and dictionaries) for storing questions and answers.
String manipulation and formatting.
Steps to Execute the Project:
Set Up the Environment:
Install Python and a code editor (e.g., PyCharm, VSCode).
Create a List of Questions and Answers:
Use a list of dictionaries, where each dictionary contains a question, a list of possible answers, and the correct answer.
Example:
questions = [
{
‘question’: ‘What is the capital of France?’
‘options’: [‘Paris’, ‘Berlin’, ‘Rome’, ‘Madrid’],
‘answer’: ‘Paris’
},
{
‘question’: ‘Who wrote “Hamlet”?’
‘options’: [‘Shakespeare’, ‘Dickens’, ‘Hemingway’, ‘Austen’],
‘answer’: ‘Shakespeare’
}
]
Display questions and collect user answers:
Loop through the list of questions and display each question and its options.
Use the input() function to allow the user to select an answer.
Ensure that the input is valid (e.g., check if the user chooses a valid option).
Check the user’s answer:
After each question, compare the user’s input with the correct answer stored in the dictionary.
Provide immediate feedback:
If correct, increment the score and display “Correct!”
If incorrect, display the correct answer and “Incorrect.”
Keep Track of the Score:
Use a variable (e.g., score) to track the user’s score throughout the quiz.
Display the score after each question or at the end of the quiz.
End the quiz and display the final score:
Once all the questions have been answered, display the user’s total score and the percentage of correct answers.
Example:
print(f’Your total score: {score}/{len(questions)}’)
Enhance the Application (Optional):
Add a time limit for each question or the entire quiz.
Provide different difficulty levels (easy, medium, hard) with varying questions.
Allow the user to replay the quiz or choose a different category.
Save the high score and display it after each quiz.
Expected Outcome:
Functionality: A simple and interactive quiz app that allows users to answer multiple-choice questions, keeps track of their score, and provides feedback on correct and incorrect answers.
Learning Outcomes:
Learn how to use lists and dictionaries to store and retrieve data.
Understand how to create an interactive user interface with Python’s built-in functions.
Gain experience with loops, conditionals, and string manipulation.
User Experience: A fun and educational quiz experience where users can answer questions and see their scores in real time.
Project 8. Create an Expense Tracker Application for Budget Management
Project Description:
The Expense Tracker Application is a Python-based tool that helps users track their income and expenses, allowing them to manage their budget effectively. The user can input various categories of expenses (e.g., food, transportation, entertainment) and their respective amounts. The app calculates and displays the total expenses, the remaining budget, and provides an overview of the spending in each category. This project will teach users how to manage and store financial data using Python Programming Projects for Beginners and create an interactive user interface for personal finance management.
Skills Required:
Python basics (variables, loops, conditionals).
Functions for modular programming.
File handling (storing data in text or CSV files).
Data structures (lists, dictionaries) for tracking expenses and categories.
Steps to Execute the Project:
Set Up the Environment:
Install Python and a code editor (e.g., PyCharm, VSCode).
Create the expense categories and budget:
Define the available categories (e.g., food, rent, transportation).
Prompt the user to enter their monthly budget amount.
Store this budget in a variable, such as monthly budget.
Define Functions for Expense Tracking:
Create a function to add an expense, specifying the category and amount.
Create a function to display the total expenses, the remaining budget, and the expenses by category.
Track and Store Expenses:
Allow the user to enter their expenses through an input form. For each expense, prompt for the category and amount.
Store these expenses in a dictionary, with categories as keys and amounts as values.
Use a loop to continuously track expenses until the user decides to exit the program.
Calculate and display the remaining budget:
After each expense entry, calculate the total expenses and subtract this from the initial budget to determine the remaining budget.
Display the total spent, the remaining budget, and a breakdown of expenses by category.
Data Persistence:
To keep the user’s data after closing the app, store the expense records in a CSV or text file (e.g., expenses.txt).
Implement functionality to load and save expenses between app sessions.
Handle Errors:
Add error handling for invalid input (e.g., non-numeric values, negative amounts).
Ensure that users can’t enter more expenses than their budget allows.
Enhance the Application (Optional):
Add a feature for generating monthly or yearly reports.
Provide visualizations (e.g., pie charts) to represent spending by category using libraries like matplotlib.
Allow the user to set and manage multiple budgets for different months or categories.
Expected Outcome:
Functionality: A working expense tracker that helps users manage their budget by tracking expenses and showing the remaining budget after each entry.
Learning Outcomes:
Understand how to store and manage financial data using Python’s data structures.
Learn how to work with file handling to persist data across sessions.
