Full Stack Java Developer Roadmap
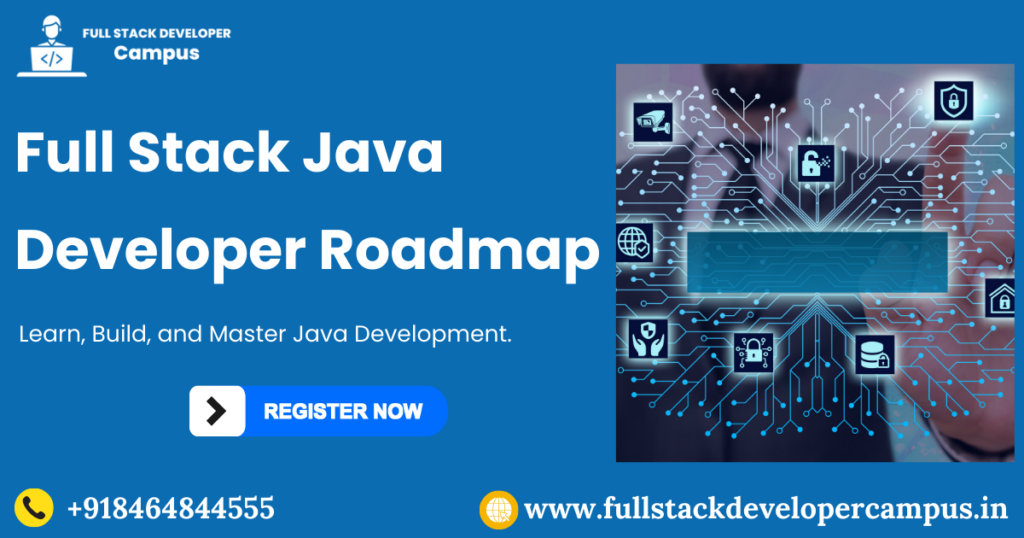
Full Stack Java Developer Roadmap
Becoming a skilled Full Stack Java Developer requires mastering various technologies, tools, and frameworks.
A structured Full-Stack Java Developer Roadmap helps developers understand the essential skills needed for front-end, back-end, and database management. Following a clear Roadmap ensures efficient learning and career growth.
1. Learn Core Java Fundamentals
The first step in the Full Stack Java Developer Roadmap is understanding Core Java concepts like Object-Oriented Programming (OOP), collections, multithreading, and exception handling. A strong foundation in Core Java is essential for back-end development.
2. Master Front-End Technologies
A Full Stack Java Developer Roadmap includes front-end skills like HTML, CSS, and JavaScript. Learning frameworks like React.js or Angular enhances user interface development, making applications interactive and responsive.
3. Back-End Development with Java
In the Full Stack Java Developer Roadmap, mastering Java frameworks like Spring Boot is crucial. Spring Boot simplifies web development by offering built-in support for RESTful APIs, making Java a preferred choice for scalable applications.
4. Database Management
A complete Full Stack Java Developer Roadmap includes SQL (MySQL, PostgreSQL) and NoSQL (MongoDB) databases. Hibernate, a Java-based ORM tool, helps developers manage database interactions efficiently.
5. API Development and Security
A well-structured Full Stack Java Developer Roadmap emphasizes building secure RESTful APIs using Spring Boot. Implementing authentication and authorization with JWT and OAuth enhances application security.
6. Version Control and DevOps
In the Full Stack Java Developer Roadmap, learning Git, GitHub, Docker, and CI/CD pipelines helps in efficient code deployment and collaboration.
7. Continuous Learning and Growth
Following a Full Stack Java Developer Roadmap ensures long-term success. Keeping up with new trends, tools, and best practices enhances career opportunities in Java full-stack development.
Back-End Development with Java
Back-end development plays a crucial role in the Full Stack Java Developer Roadmap as it handles the server-side logic, database management, and API integration. A well-structured Full Stack Java Developer Roadmap ensures that developers gain expertise in Java-based back-end technologies to build robust and scalable applications.
1. Core Java for Back-End Development
The Full Stack Java Developer Roadmap starts with mastering Core Java concepts like object-oriented programming (OOP), exception handling, collections framework, and multithreading. These fundamentals help developers create efficient back-end logic.
2. Spring Framework and Spring Boot
A critical step in the Full Stack Java Developer Roadmap is learning Spring Framework and Spring Boot. Spring Boot simplifies back-end development by providing built-in functionalities like dependency injection, RESTful API support, and microservices architecture.
