Advanced Java Programming Syllabus
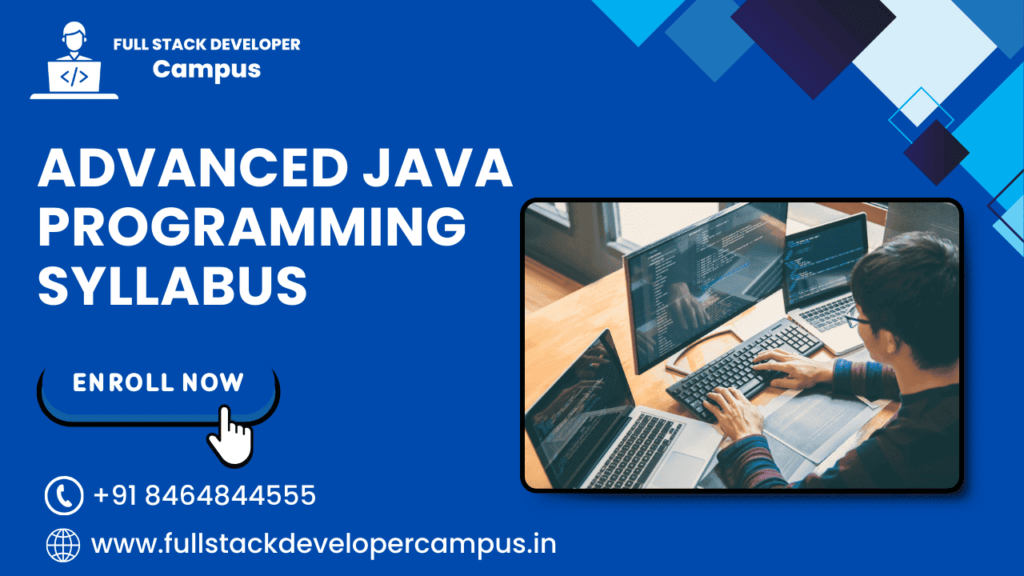
Advanced Java Programming Syllabus
Introduction to Advanced Java: Difference between Core and Advanced Java; importance in web and enterprise applications.
Java Servlets: Handling HTTP requests/responses, servlet lifecycle, session management.
JSP (Java Server Pages): Creating dynamic web pages; scripting elements, directives, and custom tags.
JDBC (Java Database Connectivity): Database connections, executing SQL queries, ResultSet, and transactions.
Multithreading: Thread lifecycle, synchronization, inter-thread communication.
Networking: Building client-server applications using Socket, ServerSocket, TCP/IP, and UDP.
Collections Framework: List, Set, Map, and efficient data manipulation.
Java Frameworks Overview: Basics of Spring and Hibernate for large-scale applications.
Building Web-Based Applications: Integrating Servlets, JSP, and JDBC for dynamic web applications.
Deployment and Tools: Using tools like Apache Tomcat, WAR file packaging, and deployment.
Advanced Java Programming Syllabus
Advanced Java programming is a vital skill for developers aiming to excel in enterprise-level applications, large-scale systems, and modern web services.
While core Java provides a solid foundation for object-oriented programming and basic application development, advanced Java introduces powerful tools and frameworks that enable developers to create robust, scalable, and secure applications tailored to the demands of today’s technology landscape.
One of the key reasons advanced Java is indispensable is its widespread use in enterprise environments. Technologies such as Servlets, JSP, and Enterprise JavaBeans (EJB) allow developers to build dynamic web applications and distributed systems. Frameworks like Spring and Hibernate streamline application development by simplifying database management, dependency injection, and integration with other services.
Moreover, advanced Java programming equips developers with the skills to handle complex tasks such as asynchronous communication using Java Messaging Service (JMS), designing RESTful web services, and implementing robust security measures to protect sensitive data.
These competencies are highly sought after in industries ranging from finance to e-commerce.
By mastering advanced Java, developers not only enhance their problem-solving capabilities but also unlock lucrative career opportunities, from backend development to software architecture, making it a cornerstone of professional growth in the tech world.
Key Features of Advanced Java You Need to Know
Advanced Java builds upon the core concepts of Java, introducing powerful features and tools that enable developers to create enterprise-grade applications, dynamic web solutions, and secure, scalable systems. Here are the key features of advanced Java that every developer should know:
- Java Networking: Advanced Java supports seamless communication between devices over networks using features like sockets, ServerSockets, and URL handling. This is crucial for developing chat applications, real-time collaboration tools, and client-server architectures.
- Database Interaction with JDBC: Java Database Connectivity (JDBC) allows applications to interact with databases efficiently. Features like PreparedStatements, batch processing, and transaction management make it easy to handle complex queries and ensure data consistency.