Gain experience with implementing error handling and user input validation.
User Experience: A simple and effective tool for managing personal finances and tracking spending habits. Users will be able to view their spending in various categories and make adjustments to stay within their budget.
Project 9. Build an Image-to-ASCII Art Converter for Artistic Fun
Project Description:
The Image-to-ASCII Art Converter Python Programming Projects for Beginners transforms images into ASCII art, a form of creating pictures using text characters. This app takes an input image and converts it into a representation of characters such as ‘@’, ‘#’, ‘*’, or ‘.’ in a gridlike structure. The application analyzes the pixel intensity of the image and uses different characters to represent varying levels of brightness. This project will help you understand image processing, working with pixel data, and creating fun artistic outputs in Python.
Skills Required:
Python basics (variables, loops, conditionals).
Understanding image manipulation using Python libraries.
Working with image files and pixel data.
Using external libraries like Pillow for image processing.
Steps to Execute the Project:
Set Up the Environment:
Install Python and a code editor (e.g., PyCharm, VSCode).
Install the Pillow library, which will be used for image processing:
pip install Pillow
Load and display the Image:
Use the Pillow library to open and display the image.
Example:
from PIL import Image
image = Image.open(‘your_image.jpg’)
image.show()
Resize the Image (Optional):
Resize the image to a smaller size (optional) for easier processing and a better ASCII representation.
Example:
image = image.resize((width, height))
Convert the image to grayscale:
Convert the image to grayscale so that pixel intensity can be easily assessed.
This step will simplify the conversion process by reducing the image to shades of gray.
Example:
grayscale_image = image.convert(‘L’) # ‘L’ mode is for grayscale
Map Pixel Intensity to ASCII Characters:
Define a set of characters based on brightness. Darker pixels should correspond to denser characters (like ‘@’ or ‘#’), and lighter pixels should correspond to lighter characters (like ‘.’ or”).
Example:
ASCII_CHARS = [‘@’, ‘#’, ‘S’, ‘%’, ‘?’, ‘*’, ‘+’, ‘;’, ‘:’, ‘,’, ‘.’]
Iterate Through Pixels:
Get pixel values from the grayscale image and map them to the corresponding ASCII characters based on their intensity.
Create a string representation of the image with characters representing the pixel values.
Generate ASCII Art:
Loop through the image pixels, map each pixel’s intensity to an ASCII character, and build the ASCII art by combining those characters.
Example:
python
CopyEdit
ascii_image = “”
for pixel_value in grayscale_image.getdata():
ascii_image += ASCII_CHARS[pixel_value // 25] # Mapping to characters
Display or Save the ASCII Art:
Output the ASCII art to the console or save it to a text file.
Example:
with open(‘output.txt’, ‘w’) as f:
f.write(ascii_image)
Enhance the Application (Optional):
Allow users to choose different ASCII character sets or image dimensions.
Add features for adjusting the contrast or brightness of the image before converting.
Convert the ASCII art back into an image (reverse process).
Provide a graphical user interface (GUI) for easier interaction, where users can upload their images and download the generated ASCII art.
Expected Outcome:
Functionality: A working Image-to-ASCII Art Converter that takes an image, processes it, and converts it into an ASCII art representation.
Learning Outcomes:
Experience in image manipulation and processing using the Pillow library.
Understanding how to map pixel intensity to different characters for creating art.
Gain knowledge of handling images and creating text-based art in Python Programming Projects for Beginners.
User Experience: Users will be able to upload an image, convert it to ASCII art, and save or view the resulting text art. This offers a fun and creative way to generate art using only text characters.
Project 10. Code an Engaging Rock, Paper, Scissors Game with Python Logic
1. Game Rules and Mechanics
The game follows three basic rules:
Rock beats Scissors (Rock crushes Scissors).
Scissors beats Paper (Scissors cut Paper).
Paper beats Rock (Paper wraps Rock).
If both choose the same, it’s a tie.
2. Key Features of the Rock, Paper, Scissors Game
A. User and Computer Interaction
The user chooses Rock, Paper, or Scissors.
The computer randomly chooses its move using Python Programming Projects for Beginners random module.
B. Game Logic Implementation
The program matches the user’s choice with the computer’s.
Based on the predefined rules, it determines the winner.
The result is displayed, and the user gets an option to play again.
C. Looping for Multiple Rounds
A loop allows the user to play multiple rounds until they choose to exit.
Each round updates the win/loss count for score tracking.
D. Input Validation
Ensures that users enter a valid choice (rock, paper, or scissors).
Prevents crashes by handling incorrect inputs gracefully.