3. Database Management with Java
Managing data effectively is essential in the Full Stack Java Developer Roadmap. Developers should learn SQL databases like MySQL and PostgreSQL, as well as NoSQL databases like MongoDB. Using Hibernate, a Java-based ORM tool, improves database interactions.
4. Building and Securing RESTful APIs
In the Full Stack Java Developer Roadmap, API development is key for connecting the front-end and back-end. Java developers should learn to create RESTful APIs using Spring Boot and secure them with JWT and OAuth authentication.
5. Deployment and DevOps Integration
The Full Stack Java Developer Roadmap also includes DevOps practices such as using Git, Docker, and CI/CD pipelines for efficient deployment and version control.
Introduction to Full Stack Java Development
In today’s software industry, Full Stack Java Development is one of the most in-demand skills. A Full Stack Java Developer Roadmap helps developers understand the technologies required to build dynamic, scalable applications. A Full Stack Java Developer Roadmap includes front-end, back-end, database management, and deployment skills, making developers proficient in handling complete web application development.
1. What is Full Stack Java Development?
A Full Stack Java Developer is someone who can work on both the client-side (front-end) and server-side (back-end) of an application. The Full Stack Java Developer Roadmap includes learning Java programming, web technologies, databases, APIs, and DevOps tools.
2. Why Choose Java for Full Stack Development?
Java is widely used in enterprise applications due to its security, platform independence, and scalability. The Full Stack Java Developer Roadmap focuses on Java’s back-end frameworks like Spring Boot, which simplifies web development and microservices architecture.
3. Essential Technologies in the Full Stack Java Developer Roadmap
A Full Stack Java Developer Roadmap includes:
Front-End: HTML, CSS, JavaScript, React.js, or Angular
Back-End: Core Java, Spring Boot, RESTful APIs
Databases: MySQL, PostgreSQL, MongoDB
DevOps: Git, Docker, Kubernetes, CI/CD
4. Career Scope in Full Stack Java Development
Following a Full Stack Java Developer Roadmap opens multiple career opportunities in web development, cloud computing, and microservices. Companies prefer full-stack developers as they can handle both front-end and back-end tasks efficiently.
Core Java Fundamentals
Mastering Core Java Fundamentals is the first step in the Full Stack Java Developer Roadmap. A strong foundation in Core Java Fundamentals helps developers build efficient back-end logic, handle data processing, and develop scalable applications. The Full Stack Java Developer Roadmap emphasizes learning essential Java concepts to ensure smooth web development.
1. Object-Oriented Programming (OOP) in Java
A key part of Core Java Fundamentals is understanding OOP principles, including:
Encapsulation – Protecting data using access modifiers
Inheritance – Reusing code from parent to child classes
Polymorphism – Implementing method overloading and overriding
Abstraction – Hiding implementation details
The Full Stack Java Developer Roadmap includes these OOP concepts as they form the backbone of Java-based applications.
2. Exception Handling in Java
Handling runtime errors is crucial in Core Java Fundamentals. The Full Stack Java Developer Roadmap recommends mastering try-catch, throws, and finally to ensure application stability.
3. Collections Framework
Efficient data handling is an important part of Core Java Fundamentals. Java’s Collections Framework includes:
List (ArrayList, LinkedList) – Stores ordered data
Set (HashSet, TreeSet) – Stores unique elements
Map (HashMap, TreeMap) – Stores key-value pairs
The Full Stack Java Developer Roadmap includes Collections for managing complex data structures.
4. Multithreading and Concurrency
Multithreading enhances performance in Java applications. Learning Thread, Runnable, and ExecutorService is essential in the Full Stack Java Developer Roadmap.
Mastering Core Java Fundamentals is a must for any developer following the Full Stack Java Developer Roadmap. A solid understanding of these concepts enables Java developers to build efficient, secure, and scalable applications.
Database Management
Database management is a crucial part of the Full Stack Java Developer Roadmap, as it enables efficient storage, retrieval, and manipulation of data. A well-structured Full Stack Java Developer Roadmap includes learning both relational and non-relational databases to handle different types of applications. Understanding database management is essential for building scalable, secure, and high-performance Java applications.
1. Types of Databases
In the Full Stack Java Developer Roadmap, developers must learn two main types of databases:
Relational Databases (SQL): MySQL, PostgreSQL
NoSQL Databases: MongoDB, Cassandra
Relational databases use structured tables and SQL queries, while NoSQL databases handle unstructured or semi-structured data, making them ideal for real-time applications.