- Servlets and JSP: Servlets provide server-side functionality for handling HTTP requests, while Java Server Pages (JSP) enable dynamic web content generation. They are essential to the structure of many modern web applications.
- Enterprise JavaBeans (EJB): EJB simplifies the development of scalable, secure enterprise applications by offering features like session beans, message-driven beans, and dependency injection.
- Spring and Hibernate Frameworks: Spring simplifies application development with its dependency injection and MVC architecture. Hibernate streamlines database interactions using Object-Relational Mapping (ORM).
- Web Services Support: Advanced Java facilitates the development of SOAP and RESTful web services, allowing integration with third-party systems and APIs.
7.Enhanced Security Features: Tools like JAAS, SSL/TLS, and cryptographic libraries ensure secure communication and user authentication. dolor sit amet, consectetur adipiscing elit. Ut elit tellus, luctus nec ullamcorper mattis, pulvinar dapibus leo.
Core Concepts of Advanced Java
- 1. Java Networking
Java Networking is a crucial topic that covers the tools and techniques for communication between devices over a network. Key areas include: - Introduction to Networking in Java: Understanding basic networking concepts and how Java facilitates communication.
- Understanding Sockets and ServerSockets: Learn how to establish client-server communication using sockets.
- Working with URLs and HTTP Connections: Handling web-based data through URL objects and HTTP protocols.
- Developing Chat Applications Using TCP/IP: Building real-time chat applications with the Transmission Control Protocol.
Datagram Packets and Multicast Sockets: Explore UDP-based communication and group messaging with multicast sockets.
2. Java Database Connectivity (JDBC)
JDBC enables Java applications to interact with databases seamlessly. Topics include:
Overview of JDBC and Its Architecture: Understanding the structure and components of JDBC.
Connecting Java Applications to Databases: Establishing database connections with drivers.
Executing SQL Queries from Java: Running SQL commands through Java.
ResultSet and PreparedStatement Usage: Efficiently retrieving and managing data.
Transaction Management and Batch Processing: Ensuring data integrity and optimizing database operations.
3. Java Servlets
Java Servlets form the backbone of server-side applications. Topics include: - Introduction to Servlets: The basics of creating and deploying servlets.
- Servlet Life Cycle: Learn how servlets are initialized, executed, and destroyed.
- Handling HTTP Requests and Responses: Processing client requests and generating responses.
- Session Management Techniques: Tracking user sessions using cookies and HttpSession.
- Servlet Filters and Listeners: Enhancing functionality and monitoring application events.
4. Java Server Pages (JSP)
JSP simplifies dynamic web content creation. Key areas are:
Fundamentals of JSP: Introduction to JSP and its advantages over servlets.
JSP Elements: Directives, Actions, and Scriptlets: Building blocks of JSP pages.
Expression Language (EL) and Custom Tags: Simplify dynamic content with EL and reusable tags.
JSP and Servlets Integration: Combining the power of JSP and servlets.
Error Handling and Exception Management in JSP: Creating robust and user-friendly applications.
5. Enterprise JavaBeans (EJB)
EJB facilitates enterprise-level application development. Topics include:
Introduction to EJB: Understanding the purpose and types of enterprise beans.
Types of Enterprise Beans: Session, Entity, and Message-Driven: Explore their unique characteristics and use cases.
EJB Life Cycle and Annotations: Managing EJB components with ease.
Dependency Injection in EJB: Simplifying configuration and resource access.
Developing Distributed Applications with EJB: Building scalable and secure applications.
6. Java Messaging Service (JMS)
JMS is essential for asynchronous communication. Key areas include:
Overview of JMS: Introduction to messaging concepts.
Point-to-Point and Publish-Subscribe Models: Understanding different messaging patterns.
Message Producers, Consumers, and Listeners: Implementing messaging functionality.
Configuring JMS Resources: Setting up queues and topics.
Integration with Enterprise Applications: Using JMS in distributed systems.
Advanced Topics in Java
1. Spring Framework
Spring Framework simplifies application development. Topics include:
Overview of Spring Core Concepts: Understanding IoC and Dependency Injection.
Spring MVC for Web Applications: Building robust web applications.
Spring Boot for Microservices Development: Rapidly develop and deploy microservices.
Integration with Hibernate and JPA: Managing database operations efficiently.
2. Hibernate Framework
Hibernate facilitates Object-Relational Mapping (ORM). Key areas include:
Overview of ORM and Hibernate: Understanding the fundamentals of ORM and the benefits of using Hibernate.
Hibernate Configuration and Mapping: Setting up Hibernate with configurations.
HQL (Hibernate Query Language): Simplifying database queries.
Caching in Hibernate: Enhancing application performance.
Implementing Relationships: One-to-One, One-to-Many, and Many-to-Many: Mapping complex relationships.
