Front End Developer Projects
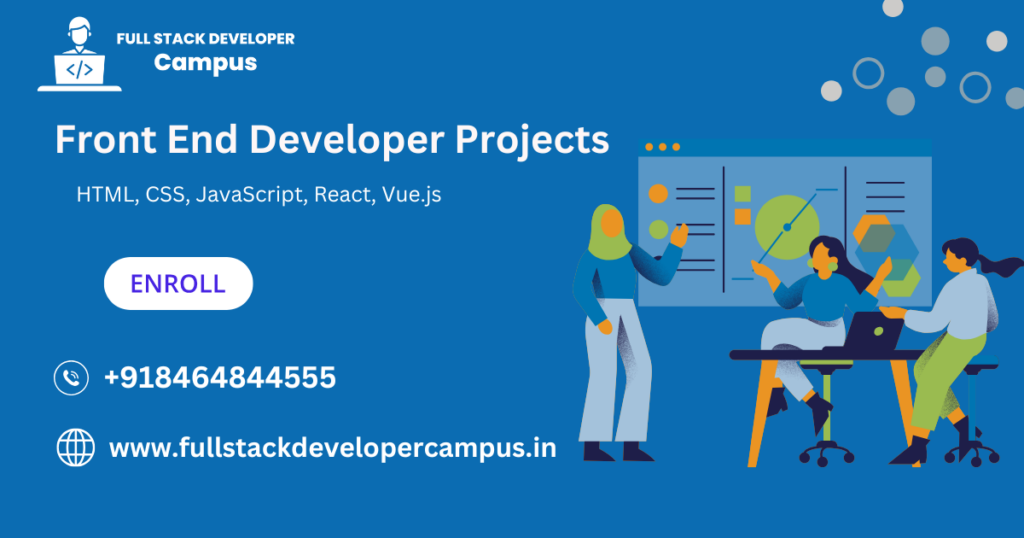
1) Project : Personal Portfolio Website
Objective:
Create a personal portfolio website using HTML and CSS to showcase skills, projects, and contact details.
Key Features:
Home Page: A brief introduction with an image and a short bio.
About Section: Details about skills, experience, and education.
Projects Section: Display completed front end developer projects with descriptions.
Contact Form: A simple form with fields for name, email, and message submission.
Responsive Design: Ensure the website adapts to different screen sizes using CSS Grid and Flexbox.
This front end developer project helps beginners understand semantic HTML, layout techniques, and responsive design while building a real-world portfolio.
2) Project : Interactive To-Do List
Objective:
Build a front end developer project that allows users to add, edit, delete, and mark tasks as completed using JavaScript.
Key Features:
Task Addition: Users can input a task and add it to the list dynamically.
Mark as Completed: Clicking a task toggles its completion status.
Edit & Delete Options: Users can update or remove tasks.
Local Storage Integration: Save tasks so they persist even after refreshing the page.
Event Handling & DOM Manipulation: Use JavaScript to update the UI dynamically.
This front end developer project strengthens ES6 concepts, event handling, and local storage usage, making it an ideal practice project for beginners.
3) Project : Collaborative Blog Repository
Objective:
Create a front end developer project where multiple developers contribute to a blog repository using Git and GitHub.
Key Features:
Project Setup: Initialize a Git repository and push it to GitHub.
Branching & Merging: Each contributor works on separate branches before merging changes.
Pull Requests: Contributors submit pull requests for code review before merging into the main branch.
Version Control: Track changes using commits and revert to previous versions if needed.
Markdown for Blog Posts: Store content in markdown files (.md) for easier collaboration.
This front end developer project enhances Git workflow knowledge, teaching best practices for version control and teamwork in real-world development.
4) Project: Responsive Landing Page
Objective:
Create a front end developer project using Bootstrap or Tailwind CSS to build a fully responsive landing page for a product or service.
Key Features:
Navigation Bar: A sticky header with smooth scrolling.
Hero Section: A visually appealing introduction with a call-to-action button.
Features Section: Display key product/service features using Bootstrap’s grid system or Tailwind’s utility classes.
Testimonials: A carousel or grid-based section showcasing user reviews.
Contact Form: A functional form styled with SASS or SCSS.
Fully Responsive Design: Ensure proper layout on mobile, tablet, and desktop.
This front end developer project helps developers master CSS frameworks and preprocessors, enabling faster and more efficient UI development.
5) Project : Weather App with API Integration
Objective:
Create a front end developer project that fetches real-time weather data using an external API while implementing advanced JavaScript concepts like promises, asynchronous programming, and local storage.