3. Enhancements for a Better User Experience
To make the game more engaging, consider adding:
A scoring system to track wins and losses.
Difficulty levels, where the computer’s moves are based on probabilities instead of being completely random.
A graphical interface (GUI) using Tkinter or Pygame for better visuals.
4. Benefits of Building This Project
Strengthens knowledge of Python conditionals, loops, and functions.
Introduces random module for computer-generated choices.
Helps develop user input handling and validation techniques.
Conclusion
Developing a Rock, Paper, Scissors game in Python is a simple yet powerful way to practice essential programming skills like user input handling, conditional logic, and using libraries like random. This project provides hands-on experience and creates a fun, interactive game that reinforces your coding foundation.
The game can be further enhanced with exciting features like scoring systems, multiplayer modes, or graphical interfaces using Tkinter or Pygame. Adding sound effects and animations can make it even more engaging.
By completing this project, you’ll not only boost your Python skills but also gain confidence to work on more advanced and creative applications in the future.
FAQs
What is Python, and why should I learn it?
Python is a high-level, interpreted programming language known for its simplicity and readability. It’s beginner-friendly and widely used in web development, data analysis, machine learning, automation, and more. Learning Python gives you access to a large ecosystem of libraries and tools, making it a versatile language for various applications.
Is Python suitable for beginners?
Yes, Python is one of the most beginner-friendly languages due to its easy-to-understand syntax and vast community support. Its focus on readability makes it an excellent choice for those just starting their programming journey.
How long does it take to learn Python?
The time to learn Python depends on your prior experience and the depth of knowledge you wish to acquire. On average, a beginner can learn the basics in 1-2 months, while mastering advanced concepts may take 6 months to a year with consistent practice.
What are the basic concepts I should learn in Python?
Beginner Python concepts include:
- Variables and data types
- Operators (arithmetic, comparison, logical)
- Control flow (if, elif, else)
- Loops (for, while)
- Functions
- Lists, tuples, and dictionaries
- Error handling with exceptions
Can Python be used for web development?
Yes, Python can be used for web development with frameworks like Django, Flask, and FastAPI. These frameworks allow you to build powerful, scalable web applications efficiently.
What are Python libraries, and why are they important?
Python libraries are pre-written code packages that provide specific functionality. Examples include Numpy for numerical computing, Pandas for data analysis, and Matplotlib for data visualization. Libraries save time by providing solutions to common problems and tasks.
What is the difference between Python 2 and Python 3?
Python 3 is the latest version and includes several improvements and optimizations over Python 2. Python 2 is no longer supported, so it is recommended to use Python 3 for all new projects.
What is Object-Oriented Programming (OOP) in Python?
Object-Oriented Programming (OOP) is a programming paradigm that organizes code into classes and objects. Python supports OOP, allowing you to define classes, create objects, and use inheritance, polymorphism, and encapsulation to structure your programs.
How do I handle errors in Python?
Errors can be handled using try, except, else, and finally blocks. This allows you to catch exceptions and prevent your program from crashing. The raise keyword can also be used to manually trigger exceptions.
What are Python functions, and how do I create them?
A function is a reusable block of code that performs a specific task. You can create functions in Python using the def keyword, followed by the function name and parameters. Functions help break down complex tasks into smaller, manageable pieces of code.
How do I install external Python libraries?
You can install external Python libraries using the pip package manager. For example, to install the requests library, run pip install requests in your command prompt or terminal.
What is the purpose of lists in Python?
A list is a collection of items stored in an ordered sequence. Lists are flexible, allowing you to store different types of data and easily modify, access, or iterate over elements. They are one of Python’s most commonly used data structures.
What is the difference between a list and a tuple in Python?
The main difference between lists and tuples is that lists are mutable (can be changed), while tuples are immutable (cannot be changed). Use tuples when you need a fixed, unchangeable collection of items.
What are some real-world applications of Python?
Python is used in a wide range of industries.
- Web development (e.g., Django, Flask)
- Data science and analysis (e.g., pandas, numpy, matplotlib)
- Machine learning and AI (e.g., TensorFlow, scikit-learn)
- Automation and scripting (e.g., automating repetitive tasks)
- Game development (e.g., Pygame)
How can I practice and improve my Python skills?
To improve your Python skills, try the following:
- Solve coding challenges on platforms like LeetCode, HackerRank, or Codewars.
- Work on personal projects that interest you.
- Contribute to open-source projects on GitHub.
- Read Python books, blogs, and tutorials to deepen your understanding.
- Join Python communities to get support and feedback.