3. Web Services in Java
Web services enable interoperability. Topics include:
Understanding Web Services: Basics of SOAP and REST.
RESTful Web Services Using JAX-RS: Building REST APIs in Java.
SOAP Web Services Using JAX-WS: Creating SOAP-based services.
JSON and XML Parsing: Handling structured data efficiently.
Securing Web Services: Implementing authentication and encryption.
4. Java Security
Security is critical in application development. Key areas include:
Java Authentication and Authorization Services (JAAS): Managing user authentication.
Securing Java Applications with SSL/TLS: Protecting data in transit.
Cryptography in Java: Encrypting and decrypting sensitive information.
Implementing Secure Sockets: Enhancing communication security.
Role-Based Access Control: Restricting access to authorized users.
Development Tools and Best Practices
1. Build Tools and IDEs
Efficient tools improve productivity. Topics include:
Using Maven and Gradle for Dependency Management: Simplifying project builds.
Advanced Features of IntelliJ IDEA and Eclipse: Leveraging powerful IDE features.
Debugging and Profiling Java Applications: Identifying and fixing issues.
2. Testing Frameworks
Testing ensures software reliability. Topics include:
Unit Testing with JUnit and TestNG: Writing and executing unit tests.
Mocking with Mockito: Simulating dependencies for isolated testing.
Integration Testing for Web Applications: Ensuring seamless component interaction.
3. Code Quality and Performance Optimization
High-quality code is vital. Key areas include:
Code Refactoring Techniques: Improving code readability and maintainability.
Best Practices for Writing Clean Code: Adopting coding standards.
Analyzing and Improving Application Performance: Detecting and resolving bottlenecks.
Hands-on Projects and Case Studies
1. E-commerce Application Development
Create a robust e-commerce platform by:
Building a Scalable Backend with Spring Boot: Handling high traffic effectively.
Implementing Payment Gateways: Enabling secure transactions.
User Authentication and Authorization: Protecting user accounts.
2. Social Media Integration
Develop APIs and connect with social platforms:
Developing APIs for Social Media Platforms: Facilitating data sharing.
Managing OAuth Authentication: Ensuring secure user authentication.
3. Chat Application with Java Networking
Build a real-time communication tool:
Real-time Messaging Using Sockets: Enabling instant communication.
Deploying a Scalable Server: Supporting multiple users simultaneously.
Java Networking structure Connections and Applications
Java Database Connectivity (JDBC)
Java Networking is about allowing different devices and systems to communicate with each other over a network using the Java programming language. The Java platform provides a comprehensive set of APIs and libraries for building networked applications that can exchange data between systems. These applications can be client-server-based, peer-to-peer, or part of distributed systems. Java networking capabilities are primarily implemented through the java.net package, which provides a wide range of features for communication over TCP/IP and other protocols.
Key Concepts in Java Networking
- IP Addressing and Ports: The foundation of Java networking is built on the concept of IP addresses and ports. An IP address uniquely identifies a device on a network, while ports are used to identify specific applications or services running on that device. For example, web servers typically use port 80, while secure servers use port 443.
- Sockets: The most basic building block for network communication in Java is the socket.A socket is a point used to send or receive data over a network. Java has the Socket class for clients and the ServerSocket class for servers to set up communication. Sockets enable bidirectional communication over TCP (Transmission Control Protocol) or UDP (User Datagram Protocol).
TCP: TCP sockets are used for reliable communication, ensuring that data is transmitted in the correct order and without errors.
UDP: UDP sockets are used for faster, but less reliable, communication, where speed is more important than accuracy, such as in video streaming. - Client-Server Model: Java networking commonly follows the client-server model, where a server listens for incoming requests from clients, processes them, and sends responses back. The server runs continuously, awaiting client connections, while clients initiate connections to the server when they need to request services.
ServerSocket: In Java, the ServerSocket class waits for client connections on a certain port.When a client connects, the server makes a Socket object to talk to that client.
Socket: The Socket class in Java provides methods to send and receive data over a network. Both the server and client use the socket to exchange messages. - Datagrams (UDP Communication): UDP is used for applications that need fast communication and can handle some loss of data packets.. Java supports UDP communication through the DatagramSocket and DatagramPacket classes. UDP does not guarantee the order or reliability of data packets, but it is faster compared to TCP.
- Multithreading in Networking: Java networking applications often require multithreading to handle multiple clients simultaneously.This can be done by using the Thread class or by implementing the Runnable interface. In a server application, each incoming client connection can be assigned to a new thread, allowing the server to handle multiple requests concurrently without waiting for one to finish before processing the next.
- URL Handling and HTTP Requests: The java.net package also provides classes to handle URLs and make HTTP requests. The URL class is used to represent a URL, while the URLConnection class helps in reading data from a remote server. These classes are used extensively in web-based Java applications to fetch resources, perform HTTP methods like GET and POST, and manage cookies.
