Java Full Stack Course Syllabus
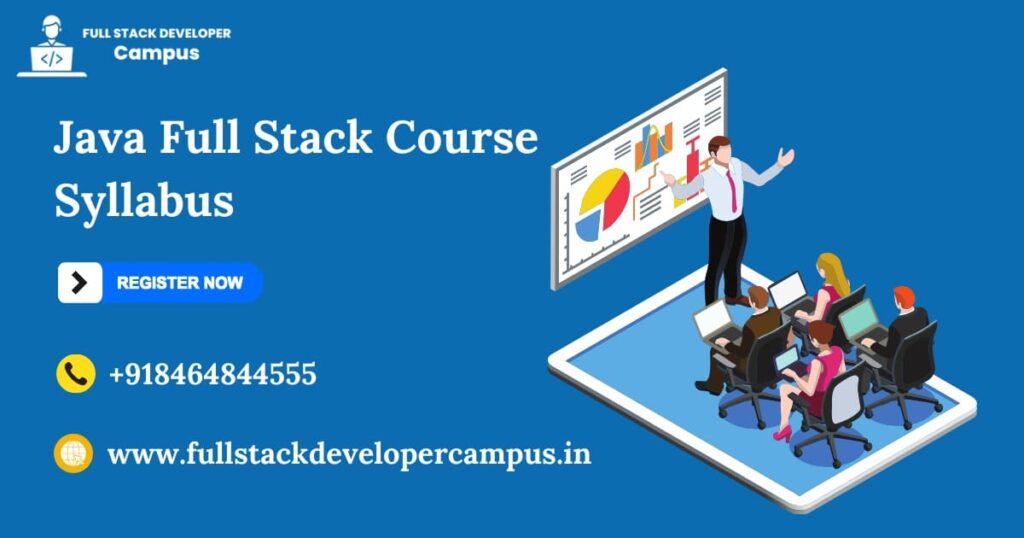
Module 1:Java Full Stack Development
Java Full Stack Course Syllabus
Java Full Stack Course Syllabus covers both front-end and back-end development, making students proficient in building complete web applications. includes technologies like HTML, CSS, JavaScript, React.js for front-end and Java, Spring Boot, Hibernate, and databases for back-end. The Java Full Stack Course Syllabus is structured to help students learn Full Stack Development systematically.
Basics of Full Stack Development
Full Stack Development refers to working on both the client side (front-end) and server side (back-end) of web applications. The Java Full Stack Syllabus includes:
Front-End Development: HTML, CSS, JavaScript, React.js
Back-End Development: Java, Spring Boot, REST APIs
Database Management: SQL, MySQL, MongoDB
Version Control & Deployment: Git, GitHub, Docker, AWS
By following this Java Full Stack Course learners gain expertise in building fully functional applications from scratch.
Importance of Java Full Stack in the IT Industry
Java Full Stack Developers are in high demand because they handle complete application development, from UI design to database management. Companies prefer professionals with Java Full Stack Course expertise because they:
Develop end-to-end applications efficiently
Reduce dependency on multiple developers
Are skilled in modern web frameworks and cloud technologies
Work across multiple domains, from startups to large enterprises
This Java Full Stack ensures that students acquire the skills required to build scalable applications.
Technologies Involved in Java Full Stack Course Syllabus
The Java Full Stack includes a wide range of technologies essential for building robust applications:
Front-End Technologies: HTML, CSS, JavaScript, React.js, Bootstrap
Back-End Technologies: Core Java, Advanced Java, Spring Boot, REST APIs
Database Management: MySQL, MongoDB, Hibernate ORM
DevOps & Deployment: Git, GitHub, Docker, Kubernetes, AWS
Security & Authentication: JWT, OAuth, Spring Security
By mastering these technologies in the Java Full Stack Syllabus, learners become highly skilled Full Stack Developers.
Career Opportunities for Java Full Stack Developers
Java Full Stack Developers have excellent job opportunities in various industries. The Java Full Stack Course prepares students for roles such as:
Java Full Stack Developer
Software Engineer (Full Stack)
Back-End Developer
Front-End Developer
Web Application Developer
With expertise in the Java Full Stack developers can work in IT companies, startups, product-based companies, and freelance projects.