2. SQL for Database Management
A Full Stack Java Developer Roadmap includes SQL concepts such as:
CRUD Operations: Create, Read, Update, Delete
Joins & Relationships: Managing multiple tables
Indexes & Optimization: Improving query performance
3. ORM with Hibernate
A critical aspect of the Full Stack Java Developer Roadmap is learning Hibernate, an ORM (Object-Relational Mapping) tool that simplifies database interactions in Java. Hibernate eliminates the need for complex SQL queries by allowing developers to use Java objects to interact with databases.
4. Database Security & Best Practices
Security is a major concern in database management. The Full Stack Java Developer Roadmap recommends:
Using encryption for sensitive data
Implementing role-based access control
Preventing SQL injection attacks
Web Services and API Development
Web services and API development are essential components of the Full Stack Java Developer Roadmap. They enable communication between different applications, allowing seamless data exchange and integration. A strong understanding of Web Services and API Development is crucial for Java developers to build scalable and efficient web applications.
1. What are Web Services?
Web services are software components that allow applications to communicate over a network. In the Full Stack Java Developer Roadmap, web services play a key role in enabling interaction between front-end and back-end systems. Web services use standard communication protocols like HTTP and support different data formats such as JSON and XML.
Types of Web Services:
SOAP (Simple Object Access Protocol): Uses XML for data exchange and follows strict standards for security and reliability.
REST (Representational State Transfer): Uses HTTP methods (GET, POST, PUT, DELETE) and is widely used due to its simplicity and scalability.
2. Understanding APIs in the Full Stack Java Developer Roadmap
APIs (Application Programming Interfaces) are the building blocks of web services. They define the rules for communication between applications. In Web Services and API Development, APIs act as intermediaries that allow different software components to interact efficiently.
Key Features of APIs:
Statelessness: APIs do not store client data between requests.
Scalability: APIs handle large numbers of requests efficiently.
Security: APIs use authentication methods like JWT and OAuth.
3. RESTful API Development with Java
A Full Stack Java Developer Roadmap includes RESTful API development using Spring Boot, which simplifies API creation. RESTful APIs follow REST principles, making them lightweight and easy to integrate.
Steps to Build a REST API in Java:
Set up a Spring Boot project.
Define REST endpoints using @RestController.
Use HTTP methods (@GetMapping, @PostMapping, @PutMapping, @DeleteMapping).
Handle requests and responses using JSON.
Secure APIs with authentication mechanisms.
4. API Security Best Practices
Security is an essential aspect of Web Services and API Development in the Full Stack Java Developer Roadmap. Developers should implement security measures to protect sensitive data.
Common Security Techniques:
Authentication & Authorization: Use JWT (JSON Web Tokens) and OAuth for secure access.
Input Validation: Prevent SQL injection and cross-site scripting (XSS).
Rate Limiting: Prevent API abuse by limiting the number of requests per user.
5. Testing and Deploying APIs
The Full Stack Java Developer Roadmap emphasizes testing APIs before deployment. Popular testing tools include:
Postman: For API testing and request validation.
Swagger: For API documentation and interactive testing.
DevOps and Deployment
DevOps and deployment are critical components of the Full Stack Java Developer Roadmap, ensuring efficient software development, testing, and deployment. DevOps bridges the gap between development and operations teams, allowing smooth collaboration, faster releases, and high-quality software delivery. A structured Full Stack Java Developer Roadmap includes DevOps practices to help developers automate workflows, improve scalability, and enhance deployment efficiency.
1. What is DevOps?
DevOps is a software development approach that integrates development (Dev) and operations (Ops) teams to improve collaboration, automate workflows, and accelerate software delivery. It plays a crucial role in the Full Stack Java Developer Roadmap, as modern applications require continuous integration, deployment, and monitoring.
Key Principles of DevOps:
Continuous Integration (CI): Merging code changes frequently to avoid conflicts.
Continuous Deployment (CD): Automating the release process for faster delivery.
Infrastructure as Code (IaC): Managing infrastructure using code for scalability.
Monitoring & Logging: Ensuring application performance and security.
2. DevOps Tools in the Full Stack Java Developer Roadmap
A well-structured Full Stack Java Developer Roadmap includes mastering the following DevOps tools:
Version Control & Collaboration:
Git & GitHub: Tracking changes and managing code repositories.
Bitbucket & GitLab: Alternative Git-based platforms with CI/CD integration.
CI/CD Pipelines:
Jenkins: Automating code builds, testing, and deployments.