- Security: In Java, security is a significant concern when working with networking.Java has classes like SSLServerSocket and SSLSocket that enable secure communication using SSL and TLS protocols. This ensures that data sent over the network is secure from eavesdropping and tampering.
Practical Use Cases of Java Networking
Web Applications: Java networking is crucial for building web applications that rely on communication between clients (browsers) and servers. Technologies like Java EE (Enterprise Edition) and frameworks like Spring depend on robust networking for handling HTTP requests and responses.
File Transfer Protocols: Java can be used to create applications that transfer files over the internet, such as FTP (File Transfer Protocol) clients and servers.
Chat Applications: Java networking is commonly used in real-time applications like chat programs, where multiple users can communicate simultaneously.
Distributed Systems: In distributed systems, Java networking allows different components of the system to communicate over a network, ensuring that data is consistently shared across machines.
Java Database Connectivity (JDBC) is a key API in Java that enables Java applications to connect and work with relational databases.. It offers a common way to link to databases, run SQL queries, and handle the results. JDBC is fundamental for developers who need to perform database operations such as reading, writing, updating, and deleting data from a database directly from a Java application. JDBC simplifies the integration of Java programs with databases and plays a crucial role in building dynamic applications like web apps, enterprise applications, and more.
Key Concepts of JDBC
- JDBC Architecture: JDBC follows a client-server architecture where the client is the Java application, and the server is the database. The interaction between the two is mediated by the JDBC API. JDBC defines two types of components:
JDBC Drivers are specific to each platform and help Java applications connect to the database.They convert Java calls into database-specific calls.
JDBC API: This is a collection of interfaces and classes that allow Java applications to interact with databases in a standardized way. - JDBC Drivers: There are four types of JDBC drivers, which are categorized based on how they connect Java applications to databases:
Type 1: The JDBC-ODBC Bridge Driver connects Java applications to databases using ODBC.
Type 2: Native-API Driver, which uses database-specific APIs.
Type 3: Network Protocol Driver, which uses a middleware server to connect.
Type 4: Thin Driver, which directly communicates with the database using the database’s native protocol, providing better performance and portability. - Connecting to the Database: The initial step in using JDBC is to connect to the database. This is done by loading the database driver and creating a Connection object using the DriverManager.getConnection() method. The connection string includes the database URL, username, and password.
java
String url = “jdbc:mysql://localhost:3306/mydb”;
String username = “root”;
String password = “password”;
Connection conn = DriverManager.getConnection(url, username, password); - Running SQL Queries: After the connection is set up, the next step is to create a Statement or PreparedStatement object to run SQL queries.JDBC supports both static and dynamic queries:
Statement: Used for executing simple SQL queries like SELECT, INSERT, UPDATE, and DELETE.
PreparedStatement: Used for executing precompiled SQL queries, which can improve performance, especially when the same query is executed repeatedly with different parameters.
Example:
java
Statement stmt = conn.createStatement();
ResultSet rs = stmt.executeQuery(“SELECT * FROM users”); - The ResultSet object contains the data that comes back from a query.It provides methods like next(), getString(), getInt(), and others to retrieve the data from the result set. You can iterate over the result set using a loop.
java
while (rs.next()) {
int id = rs.getInt(“id”);
String name = rs.getString(“name”);
System.out.println(id + “: ” + name);
} - Handling Exceptions: Database operations may throw SQLException, which must be handled properly using try-catch blocks. It’s essential to handle database errors gracefully and close resources properly to prevent memory leaks.
- Transaction Management: JDBC supports transaction management, which allows developers to manage a group of operations as a single unit of work. This ensures that either all operations succeed or none of them are applied (atomicity). Transactions are controlled using commit(), rollback(), and setAutoCommit() methods.
java
conn.setAutoCommit(false); // Disable auto-commit
try {
//Run several SQL commands.
conn.commit(); // Commit transaction
} catch (SQLException e) {
conn.rollback(); // Rollback transaction on failure
} - Closing Resources: It is crucial to close database resources like Connection, Statement, and ResultSet to free up database connections and avoid memory leaks. This can be done in the finally block or by using Java’s try-with-resources statement.
java
try (Connection conn = DriverManager.getConnection(url, username, password);
Statement stmt = conn.createStatement();
ResultSet rs = stmt.executeQuery(“SELECT * FROM users”)) {
while (rs.next()) {
System.out.print(rs.getString(“name”));
}
} catch (SQLException e) {
e.printStackTrace();
}
Advanced JDBC Features
1. Batch Processing: JDBC allows executing multiple SQL statements in a single batch, which can improve performance when working with large volumes of data. The addBatch() and executeBatch() methods are used to add queries to a batch and execute them together.