Key Features:
Search Functionality: Users can enter a city name to get weather details.
API Integration: Use the Fetch API to retrieve data from a weather API.
Asynchronous Data Handling: Utilize async/await for smooth API calls.
Local Storage: Save the last searched city so users can see the weather instantly on the next visit.
Dynamic UI Updates: Display temperature, humidity, and weather conditions dynamically.
Error Handling: Show messages for invalid city names or network issues.
This front end developer project helps in mastering asynchronous programming, API handling, and data persistence, which are essential for modern web development.
6) Project : Interactive Quiz App
Objective:
Build a front end developer project using React that allows users to take quizzes, track scores, and get instant feedback.
Key Features:
Component-Based Architecture: Use reusable React components for questions, answers, and results.
Props & State Management: Pass question data via props and handle responses using useState.
React Hooks: Implement useEffect for managing side effects, such as fetching quiz questions.
Score Tracking: Update and display the user’s score dynamically.
Timer Functionality (Optional): Add a countdown timer for each question using useEffect and setTimeout.
Responsive UI: Use CSS frameworks like Tailwind CSS or Bootstrap for a clean design.
This front end developer project helps in understanding React components, props, state management, and hooks, which are essential for modern web applications.
7) Project : Movie Search App
Objective:
Develop a front end developer project that allows users to search for movies using an external API, implementing REST API integration, Axios, and Fetch.
Key Features:
Search Functionality: Users can enter a movie name to fetch details.
REST API Integration: Use the OMDb API or TMDB API to retrieve movie data.
Axios & Fetch Usage: Implement both Axios and Fetch API to understand their differences.
Dynamic UI Updates: Display movie posters, titles, ratings, and descriptions.
Loading & Error Handling: Show a loading animation while fetching data and handle errors gracefully.
Favorites Feature: Allow users to save favorite movies using local storage.
This front end developer project strengthens skills in API integration, data fetching, and asynchronous JavaScript, which are crucial for real-world applications.
8) Project : Secure Login & Registration System
Objective:
Build a front end developer project with secure authentication using OAuth, JWT authentication, and role-based access.
Key Features:
User Registration & Login: Implement a signup and login form with email/password authentication.
JWT Authentication: Store and manage user sessions securely using JSON Web Tokens (JWT).
OAuth Integration: Allow users to log in via Google, Facebook, or GitHub authentication.
Role-Based Access: Differentiate user roles (admin, regular user) with restricted access to certain pages.
HTTPS & Secure Storage: Encrypt user data and store authentication tokens securely in HTTP-only cookies.
Session Management: Implement auto-logout when a token expires.
This front end developer project improves skills in authentication, security, and role-based access, which are critical for protecting user data in modern web applications.
9) Project : Optimized Image Gallery
Objective:
Develop a front end developer project that loads images efficiently using lazy loading, code splitting, and minification to improve performance.
Key Features:
Lazy Loading: Load images only when they appear in the viewport, reducing initial page load time.
Code Splitting: Use React Suspense & Lazy (for React) or dynamic imports (for vanilla JavaScript) to load components only when needed.
Minified Assets: Optimize CSS and JavaScript by using tools like Terser, UglifyJS, or Webpack for minification.
Optimized Images: Use WebP format and compress images to reduce file sizes without losing quality.
Caching & CDN Usage: Implement service workers or a CDN to store and serve assets faster.
This front end developer project enhances skills in performance optimization techniques, ensuring a smooth and efficient user experience in modern web applications.
10) Project :Personal Blog Website Deployment
Objective:
Deploy a front end developer project on Netlify or Vercel with SEO optimization and a CI/CD pipeline for continuous updates.
Key Features:
Static Site Generation: Use Next.js (or plain HTML/CSS/JS) for better performance.
Deployment on Netlify/Vercel: Host the site for free with automatic updates from GitHub.
CI/CD Integration: Set up GitHub Actions or Vercel auto-deploy to push changes automatically.
SEO Optimization:
Use proper meta tags (title, description, keywords).
Implement Open Graph (OG) tags for social media previews.
Generate an XML sitemap for search engines.
Custom Domain: Connect a custom domain (e.g., from Namecheap or GoDaddy).
This front end developer project helps in real-world deployment, CI/CD integration, and SEO, making a website accessible and scalable.
11) Project :Offline Notes App
Objective:
Develop a front end developer project that allows users to create, save, and manage notes even without an internet connection, using Progressive Web App (PWA) technologies.
Key Features:
Service Workers: Cache the application assets to make it available offline.
Web App Manifest: Enable installation on mobile and desktop like a native app.
Local Storage/IndexedDB: Save notes locally when offline and sync them when online.