This Java Full Stack ensures that students gain complete knowledge of Full Stack Development and become job-ready professionals.
Module 2: Front-End Development
HTML5, CSS3, and JavaScript
The Java Full Stack Syllabus begins with HTML5, CSS3, and JavaScript, the foundational technologies for web development.
HTML5: Structuring web pages with semantic tags like <header>, <section>, <article>, and <footer>.
CSS3: Styling web pages with properties like Flexbox, Grid, animations, and media queries.
JavaScript: Enabling dynamic behavior using variables, functions, loops, and DOM manipulation.
Mastering these technologies in the Java Full Stack Course ensures a strong front-end foundation.
Bootstrap Framework for Responsive Design
Bootstrap is an essential framework in the Java Full Stack Course used for creating mobile-friendly web applications.
Predefined CSS classes for layout, typography, forms, buttons, and modals.
Responsive grid system for designing web pages that work on all devices.
JavaScript components like carousel, navbar, and modals to enhance UI.
Learning Bootstrap in the Full Stack Syllabus helps developers build attractive and responsive websites efficiently.
Introduction to React.js for UI Development
React.js is a powerful front-end library covered in the Full Stack Course Syllabus for building interactive user interfaces.
Component-Based Architecture: Reusable UI components for modular development.
Virtual DOM: Optimized rendering for better performance.
JSX (JavaScript XML): Writing HTML-like syntax inside JavaScript for easy UI design.
React.js is a key part of the Full Stack Course Syllabus, enabling developers to build modern, high-performance web applications.
Components, Props, and State in React
The Full Stack Course Syllabus covers the fundamentals of React.js, focusing on:
Components: Building UI elements as reusable blocks.
Props (Properties): Passing data between components.
State: Managing dynamic data within a component.
Understanding these concepts in the Full Stack Course Syllabus allows developers to build scalable and maintainable React applications.
Routing and API Integration in React
React Router is an essential part of the Java Full Stack Course, used for navigating between different pages in a React application.
React Router: Implementing single-page application (SPA) navigation.
API Calls with Fetch & Axios: Fetching data from RESTful APIs.
State Management with Redux: Managing global application state efficiently.
By covering Routing and API Integration, the Java Full Stack Syllabus ensures developers can build fully functional web applications.
Java Full Stack Course Concepts Related to Front-End
This module in the Java Full Stack Course equips learners with essential front-end skills, making them proficient in:
Responsive UI Development
Dynamic Web Application Development
Component-Based UI Design with React.js
Integration of APIs for Data Fetching
Module 3: Core Java & Object-Oriented Programming (OOPs)
Java Full Stack Course Syllabus Fundamentals
The Java Full Stack Syllabus begins with Core Java, which is the foundation for back-end development. Core Java includes the basic concepts needed to build strong programming logic. The Java Full Stack Syllabus ensures that students understand:
Java syntax, data structures, and algorithmic thinking.
The role of Java in full stack development.
How Java integrates with databases and frameworks like Spring Boot.
By mastering Core Java in the Java Full Stack Course, learners gain confidence in Java programming.
Java Data Types, Variables, and Operators
The Java Full Stack Course covers the essential building blocks of Java programming:
Data Types: Primitive types (int, float, char, boolean) and non-primitive types (String, Arrays, Objects).
Variables: Declaration, initialization, and scope.
Operators: Arithmetic, relational, logical, bitwise, and assignment operators.
Understanding these concepts in the Full Stack Course Syllabus helps developers write efficient and error-free Java programs.
Control Statements (if, switch, loops)
Control statements are a key part of Java logic and decision-making, covered in the Java Full Stack Course :
Conditional Statements: if, if-else, switch-case.
Looping Statements: for, while, do-while.
Jump Statements: break, continue, return.
These concepts in the Full Stack Course Syllabus enable developers to control program flow effectively.
Object-Oriented Programming (OOPs)
OOP is a crucial part of the Full Stack Course Syllabus, making Java applications scalable and reusable. The key concepts covered include:
Classes & Objects: Blueprint of Java programs.