2. CallableStatement for Stored Procedures: JDBC provides the CallableStatement interface for executing stored procedures in the database. This is useful for executing complex queries or operations that are encapsulated within the database.
java
CallableStatement stmt = conn.prepareCall(“{call get_user_by_id(?)}”);
stmt.setInt(1, userId);
ResultSet rs = stmt.executeQuery();
3. Connection Pooling: For high-performance applications, managing database connections is critical.Connection pooling lets you use a small number of connections again and again instead of creating a new one for each request.. This lowers extra costs and boosts the application’s efficiency. Frameworks such as Apache Commons DBCP and HikariCP are commonly used for connection pooling in Java.
Understanding Java Servlets for Web Development
A Java Servlet is a server-side part that enhances what a web server can do. It is used to handle requests from clients (typically web browsers) and generate dynamic content in response. Servlets are an integral part of Java web technologies, particularly in Java EE (Enterprise Edition), and they interact with the client through HTTP protocols, generating dynamic responses such as HTML, XML, or JSON.
Core Concepts of Java Servlets
Servlet Container:
A servlet container, also known as a servlet engine, is part of a web server that manages servlets.. The container handles lifecycle management, including initializing servlets, processing requests, and sending responses. Popular servlet containers include Apache Tomcat, Jetty, and GlassFish.
- Request and Response:
The request refers to the HTTP request sent by a client (e.g., a browser). This request can contain parameters, headers, and other data. The response is what the server sends back after processing the request, typically in the form of HTML, JSON, or other data.
A servlet processes the request by reading data from it, performing some logic (like database operations or business logic), and then generating a response to be sent back to the client.
Servlet API: - The Servlet API defines a set of interfaces and classes that enable the creation and management of servlets. The main interface in the API is javax.servlet.Servlet, and every servlet must implement it.
- Important classes include HttpServlet (for handling HTTP requests), ServletRequest (to retrieve client data), and ServletResponse (to send data back to the client).
Servlet Lifecycle
The servlet container controls the lifecycle of a servlet, which includes these phases:
Initialization:
When the servlet is requested for the first time, the servlet container sets it up by calling the init() method.This method is called only once during the lifetime of the servlet, typically when the server starts or when the servlet is first accessed.
Request Handling:
After starting up, the servlet is able to manage several client requests. Each request is processed in the service() method, where the servlet determines the type of request (GET, POST, etc.) and responds accordingly. For HTTP requests, the doGet() and doPost() methods of HttpServlet are commonly used to handle GET and POST requests respectively.
Destruction:
When the servlet is not needed anymore or the web application is shutting down, the servlet container calls the destroy() method. method.This lets the servlet free up any resources, such as database connections or file handles, before it is taken out of memory.
Types of Servlets - GenericServlet: A general-purpose servlet that can handle any protocol, not just HTTP. It provides basic functionality but requires overriding methods like service() to handle specific protocols.
- HttpServlet: A specialized subclass of GenericServlet, specifically designed to handle HTTP requests. It simplifies the process of writing web applications by providing pre-built methods for handling GET, POST, PUT, DELETE, and other HTTP methods.
Benefits of Java Servlets
Portability: Servlets are created using Java, a language that works on any platform.. This allows servlets to run on any platform that supports a Java Runtime Environment (JRE).
Performance: Unlike traditional CGI (Common Gateway Interface), servlets do not need to be reloaded or recompiled for each request. They run within the servlet container, which optimizes their performance through techniques like multithreading and connection pooling.
Scalability: Servlets support multiple concurrent requests through threading, making them highly scalable. This is particularly useful for handling large volumes of web traffic.
Security: Java provides built-in security features like SSL (Secure Socket Layer) support, user authentication, and authorization, which help protect the web applications that use servlets.
Extensibility: Servlets are easily extendable and can be combined with other Java EE technologies, like JavaServer Pages (JSP), Enterprise JavaBeans (EJB), and Java Persistence API (JPA), to create robust and feature-rich web applications.
Servlet Mappings
Servlets are often mapped to specific URLs, which means that whenever a client accesses a particular URL, the corresponding servlet processes the request. This mapping can be configured in the web.xml file (deployment descriptor) or using annotations in the servlet class. For example:
Using web.xml:
xml
<servlet>
<servlet-name>MyServlet</servlet-name>
<servlet-class>com.example.MyServlet</servlet-class>
</servlet>
<servlet-mapping>
<servlet-name>MyServlet</servlet-name>
<url-pattern>/myServlet</url-pattern>
</servlet-mapping>
Using Annotations:
java
@WebServlet(“/myServlet”)
public class MyServlet extends HttpServlet {
// Servlet code here
}
Java Server Pages (JSP): clarify Web Applications
Java Server Pages (JSP) is a technology enabling developers to generate dynamic web pages through the use of Java. It simplifies the development of web applications by separating the user interface (UI) from the business logic, making the code more maintainable and scalable. JSP is part of the Java EE (Enterprise Edition) platform and integrates seamlessly with other Java technologies, such as Servlets and Enterprise JavaBeans (EJBs), to create robust, enterprise-level web applications.