GitHub Actions: Streamlining workflows and CI/CD automation.
Containerization & Orchestration:
Docker: Packaging applications with dependencies to ensure consistent deployment.
Kubernetes: Managing containerized applications for scalability and high availability.
Cloud Deployment Platforms:
AWS (Amazon Web Services): Hosting and scaling Java applications.
Google Cloud & Azure: Alternative cloud platforms for deploying full-stack applications.
3. Steps for Java Application Deployment
The Full Stack Java Developer Roadmap includes several steps for deploying Java applications effectively:
Step 1: Prepare the Application for Deployment
Ensure the Java application is fully tested.
Use Maven or Gradle to build the project.
Step 2: Set Up CI/CD Pipelines
Use Jenkins or GitHub Actions to automate code integration and testing.
Automate testing using JUnit or Selenium.
Step 3: Containerization with Docker
Create a Dockerfile to package the application.
Build and run the Docker container for testing.
Step 4: Deploy to the Cloud
Use AWS EC2 or Kubernetes for cloud deployment.
Configure Nginx or Apache as a reverse proxy.
Step 5: Monitor and Maintain the Application
Use Prometheus & Grafana for performance monitoring.
Set up Logstash & Kibana for logging and error tracking.
4. Importance of DevOps in Full Stack Java Development
The Full Stack Java Developer Roadmap highlights DevOps as a key skill for modern developers. It helps in:
Faster releases: Automating testing and deployment speeds up the process.
Improved collaboration: Developers and operations teams work together efficiently.
Scalability & security: Cloud-based deployment ensures high availability and security.
Security Best Practices in Java Development
Security is a critical aspect of the Full Stack Java Developer Roadmap, as Java applications often handle sensitive data and business logic. Following Security Best Practices in Java Development ensures protection against cyber threats, data breaches, and unauthorized access. To safeguard applications, Java developers must implement secure coding techniques, authentication mechanisms, and encryption methods.
1. Importance of Security in Java Development
Java is widely used for enterprise applications, making it a primary target for security attacks. The Full Stack Java Developer Roadmap emphasizes learning security best practices to:
Prevent SQL Injection, Cross-Site Scripting (XSS), and Cross-Site Request Forgery (CSRF) attacks.
Protect sensitive user data from unauthorized access.
Ensure compliance with security standards like OWASP Top 10 and GDPR.
2. Secure Coding Practices in Java
1. Input Validation & Sanitization
Never trust user inputs; always validate and sanitize them before processing.
Use regular expressions to restrict invalid input.
Avoid using direct SQL queries; use Prepared Statements to prevent SQL Injection.
PreparedStatement stmt = conn.prepareStatement(“SELECT * FROM users WHERE email = ?”);
stmt.setString(1, userEmail);
ResultSet rs = stmt.executeQuery();
2. Use Secure Authentication & Authorization
Implement JWT (JSON Web Tokens) or OAuth 2.0 for secure user authentication.
Use Spring Security to manage authentication and role-based access control (RBAC).
@Bean
public SecurityFilterChain securityFilterChain(HttpSecurity http) throws Exception {
http.authorizeHttpRequests(auth -> auth
.requestMatchers(“/admin/**”).hasRole(“ADMIN”)
.requestMatchers(“/user/**”).hasAnyRole(“USER”, “ADMIN”)
.anyRequest().authenticated()
)
.formLogin(Customizer.withDefaults());
return http.build();
}
3. Data Encryption and Secure Storage
1. Encrypt Sensitive Data
Use AES (Advanced Encryption Standard) or RSA encryption for data protection.
Store passwords securely using BCrypt hashing instead of plain text.
String hashedPassword = new BCryptPasswordEncoder().encode(“mySecurePassword”);
2. Secure Database Connections
Use SSL/TLS encryption for database connections.
Use environment variables for database credentials.
4. Preventing Common Security Threats
1. Protect Against XSS (Cross-Site Scripting)
Encode user-generated content before rendering it on the webpage.
Implement a Content Security Policy (CSP) to control and restrict script execution.
2. Prevent CSRF (Cross-Site Request Forgery) Attacks
Implement CSRF tokens in web forms to prevent unauthorized requests.
Use Spring Security’s built-in CSRF protection.
3. Secure API Endpoints
Implement rate limiting to prevent DDoS attacks.
Use API keys or OAuth tokens for authentication.
5. Security Testing & Monitoring
1. Perform Security Testing
Use OWASP ZAP or Burp Suite to test web application security.