Encapsulation: Protecting data with private access modifiers.
Inheritance: Reusing code by extending parent classes.
Polymorphism: Method overloading and overriding for flexibility.
Abstraction: Hiding implementation details using abstract classes and interfaces.
OOP principles in the Full Stack Course Syllabus help developers build structured and efficient Java applications.
Exception Handling and Multithreading
The Java Full Stack includes error handling and concurrent programming:
Exception Handling: try-catch, finally, throw, throws, custom exceptions.
Multithreading: Running multiple tasks in parallel using Thread, Runnable, and synchronization.
Learning these advanced topics in the Java Full Stack ensures smooth and efficient Java applications.
Java Full Stack Topics in Core Java
The Java Full Stack Syllabus covers:
Java syntax, variables, and data types.
Control structures and loops.
Object-oriented programming principles.
Exception handling for robust applications.
Multithreading for concurrent execution.
By mastering these Java Full Stack Syllabus topics, developers gain a strong foundation in Java programming, essential for full stack development.
Module 4: Advanced Java
Servlets and JSP (Java Server Pages)
The Java Full Stack Syllabus includes Servlets and JSP, essential for dynamic web application development.
Servlets: Java programs that handle requests and responses in web applications.
Life cycle: init(), service(), destroy().
Request handling using doGet() and doPost().
JSP (Java Server Pages): Used for creating dynamic web pages.
JSP Tags: <jsp:include>, <jsp:forward>.
Scriptlets, Expressions, and Directives.
Mastering Servlets and JSP in the Java Full Stack Course helps developers build server-side applications.
JDBC (Java Database Connectivity)
JDBC is a core part of the Java Full Stack Syllabus for database interaction in Java applications.
JDBC API: Establishing a connection between Java applications and databases.
Key JDBC Classes: DriverManager, Connection, Statement, PreparedStatement, ResultSet.
CRUD Operations: Performing Create, Read, Update, and Delete operations on databases.
Transaction Management: Handling database transactions effectively.
By learning JDBC in the Java Full Stack Course developers can integrate databases into Java applications.
Java Full Stack Syllabus Topics in Enterprise Java
Enterprise Java is an advanced topic covered in the Java Full Stack Course enabling scalable business applications.
Java EE Architecture: Components like Servlets, JSP, EJB, and Web Services.
JPA (Java Persistence API): Managing relational data with ORM.
Microservices & Cloud Integration: Deploying Java applications on cloud platforms.
These topics in the Java Full Stack Syllabus prepare developers for large-scale application development.
Spring Framework Basics
The Java Full Stack Syllabus includes Spring Framework, a powerful tool for building Java applications.
Spring Core: Dependency Injection (DI) and Inversion of Control (IoC).
Spring MVC: Web application development using Model-View-Controller architecture.
Spring Security: Authentication and authorization mechanisms.
Spring Framework is an essential part of the Java Full Stack Syllabus, simplifying Java application development.
Spring Boot for Microservices
Spring Boot is a critical topic in the Java Full Stack Syllabus, allowing rapid development of standalone Java applications.
Spring Boot Features: Auto-configuration, embedded servers, minimal XML configuration.
Microservices Architecture: Creating independent and scalable services.
Spring Boot Actuator: Monitoring and managing applications.
Learning Spring Boot in the Full Stack Course Syllabus helps developers build high-performance applications.
RESTful APIs with Spring Boot
The Full Stack Course Syllabus covers RESTful APIs, crucial for communication between front-end and back-end systems.
Creating REST APIs: Using @RestController, @GetMapping, @PostMapping, @PutMapping, @DeleteMapping.
Consuming APIs: Fetching data from databases and returning JSON responses.
Security in REST APIs: Implementing JWT and OAuth for authentication.
This topic in the Full Stack Syllabus enables developers to build API-driven applications.
Hibernate for ORM (Object-Relational Mapping)
Hibernate is an essential ORM framework included in the Java Full Stack used for database operations.
Hibernate Basics: Replacing JDBC with Hibernate for efficient database interaction.
Annotations in Hibernate: @Entity, @Table, @Id, @Column.