What is JSP?
JSP is a server-side technology that enables the generation of dynamic HTML, XML, or other content by embedding Java code directly into HTML pages. Unlike traditional HTML, which is static, JSP allows Java code to be inserted within special tags, so the server can process this code and generate dynamic content for the client. JSP files have the extension .jsp, and when a client requests a JSP page, the server processes the Java code within it and sends back the result to the client.
How JSP Works
JSP works by combining static HTML with dynamic Java code. The process involves several steps:
- Request: A client sends an HTTP request to the server for a JSP page.
- Compilation: In the event that the requested JSP page has not been previously compiled, the JSP engine will convert it into a Java Servlet.. This is a one-time operation, which converts the JSP file into a servlet that can process requests.
- Execution: The generated servlet processes the Java code embedded in the JSP file. It executes the logic (e.g., interacting with databases, processing forms) and generates the response, which is typically HTML.
- Response: The server transmits the dynamically created HTML content to the client, where it is rendered in the browser.
JSP Elements
JSP allows developers to embed Java code in several ways using different types of elements:
1.Directives: These elements provide global information about the JSP page, such as the language, page encoding, and error handling. A directive is placed at the top of the page and is defined using <%@ %>. Example:
jsp
<%@ page language=”java” contentType=”text/html; charset=ISO-8859-1″ %>
2.Declarations: Java code can be declared within the JSP page using <%! %>. Declarations define methods or variables that are accessible throughout the page.
Jsp
<%! int count = 0; %>
3.Scriplets: These are Java code snippets embedded in the JSP page using <% %>. Scriplets can execute logic, such as loops or conditionals, but the result is embedded in the output stream.
jsp
<% for (int i = 0; i < 10; i++) { %>
<p>Number: <%= i %></p>
<% } %>
4.Expressions: These are used to output data directly to the response stream.The Java expression is evaluated, and the result is converted to a string and included in the output.. This is written using <%= %>.
Jsp
<%= “Hello, World!” %>
JSP vs. Servlets
While both JSP and Servlets are Java technologies for building web applications, they have key differences:
Servlets are Java classes used to handle requests and responses, typically generating HTML dynamically. The Java code in Servlets is written entirely in Java, which can make them less efficient when trying to manage the presentation layer.
JSP, on the other hand, allows for embedding Java code into HTML, making it easier to create user interfaces. JSP separates the presentation layer (HTML) from the business logic (Java code), allowing developers to focus more on designing the UI rather than dealing with Java logic directly within the page.
Advantages of JSP
- Separation of Concerns: JSP promotes the separation of business logic from the user interface. The Java code is separated from the HTML structure, which makes the code cleaner, easier to maintain, and scalable. This separation allows frontend developers to focus on the layout while backend developers can handle the business logic in Java classes or beans.
- Ease of Development: JSP makes it easier to develop web applications by simplifying the embedding of dynamic content. The syntax is straightforward, especially when compared to traditional server-side scripting languages like PHP, as JSP integrates seamlessly with Java.
- JSP promotes reusability and maintainability by utilizing tag libraries, including the JavaServer Pages Standard Tag Library (JSTL), as well as custom tags. This allows developers to reuse common functionality across multiple JSP pages, improving maintainability and reducing code duplication.
- Performance: Once a JSP page is compiled into a Servlet, it behaves much like any other servlet in terms of performance. Since the compilation occurs only once, subsequent requests are processed faster.
- Integration with Java EE: JSP works well with other Java EE technologies, such as EJB, JPA (Java Persistence API), and JDBC (Java Database Connectivity), allowing developers to build full-fledged, enterprise-level applications with ease.
Exploring Enterprise JavaBeans (EJB) for Enterprise Solutions
Enterprise JavaBeans (EJB) is a Java technology used to simplify the development of large-scale, distributed, and transactional enterprise applications. EJB is part of the Java EE (Enterprise Edition) platform, providing a set of services for building and deploying robust, secure, and scalable applications in Java. It allows developers to focus on business logic while the underlying platform handles common tasks like transaction management, security, and concurrency.
What is EJB?
An Enterprise JavaBean is a server-side component that encapsulates business logic in a Java application. EJBs are designed to be used in distributed, multi-tiered applications, where the business logic is separated from the user interface and data access layers. EJBs provide a standard way to build reusable and scalable business components that can be easily integrated into larger enterprise systems.