Conduct penetration testing to find vulnerabilities.
2. Enable Logging & Monitoring
Use Log4j or SLF4J for application logging.
Monitor logs using ELK Stack (Elasticsearch, Logstash, Kibana) to detect security breaches.
Testing and Debugging in Java
Testing and debugging are essential aspects of the Full Stack Java Developer Roadmap, ensuring that applications run smoothly, efficiently, and without errors. By incorporating proper testing methodologies and debugging techniques, Java developers can identify and fix issues early in the development cycle, leading to more robust and reliable applications.
1. Importance of Testing in Java Development
Testing plays a crucial role in software development as it:
Helps detect bugs and errors before deployment.
Ensures that the application meets business requirements.
Improves the performance, security, and maintainability of Java applications.
Enhances user experience by preventing unexpected crashes or failures.
In the Full Stack Java Developer Roadmap, mastering different types of testing is vital for building high-quality software.
2. Types of Testing in Java
1. Unit Testing
Tests individual components or methods of a Java application.
Ensures that each unit of code works as expected.
Commonly used frameworks: JUnit and TestNG.
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.*;
class CalculatorTest {
@Test
void testAddition() {
Calculator calc = new Calculator();
assertEquals(10, calc.add(5, 5));
}
}
2. Integration Testing
Verifies the interaction between multiple components or modules.
Ensures data flow between services is correct.
Frameworks: Spring Test, TestContainers.
3. Functional Testing
Tests if the application meets functional requirements.
Frameworks: Selenium (for UI testing), Cucumber (for BDD testing).
4. Performance Testing
Checks the speed, responsiveness, and stability of a Java application.
Frameworks: JMeter, Gatling.
5. Security Testing
Identifies vulnerabilities in the application.
Tools: OWASP ZAP, SonarQube.
3. Debugging Techniques in Java
Debugging is an essential skill in the Full Stack Java Developer Roadmap. It helps developers identify and fix errors in code.
1. Using Debuggers in IDEs
Eclipse & IntelliJ IDEA provide built-in debuggers for step-by-step execution.
Features like breakpoints, watch variables, and step into/step over help trace issues.
2. Logging for Debugging
Using logging frameworks like Log4j and SLF4J helps track errors and application flow.
Example:
import org.apache.logging.log4j.Logger;
import org.apache.logging.log4j.LogManager;
public class DebugExample {
private static final Logger logger = LogManager.getLogger(DebugExample.class);
public static void main(String[] args) {
logger.info(“Application Started”);
try {
int result = 10 / 0;
} catch (Exception e) {
logger.error(“Error: Division by zero”, e);
}
}
}
3. Exception Handling for Debugging
Use try-catch blocks to handle exceptions gracefully.
Example:
try {
int data = 50 / 0;
} catch (ArithmeticException e) {
System.out.println(“Error: ” + e.getMessage());
}
4. Analyzing Stack Traces
Stack traces provide useful information about errors and their sources.
Example:
Exception in thread “main” java.lang.ArithmeticException: / by zero
at DebugExample.main(DebugExample.java:10)
5. Remote Debugging
Debug applications running on remote servers using JPDA (Java Platform Debugger Architecture).
Example of enabling remote debugging:
java -agentlib:jdwp=transport=dt_socket,server=y,suspend=n,address=5005 -jar app.jar
4. Best Practices for Testing and Debugging in Java
Write Test Cases Before Writing Code (Test-Driven Development – TDD).
Use Mocks & Stubs for unit testing using Mockito.
Automate Tests in CI/CD pipelines for continuous integration.
Use Code Coverage Tools like JaCoCo to measure test effectiveness.
Roadmap to Becoming a Full Stack Java Developer
Becoming a Full Stack Java Developer requires mastering both front-end and back-end technologies, along with essential tools for development, testing, and deployment. The Full Stack Java Developer Roadmap provides a structured learning path to help developers acquire the necessary skills to build end-to-end applications.
1. Learn Core Java and OOP Concepts
The foundation of the Full Stack Java Developer Roadmap starts with mastering Core Java concepts such as:
Object-Oriented Programming (OOP): Encapsulation, Inheritance, Polymorphism, Abstraction.
Exception Handling: Try-Catch, Throw, Throws, Finally.
Collections Framework: Lists, Sets, Maps, and Queues.
Multithreading & Concurrency: Thread management and synchronization.
2. Master Front-End Technologies
A Full Stack Java Developer must be proficient in front-end development using:
HTML, CSS, JavaScript: Essential for building web pages.