Push Notifications: Send reminders or alerts for important notes.
Dark Mode Support: Provide a toggle for better accessibility.
Responsive Design: Ensure the app works seamlessly on mobile and desktop.
This front end developer project improves skills in PWA development, offline support, and local data management, making it a great choice for modern web applications.
12) Project :E-commerce Cart System
Objective:
Build a front end developer project that manages an e-commerce shopping cart using Redux Toolkit and React Context API for efficient state management.
Key Features:
Product Listing Page: Display a list of products fetched from an API or local JSON data.
Add to Cart Functionality: Allow users to add/remove items from the cart dynamically.
Redux Toolkit Integration: Use Redux slices to manage global cart state.
Context API for Theme & User Preferences: Implement a lightweight Context API for non-cart data like theme selection or user preferences.
Persistent Cart Storage: Store the cart in local storage to retain items on page refresh.
Total Price Calculation: Update the cart total in real time.
Checkout Process Simulation: Provide a simple checkout page with order summary.
This front end developer project enhances skills in state management, Redux Toolkit, Context API, and global state handling, making it an ideal real-world learning experience.
13) Project :Interactive Product Showcase
Objective:
Create a front end developer project that enhances product display using animations and micro-interactions with CSS transitions and Framer Motion in React.
Key Features:
Hover Effects: Use CSS animations and transitions to highlight product images on hover.
Framer Motion Animations: Implement smooth fade-ins, slide-ins, and scale effects when a product is viewed.
Image Carousel: Add an interactive image slider with auto-scroll and swipe support.
3D Flip Cards: Use CSS transforms to create flipping product cards with front and back details.
Click Animations: Animate buttons and UI elements for better user feedback.
Lazy Loading Images: Optimize performance by loading images only when needed.
Dark Mode Support: Smooth transitions between light and dark themes.
This front end developer project strengthens skills in CSS animations, Framer Motion, and UI/UX enhancements, improving user engagement and visual appeal.
14) Project :Test-Driven Form Validation
Objective:
Develop a front end developer project focused on form validation using a test-driven development (TDD) approach with Jest, React Testing Library, and Cypress for unit and end-to-end (E2E) testing.
Key Features:
User Input Validation: Validate fields like name, email, password, and phone number.
Real-Time Error Handling: Show error messages dynamically as users type.
Jest & React Testing Library: Write unit tests for validation functions and form components.
Cypress E2E Testing: Automate full user interactions, including form submission and error handling.
Mock API Testing: Simulate API responses to check validation with real-world scenarios.
Accessibility Testing: Ensure the form is accessible (a11y) for all users.
This front end developer project enhances skills in test-driven development (TDD), automated testing, and form validation, ensuring a robust and bug-free user experience.
15) Project :Multi-Language Blog Website
Objective:
Develop a front end developer project that ensures accessibility (a11y) and supports multiple languages (i18n) using best web development practices.
Key Features:
Language Switcher: Implement an i18n library (e.g., react-i18next) to support multiple languages.
Semantic HTML & ARIA Labels: Use proper heading structures, alt text, and ARIA roles to enhance accessibility.
Keyboard Navigation: Ensure users can navigate using only the keyboard (Tab, Enter, Space).
Screen Reader Compatibility: Test with screen readers (e.g., NVDA, VoiceOver) to ensure clarity.
Contrast & Font Adjustments: Provide high-contrast mode and font size adjustments for visually impaired users.
Right-to-Left (RTL) Support: Adapt layouts for RTL languages like Arabic and Hebrew.
SEO Optimization: Ensure metadata, structured data, and best SEO practices are followed.
This front end developer project enhances skills in web accessibility (a11y), internationalization (i18n), and UX best practices, making it inclusive and user-friendly for diverse audiences.
Conclusion
Mastering front end developer projects is essential for building real-world applications and improving development skills. This module-wise breakdown covers key aspects like HTML, CSS, JavaScript, React, API integration, authentication, state management, animations, testing, and deployment.
By working on projects like a portfolio website, e-commerce cart system, interactive product showcase, progressive web apps (PWA), and accessibility-friendly blog websites, developers gain hands-on experience in modern web development.
Focusing on performance optimization, security, and accessibility ensures that applications are fast, secure, and user-friendly. Additionally, incorporating testing methodologies and CI/CD pipelines helps maintain code quality.
By following this structured front end developer project roadmap, developers can confidently build scalable, optimized, and interactive web applications, preparing for real-world challenges in the tech industry.