Types of Enterprise JavaBeans
EJBs come in different types, each serving specific purposes in enterprise applications:
- Session Beans: These are used to handle business logic and typically represent a single client interaction. They can be stateless or stateful.
Stateless Session Beans do not maintain any client-specific state between method calls. They are typically used for operations that do not depend on the client’s previous interactions.
Stateful Session Beans, on the other hand, maintain client-specific state across method calls, making them suitable for long-running conversations with clients.
Message-Driven Beans (MDBs): These are used to handle asynchronous messaging in enterprise applications. They allow applications to process messages (e.g., from a queue or topic) without requiring immediate responses, enabling more scalable and decoupled systems.
Entity Beans (deprecated in newer versions of EJB): These were used to represent persistent data and map it to Java objects, enabling interaction with a database. However, Entity Beans have been replaced by Java Persistence API (JPA) for persistence management.
Key Features of EJB
- Transaction Management: EJBs provide built-in support for managing transactions, ensuring that operations are completed successfully or rolled back in case of failure. This is crucial in enterprise applications where consistency and reliability are paramount.
- Security: EJBs integrate with the Java EE security model to provide declarative security, such as authentication and authorization. Developers can specify security policies in the deployment descriptor or via annotations, ensuring that only authorized users can access specific business methods.
- Concurrency: EJBs handle concurrency control, meaning multiple clients can invoke methods concurrently without causing issues like data corruption. The container ensures thread safety when handling requests.
Scalability: EJBs are designed to scale in enterprise environments. They can be clustered to handle high loads, ensuring that applications remain performant even under heavy traffic.
Benefits of EJB
- Simplified Development: EJBs abstract away much of the complexity involved in building enterprise applications. Developers can focus on writing business logic while the EJB container handles services like transactions, security, and concurrency.
- Reusability: EJBs can be reused across multiple applications or components, promoting modular design and reducing code duplication.
- Integration: EJBs integrate seamlessly with other Java EE technologies, such as Java Database Connectivity (JDBC), Java Message Service (JMS), and Java Naming and Directory Interface (JNDI), making them an essential component of Java-based enterprise solutions.
Java Messaging Service (JMS): Asynchronous Communication Made Easy
In the world of enterprise applications, communication between different systems and components is essential. Traditional communication methods like synchronous HTTP or direct database connections can become slow and inefficient when dealing with complex, large-scale systems. This is where Java Messaging Service (JMS) comes in, offering a robust and scalable way to handle asynchronous communication between different parts of an application or across applications.
What is JMS?
Java Messaging Service (JMS) is an Application Programming Interface (API) that enables Java applications to asynchronously send and receive messages. JMS is part of the Java EE (Enterprise Edition) platform and provides a standard way for Java applications to communicate through messaging systems like queues and topics, which are the foundation of message-oriented middleware (MOM). With JMS, applications can send messages without having to wait for immediate responses, making it ideal for handling long-running or time-consuming operations.
Synchronous vs. Asynchronous Communication
Before diving into JMS, it’s important to understand the difference between synchronous and asynchronous communication:
Synchronous communication: occurs when the sender awaits a reply from the receiver prior to proceeding.continuing. This approach can lead to bottlenecks and reduced performance if there are delays.
Asynchronous communication: occurs when the sender does not await a reply.. It sends a message and continues its task. The receiver processes the message later and sends a response, if needed. Asynchronous communication enables better scalability and flexibility, particularly in distributed systems.
JMS makes asynchronous communication straightforward, allowing messages to be queued up for processing at a later time.
Key Components of JMS
JMS uses two main types of messaging models to deliver messages:
Point-to-Point (Queue-based):
In this model, a message is sent to a queue, and only one consumer retrieves the message. It’s a one-to-one communication model where the sender and receiver don’t need to be aware of each other’s existence.
Example: A customer service system where orders are placed in a queue, and the next available customer service agent processes the order.
Publish-Subscribe (Topic-based):
In this model, messages are sent to a topic, and multiple consumers can receive the message. This allows for one-to-many communication, where multiple subscribers can act on the same message.
Example: A stock price alert system where different clients (subscribers) receive updates on stock prices from a central service (publisher).
How Does JMS Work?
JMS allows for the creation, sending, receiving, and reading of messages between Java applications. Here’s how the messaging process typically works:
- Message Producer: The sender or producer creates a message and sends it to a destination (either a queue or a topic).
- The message consumer is responsible for obtaining the message from its designated location and subsequently processing it.
- Message Broker: A JMS-compliant message broker or server (such as ActiveMQ, RabbitMQ, or IBM MQ) handles the routing of messages between producers and consumersIt guarantees the delivery of messages, even when the recipient is momentarily inaccessible.