Hibernate Query Language (HQL): Writing queries to interact with the database.
Lazy & Eager Loading: Optimizing database performance.
By learning Hibernate in the Java Full Stack Course developers can manage databases effectively without writing complex SQL queries.
Module 5: Back-End Development
Java Full Stack Syllabus Related to Back-End
The Full Stack Course Syllabus covers essential back-end technologies to handle server-side logic, data processing, and integration. The key areas of back-end development in the Java Full Stack Syllabus include:
Server-side programming with Java, Node.js, and Express.js.
Database connectivity and management with SQL and NoSQL databases.
Building RESTful APIs for front-end and back-end communication.
Authentication & Security using JWT and OAuth.
This Java Full Stack Syllabus ensures developers can handle complete back-end operations efficiently.
Node.js and Express.js Basics
Though Java is the primary focus of the Full Stack Course Syllabus, it also covers Node.js and Express.js, which are widely used in back-end development.
Node.js: A runtime environment for running JavaScript on the server.
Express.js: A lightweight web framework for handling HTTP requests.
Middleware in Express.js: Functions for logging, authentication, and error handling.
By learning Node.js and Express.js, developers in the Java Full Stack Syllabus can build scalable server-side applications.
RESTful API Development
RESTful APIs allow communication between front-end and back-end systems in the Java Full Stack Syllabus.
Creating APIs using Java (Spring Boot) and Node.js (Express.js).
HTTP Methods: GET, POST, PUT, DELETE.
Handling JSON Data for structured responses.
Error Handling: Managing status codes and exceptions.
The Full Stack Course Syllabus ensures developers can create high-performance RESTful APIs for application integration.
Integration with Databases
A crucial part of the Java Full Stack Course is database integration, ensuring data persistence and retrieval.
Relational Databases (SQL): MySQL, PostgreSQL, and Oracle using JDBC and Hibernate.
NoSQL Databases (MongoDB): Storing unstructured data with flexibility.
ORM (Object-Relational Mapping): Using Hibernate to interact with databases efficiently.
The Full Stack Course Syllabus equips developers to manage databases for full stack applications.
Authentication and Authorization (JWT, OAuth)
Security is an essential part of the Java Full Stack Course Syllabus, focusing on user authentication and access control.
JWT (JSON Web Token): Secure token-based authentication.
OAuth 2.0: Third-party authentication like Google, Facebook login.
Spring Security: Protecting Java applications from unauthorized access.
This module in the Java Full Stack Syllabus ensures applications are secure and prevent unauthorized access.
Deployment of Back-End Applications
The Java Full Stack Syllabus covers deployment strategies for hosting back-end applications.
Cloud Platforms: AWS, Google Cloud, and Azure.
Containerization: Using Docker for packaging applications.
CI/CD Pipelines: Automating deployment with Jenkins and GitHub Actions.
By mastering deployment techniques in the Java Full Stack Course developers can launch applications in real-world environments efficiently.
Module 6: Database Management
SQL and NoSQL Databases
The Full Stack Course Syllabus covers both SQL (Structured Query Language) and NoSQL (Non-Relational) databases for efficient data storage and retrieval.
SQL Databases: Structured, relational databases that store data in tables.
NoSQL Databases: Flexible, schema-less databases designed for handling large-scale data.
Understanding SQL and NoSQL in the Java Full Stack Syllabus helps developers choose the right database for their applications.
MySQL, PostgreSQL, MongoDB
The Java Full Stack Syllabus focuses on industry-standard databases:
MySQL: Open-source relational database management system (RDBMS).
PostgreSQL: Advanced, open-source RDBMS with powerful features.
MongoDB: A NoSQL document-based database for handling large amounts of unstructured data.
By covering MySQL, PostgreSQL, and MongoDB, the Java Full Stack Course Syllabus ensures developers can work with different database architectures.
Database Queries and Indexing
The Java Full Stack Syllabus teaches efficient data retrieval using queries and indexing:
SQL Queries: SELECT, INSERT, UPDATE, DELETE.
Joins: Inner, Left, Right, Full Joins for combining data from multiple tables.