Bootstrap, Tailwind CSS: Responsive design frameworks.
JavaScript Frameworks: React.js, Angular, or Vue.js for dynamic UI.
3. Back-End Development with Java
Back-end development is a crucial part of the Full Stack Java Developer Roadmap, including:
Java Servlets & JSP: Basics of web applications.
Spring Boot Framework: Dependency injection, REST APIs, and Microservices.
Spring Security: Authentication & Authorization.
Hibernate & JPA: ORM (Object-Relational Mapping) for database interactions.
4. Database Management
A Full Stack Java Developer must understand SQL and NoSQL databases, including:
SQL Databases: MySQL, PostgreSQL, or Oracle.
NoSQL Databases: MongoDB for handling unstructured data.
5. Web Services & APIs
RESTful APIs: Design, develop, and consume RESTful web services.
GraphQL: An alternative to REST APIs.
Postman: API testing tool.
6. DevOps & Deployment
Deploying Java applications efficiently is an essential part of the Full Stack Java Developer Roadmap:
Version Control: Git, GitHub, GitLab.
CI/CD Pipelines: Jenkins, GitHub Actions.
Containerization & Deployment: Docker, Kubernetes, AWS, and Azure.
7. Security Best Practices
Spring Security for Authentication.
Input Validation & SQL Injection Prevention.
Data Encryption & Logging Mechanisms.
Conclusion
Becoming a Full Stack Java Developer requires a strong foundation in Core Java, expertise in front-end and back-end development, proficiency in databases, and knowledge of DevOps tools for deployment. By following the Full Stack Java Developer Roadmap, developers can systematically acquire the skills needed to build complete, scalable, and secure applications. Continuous learning, hands-on projects, and staying updated with industry trends are key to excelling in this role. With dedication and practice, anyone can become a proficient Full Stack Java Developer and unlock exciting career opportunities.
FAQ's
What is a Full Stack Java Developer?
A Full Stack Java Developer is a professional who develops both the front-end (UI/UX) and back-end (server-side logic) of web applications using Java and related technologies. They work with databases, APIs, and deployment tools to build complete web applications.
What are the key skills required to become a Full Stack Java Developer?
Becoming a Full Stack Java Developer requires diverse skills.
Front-End: HTML, CSS, JavaScript, React.js/Angular
Back-End: Java, Spring Boot, Hibernate
Databases: MySQL, PostgreSQL, MongoDB
Tools: Git, Docker, Kubernetes, Jenkins
Testing & Debugging: JUnit, Mockito
Time to become a Full Stack Java Developer varies, typically 6-12 months.
It depends on your learning speed and dedication. Typically, it takes 6 months to 1 year of continuous learning, hands-on practice, and project development to become proficient.
Is Java still relevant for Full Stack Development?
Yes! Java is widely used in enterprise applications due to its scalability, security, and extensive frameworks like Spring Boot, which makes it highly relevant for full stack development.
What front-end technologies should Java developers learn?
A Full Stack Java Developer should learn:
- HTML, CSS, JavaScript (Fundamentals)
- Frameworks like React.js or Angular (for building dynamic UI)
Bootstrap or Tailwind CSS (for responsive design)
What are the most popular back-end frameworks for Java?
The most commonly used back-end Java frameworks include:
- Spring Boot – for building REST APIs and microservices.
- Hibernate – for database interaction using ORM (Object-Relational Mapping).
- Jakarta EE (formerly Java EE) – for enterprise applications.
Which database technologies should a Full Stack Java Developer know?
Know both SQL and NoSQL databases.
- SQL Databases: MySQL, PostgreSQL, Oracle
NoSQL Databases: MongoDB, Firebase
What tools and platforms should a Full Stack Java Developer master?
A Full Stack Java Developer must be proficient in multiple technologies.
- Version Control: Git, GitHub, GitLab
- Deployment: Docker, Kubernetes, AWS, Heroku
CI/CD Pipelines: Jenkins, GitHub Actions
What are the best resources to learn Full Stack Java Development?
- Online Courses: Udemy, Coursera, freeCodeCamp
- Documentation: Oracle Java Docs, Spring Framework Docs
Projects & Practice: GitHub open-source projects, LeetCode for coding challenges
What career opportunities are available for Full Stack Java Developers?
Full Stack Java Developers are in high demand and can work as:
- Full Stack Developer
- Java Back-End Developer
- Software Engineer
- Web Application Developer
- Microservices Developer