Advantages of JMS
Asynchronous Communication: By allowing messages to be sent and received asynchronously, JMS helps to decouple different components of an application, improving overall system performance and reliability. The sender can continue with other tasks while the receiver processes the message later.
Reliability: JMS guarantees message delivery, even in case of system failures. Message brokers often provide features like persistent messaging (where messages are stored until delivered) and acknowledgments, ensuring that messages are not lost.
Scalability: JMS can handle a high volume of messages, making it suitable for distributed, large-scale systems. The ability to use queues and topics means that JMS can support many different consumers, load balancing, and fault tolerance.
Loose Coupling: JMS decouples the sender and receiver, meaning the producer does not need to know about the consumer’s existence or state. This allows for flexible and scalable architectures, as components can evolve independently.
Integration: JMS is widely supported and integrates seamlessly with many enterprise systems, including database systems, web services, and enterprise service buses (ESBs). It’s commonly used in message-driven beans (MDBs) within Java EE applications to enable reactive processing.
Use Cases of JMS
JMS is widely used in various scenarios, especially in enterprise applications where asynchronous communication is critical:
Order Processing Systems: In an e-commerce platform, when a user places an order, the system can send an order message to a queue that is processed asynchronously by a backend service.
Stock Trading Platforms: Messages about stock price changes or trades can be published to a topic, and multiple clients (such as trading applications or alerts) can subscribe to receive real-time updates.
Inventory Management Systems: In large retail environments, updates about stock levels can be sent asynchronously to different parts of the system for real-time inventory management.
Conclusion
In conclusion, an advanced Java programming syllabus serves as an essential roadmap for mastering Java’s complex concepts and tools. By covering a range of topics from advanced object-oriented programming principles to sophisticated frameworks, multi-threading, design patterns, and data management, this syllabus ensures that learners gain the skills necessary to build robust, efficient, and scalable applications. Emphasizing hands-on practice and real-world project development, it prepares students and professionals to tackle challenges in modern software development. As technology continues to evolve, mastering advanced Java techniques positions developers for success in both academic and professional settings.
FAQs
What is Advanced Java Programming?
Advanced Java Programming focuses on concepts beyond the basics of Java, including topics such as multi-threading, networking, Java databases (JDBC), JavaFX, and web development frameworks like Spring.
What prerequisites are needed for this course?
Basic knowledge of Java fundamentals, such as variables, control structures, object-oriented programming (OOP), and core libraries, is essential before diving into advanced topics.
What topics are covered in Advanced Java Programming?
- Multi-threading and concurrency
- Networking (Sockets and HTTP)
- Java Database Connectivity (JDBC)
- JavaFX for graphical user interfaces
- Spring Framework for web development
- Java Web Services (REST, SOAP)
- Design patterns and best practices
How is this course different from basic Java?
While basic Java focuses on understanding core concepts like variables and loops, advanced Java introduces complex topics like handling multi-threading, building enterprise-level applications, and integrating Java with databases and web services.
Do I need to know other programming languages for this course?
No, knowing other languages is not required. The focus is on deepening your understanding of Java, though familiarity with HTML, CSS, and JavaScript may be helpful for web-based topics.
How is the course structured?
The course typically starts with advanced OOP principles and gradually introduces more complex topics like multi-threading, database integration, and web development.
What tools and environments are used?
You will likely use an Integrated Development Environment (IDE) like IntelliJ IDEA or Eclipse and tools such as Maven for project management, and databases like MySQL or PostgreSQL for JDBC.
How much coding is involved in this course?
The course involves significant hands-on coding to practice concepts such as creating multi-threaded applications, working with databases, and building Java-based web applications.
What is JavaFX, and why is it important in this course?
JavaFX is a library for building rich graphical user interfaces (GUIs). It is important for creating modern desktop applications with Java.
What is JDBC, and why do we need it in Advanced Java?
Java Database Connectivity (JDBC) is an API that allows Java programs to interact with databases. It is essential for developing database-driven applications, like e-commerce platforms or inventory management systems.
What are design patterns, and why should I learn them?
Design patterns are reusable solutions to common software design problems. Learning them helps you write clean, maintainable, and efficient code.
Will I learn about web development in this course?
Yes, topics like Java-based web frameworks (Spring, Hibernate) and web services (REST, SOAP) are covered to help you build robust web applications.
Is there a project or final exam in the course?
Many advanced Java courses include a final project where students apply the skills learned throughout the course to build a full-fledged application or system.
Can I take this course online?
Yes, many universities and online platforms offer advanced Java programming courses. You can learn at your own pace with video lectures, assignments, and forums for support.
What career opportunities can I pursue after completing this course?
After mastering advanced Java, you can pursue roles like Java Developer, Backend Developer, Web Developer, or Software Engineer, with skills applicable to enterprise applications, web services, and more.