Indexing: Optimizing query performance with indexes (B-Tree, Hash Index).
These concepts in the Java Full Stack Syllabus improve database efficiency and speed.
Java Full Stack Syllabus for Database Management
The Java Full Stack Syllabus includes:
Schema Design: Creating well-structured database schemas.
Normalization: Reducing redundancy and improving consistency.
Data Backup & Recovery: Ensuring data safety and integrity.
Mastering database management in the Java Full Stack Syllabus enables developers to handle real-world data operations.
Data Modeling and Relationships
A key part of the Java Full Stack Course Syllabus is designing effective data models:
Entity-Relationship (ER) Models: Defining relationships between data entities.
Primary Key & Foreign Key Constraints: Ensuring referential integrity.
One-to-One, One-to-Many, Many-to-Many Relationships.
By learning data modeling, developers in the Java Full Stack Syllabus can design efficient database structures.
ORM with Hibernate and JPA
The Full Stack Course Syllabus covers Hibernate and JPA (Java Persistence API) for Object-Relational Mapping (ORM):
Hibernate Annotations: @Entity, @Table, @Column, @OneToMany, @ManyToOne.
CRUD Operations: Performing database transactions using Hibernate.
Lazy vs. Eager Loading: Optimizing database queries for performance.
By mastering ORM with Hibernate and JPA, developers in the Java Full Stack Course can efficiently integrate Java applications with databases.
Module 7: DevOps and Deployment
Java Full Stack Course Syllabus Related to DevOps
The Java Full Stack Syllabus covers DevOps concepts to ensure smooth development, testing, and deployment of applications. Key topics include:
Version Control with Git & GitHub for tracking changes in code.
CI/CD (Continuous Integration & Deployment) for automated software delivery.
Containerization using Docker & Kubernetes for scalable applications.
Cloud Computing (AWS, Azure, GCP) for hosting Java Full Stack applications.
Application Hosting & Maintenance for real-world deployment.
By mastering DevOps and Deployment in the Java Full Stack Syllabus, developers can efficiently manage the software development lifecycle.
Git and GitHub for Version Control
Version control is a crucial part of the Java Full Stack Course helping teams collaborate and manage code efficiently.
Git Basics: Initializing repositories, staging changes, committing code.
Branching & Merging: Managing feature development with Git branches.
GitHub Repositories: Storing and sharing code online.
Pull Requests & Code Reviews: Collaborating in development teams.
Learning Git & GitHub in the Java Full Stack Course Syllabus ensures developers follow industry-standard code management practices.
CI/CD (Continuous Integration and Continuous Deployment)
The Java Full Stack Syllabus covers CI/CD pipelines to automate the software delivery process:
Continuous Integration (CI): Automatically testing and integrating code changes.
Continuous Deployment (CD): Automating software releases.
Tools Used: Jenkins, GitHub Actions, GitLab CI/CD, Travis CI.
Pipeline Setup: Running tests, building, and deploying applications.
With CI/CD knowledge, developers in the Java Full Stack Course can ensure smooth and reliable deployments.
Docker and Kubernetes Basics
The Java Full Stack Course introduces containerization for deploying applications in isolated environments:
Docker Basics: Creating and managing containers.
Docker Images & Containers: Packaging Java applications for deployment.
Kubernetes Basics: Orchestrating multiple containers efficiently.
Scaling Applications: Managing workloads with Kubernetes clusters.
By learning Docker & Kubernetes, developers in the Full Stack Course Syllabus can deploy scalable applications in modern environments.
Cloud Platforms (AWS, Azure, GCP)
Cloud computing is an essential part of the Java Stack Course Syllabus, covering popular platforms:
Amazon Web Services (AWS): EC2, S3, RDS, Lambda.
Microsoft Azure: Virtual Machines (VMs), Azure App Services.
Google Cloud Platform (GCP): Compute Engine, Firebase Hosting.
The Full Stack Course Syllabus helps developers deploy applications on cloud platforms, making them industry-ready.
Application Hosting and Maintenance
The Full Stack Course Syllabus includes hosting techniques to make applications live:
Web Server Setup: Apache Tomcat, Nginx.