FAQs
1. What are front end developer projects?
Front end developer projects involve designing and developing the user interface (UI) of websites and web applications using HTML, CSS, JavaScript, and frameworks like React, Angular, or Vue.js. These projects focus on building interactive, visually appealing, and responsive web experiences.
2. Why are front end projects important for learning?
Front end projects help developers:
- Apply theoretical concepts in real-world scenarios.
- Improve their problem-solving and debugging skills.
- Build a portfolio to showcase expertise.
- Gain practical experience with industry-standard tools like Git, APIs, and state management solutions.
3. What are some beginner-friendly front end projects?
- Personal Portfolio Website – Showcasing skills and past work.
- To-Do List App – Managing tasks with local storage.
- Weather App – Fetching live weather data from an API.
- Landing Page – Practicing CSS layouts and responsiveness.
4. What are some advanced front end projects?
- E-commerce Website – Integrating product listings, cart, and payment gateway.
- Chat Application – Using WebSockets or Firebase for real-time communication.
- Dashboard for Analytics – Displaying charts and statistics with data visualization libraries.
- Progressive Web App (PWA) – Creating an installable, offline-capable web app.
5. How can I improve my front end projects?
- Write clean and maintainable code following best practices.
- Make projects responsive for mobile and desktop screens.
- Implement performance optimization by reducing unnecessary code and assets.
- Follow accessibility (a11y) guidelines to make the UI usable for all users.
- Use Git and GitHub for version control and collaboration.
6. What technologies should I learn for front end development?
- Core Web Technologies: HTML, CSS, JavaScript.
- CSS Frameworks: Bootstrap, Tailwind CSS, Material UI.
- JavaScript Frameworks & Libraries: React, Next.js, Vue.js, Angular.
- State Management: Redux, Context API, Recoil.
- API Handling: REST APIs, GraphQL, Axios, Fetch.
- Testing Tools: Jest, React Testing Library, Cypress.
7. How do I deploy my front end projects?
- Netlify & Vercel – Best for React, Next.js, and static sites.
- GitHub Pages – Free hosting for personal projects.
- Firebase Hosting – Serverless hosting for dynamic applications.
- AWS Amplify & DigitalOcean – Suitable for larger projects requiring scalability.
8. What are the best platforms to find front end developer project ideas?
- Frontend Mentor – Real-world projects with design specifications.
- DevChallenges.io – Hands-on coding challenges for developers.
- CodePen & Dribbble – Explore UI/UX designs for inspiration.
- GitHub Trending Repositories – Learn from open-source front end projects.
9. What are the key skills needed for front end developer projects?
- UI/UX Design Understanding – Creating visually appealing layouts.
- Responsive Web Development – Ensuring usability across different devices.
- JavaScript & Frameworks – Implementing dynamic features.
- Version Control (Git/GitHub) – Tracking changes and collaborating.
Debugging & Testing – Ensuring code reliability with automated tests.
10. How can I make my front end projects unique?
- Add custom animations and micro-interactions using CSS or Framer Motion.
- Improve performance and SEO optimization for faster loading times.
- Implement dark mode and accessibility features for a better user experience.
- Use modern UI design trends to make your project visually appealing.
11. What are Progressive Web Apps (PWAs) in front end development?
PWAs are web applications that provide a native app-like experience by supporting:
- Offline functionality using Service Workers.
- Push notifications to engage users.
- App installation on mobile and desktop devices.
12. How important is front end testing?
Testing helps identify and fix bugs early, ensuring a smooth user experience. Front end developers use:
- Jest & React Testing Library for unit testing.
- Cypress & Selenium for end-to-end testing.
- Lighthouse Audits for performance and accessibility testing.
13. How can I monetize my front end development skills?
- Freelancing – Work on platforms like Upwork, Fiverr, or Toptal.
- Building SaaS Applications – Develop and sell your own web apps.
- Teaching & Blogging – Share knowledge on YouTube, Medium, or a personal blog.
- Selling Templates & UI Kits – Create and sell website themes or components.
14. How long does it take to become proficient in front end development?
The timeline varies based on experience and dedication:
- 3-6 months – Basic understanding of HTML, CSS, JavaScript.
- 6-12 months – Proficiency in React, state management, and API integration.
- 1+ year – Advanced skills in performance optimization, testing, and scalability.
15. What is the future of front end development?
Front end development is evolving with trends like:
- AI-driven UI development – Using AI tools to automate design and code generation.
- Web3 & Blockchain Integration – Decentralized applications (DApps).
- Enhanced Performance with WASM – Running high-performance applications in the browser.
- Server-side Rendering (SSR) & Static Site Generation (SSG) – Faster page loads with frameworks like Next.js.