Domain & SSL Configuration: Securing websites with HTTPS.
Monitoring & Logging: Using Prometheus, Grafana, ELK Stack.
Performance Optimization: Load balancing, caching strategies.
By covering application hosting & maintenance, the Full Stack Course Syllabus ensures developers can manage live applications efficiently.
Module 8: Project Development & Best Practices
Java Full Stack Syllabus for Project Development
The Java Full Stack Syllabus ensures hands-on experience by guiding students through real-world project development. This module covers:
End-to-End Full Stack Development using Java, Spring Boot, React, and databases.
Best Coding Practices for maintainable and scalable applications.
Debugging, Testing, and Optimization to improve performance.
Agile Methodology & Software Development Lifecycle (SDLC) for effective team collaboration.
Final Project Deployment on cloud platforms or web servers.
By completing this module, learners in the Java Full Stack Syllabus will be industry-ready full-stack developers.
Building a Real-World Project Using Java Full Stack
The Full Stack Course Syllabus includes a capstone project covering:
Front-End Development: Using React.js, HTML5, CSS3, JavaScript, and Bootstrap for a responsive UI.
Back-End Development: Using Java, Spring Boot, and RESTful APIs for server-side logic.
Database Integration: Using MySQL, PostgreSQL, or MongoDB for data storage.
User Authentication: Implementing JWT or OAuth for security.
Hosting & Deployment: Deploying on AWS, Azure, or Firebase for public access.
This Java Full Stack Syllabus project provides hands-on experience in real-world development.
Code Optimization and Best Practices
The Java Full Stack Course Syllabus covers industry-standard coding best practices to ensure efficiency and maintainability:
Code Modularity & Reusability: Using functions, components, and microservices.
Performance Optimization: Lazy loading, caching, and database indexing.
Code Formatting & Documentation: Using tools like Prettier, ESLint, and Javadoc.
Security Best Practices: Preventing SQL Injection, XSS, and CSRF attacks.
By following these best practices, developers in the Java Full Stack Course Syllabus can write clean, optimized, and secure code.
Debugging and Testing Strategies
Testing is a crucial part of the Java Full Stack Course Syllabus, ensuring application reliability. Key topics include:
Debugging Techniques: Using IDE Debuggers, Loggers, and Chrome DevTools.
Unit Testing: Writing JUnit and Mockito test cases for Java code.
Integration Testing: Ensuring APIs and services work together.
End-to-End Testing: Using Selenium, Cypress, or Jest for UI testing.
With proper debugging and testing in the Java Full Stack Course Syllabus, developers can build error-free applications.
Agile Methodology and Software Development Lifecycle (SDLC)
The Java Full Stack Course Syllabus introduces Agile & SDLC for structured development processes:
Agile Development: Working in sprints using Scrum or Kanban.
Version Control: Managing code with Git & GitHub.
Requirement Analysis & Planning: Understanding client needs.
Development & Deployment: Building and testing applications iteratively.
By learning Agile methodology, developers in the Java Full Stack Course Syllabus can work effectively in professional environments.
Final Project Submission and Deployment
The last stage of the Java Full Stack Course Syllabus is deploying a fully functional project:
Hosting Options: Deploying on AWS EC2, Azure, Heroku, Firebase, or Netlify.
Domain & SSL Setup: Making applications secure and publicly accessible.
Post-Deployment Monitoring: Using Google Analytics, Log Monitoring, and Performance Testing.
With successful project deployment, students in the Java Full Stack Course Syllabus will have a strong portfolio to showcase their skills.
Conclusion:
The Java Full Stack Course Syllabus is a comprehensive learning path designed to equip developers with in-depth knowledge of front-end, back-end, database management, DevOps, and deployment. This course covers essential technologies, including Java, Spring Boot, React.js, Node.js, MySQL, MongoDB, Docker, Kubernetes, and cloud platforms like AWS, Azure, and GCP.
It ensures a hands-on learning experience by integrating real-world projects, best coding practices, debugging techniques, and Agile methodology. By the end of the course, learners will have developed a fully functional Java Full Stack application, making them industry-ready. The syllabus also emphasizes CI/CD pipelines, version control with Git, security best practices, and performance optimization, preparing developers to work in modern software environments.
With career opportunities in roles such as Java Full Stack Developer, Back-End Developer, Software Engineer, and DevOps Engineer, this course provides the essential skills to build scalable, secure, and high-performing applications.
FAQs
1. What is covered in the Java Full Stack Course Syllabus?
The Java Full Stack Course Syllabus includes front-end development (HTML, CSS, JavaScript, React.js), back-end development (Java, Spring Boot, Node.js, REST APIs), database management (MySQL, MongoDB), DevOps tools (Git, Docker, Kubernetes, CI/CD), and cloud deployment (AWS, Azure, GCP). It also includes hands-on project development and best coding practices.
2. Who should learn the Java Full Stack Course?
This course is ideal for beginners, students, software engineers, and professionals who want to become full-stack developers. A basic understanding of programming concepts can be helpful, but not mandatory.
3. What tools and technologies will I use in this course?
The Java Full Stack Course Syllabus covers a wide range of tools and technologies, including:
- Frontend: HTML, CSS, JavaScript, React.js, Bootstrap
- Backend: Java, Spring Boot, Node.js, Express.js
- Database: MySQL, PostgreSQL, MongoDB
- Version Control: Git, GitHub
- DevOps & Deployment: Docker, Kubernetes, AWS, CI/CD Pipelines
4. How long does it take to complete the Java Full Stack Course?
The duration varies based on learning pace, but typically, it takes 3 to 6 months to complete the syllabus, including hands-on practice and project development.
5. What projects will be covered in this course?
The Java Full Stack Course Syllabus includes real-world projects such as:
- E-commerce Website (Product listing, cart, and payment integration)
- Social Media App (User authentication, profile management, and posting)
- Blogging Platform (Content management with a database)
- Employee Management System (CRUD operations, authentication, and API integration)
6. What career opportunities are available after completing this course?
After completing the Java Full Stack Course Syllabus, you can apply for roles such as:
- Java Full Stack Developer
- Back-End Developer
- Front-End Developer
- Software Engineer
- DevOps Engineer
- Cloud Developer
7. Will I learn cloud deployment in this course?
The Java Full Stack Course Syllabus includes cloud deployment topics such as AWS EC2, Azure App Services, Firebase Hosting, and Kubernetes to help you deploy real-world applications.
8. Does the course include hands-on projects?
the syllabus emphasizes practical learning through multiple projects and a final capstone project, ensuring hands-on experience with Java Full Stack development.
9. Will I learn DevOps tools in this course?
Yes, DevOps is a key part of the Java Full Stack Course Syllabus. You will learn Git, GitHub, CI/CD (Jenkins, GitHub Actions), Docker, Kubernetes, and cloud deployment strategies.
10. How will this course help in job placement?
By completing the Java Full Stack Course Syllabus, you will gain in-demand skills, build portfolio projects, and learn industry best practices, which will enhance your resume and job opportunities.
11. Is this course suitable for beginners?
the Java Full Stack Course Syllabus starts from the basics and gradually moves to advanced topics. Even beginners with little or no programming knowledge can follow along, as each module includes step-by-step explanations.
12. What is the role of Java in full stack development?
Java plays a crucial role in backend development for full stack applications. It is widely used with Spring Boot to build scalable REST APIs, manage databases, and implement business logic. The Java Full Stack Course Syllabus ensures you master Java’s role in a full stack environment.
13. Does this course cover Java frameworks like Spring Boot?
Yes, the Java Full Stack Course Syllabus includes Spring Boot, which is essential for modern backend development. It covers dependency injection, microservices, REST APIs, security, and database integration using Hibernate.
14. What kind of database technologies will I learn?
The Java Full Stack Course Syllabus covers both SQL (MySQL, PostgreSQL) and NoSQL (MongoDB) databases. You will learn how to design databases, write optimized queries, and integrate them with Java applications.
15. Will this course cover API development and integration?
The Java Full Stack Course Syllabus includes RESTful API development using Spring Boot and Node.js. You will learn how to create APIs, connect them with front-end applications, and integrate third-party APIs.