Java Full Stack Interview Questions
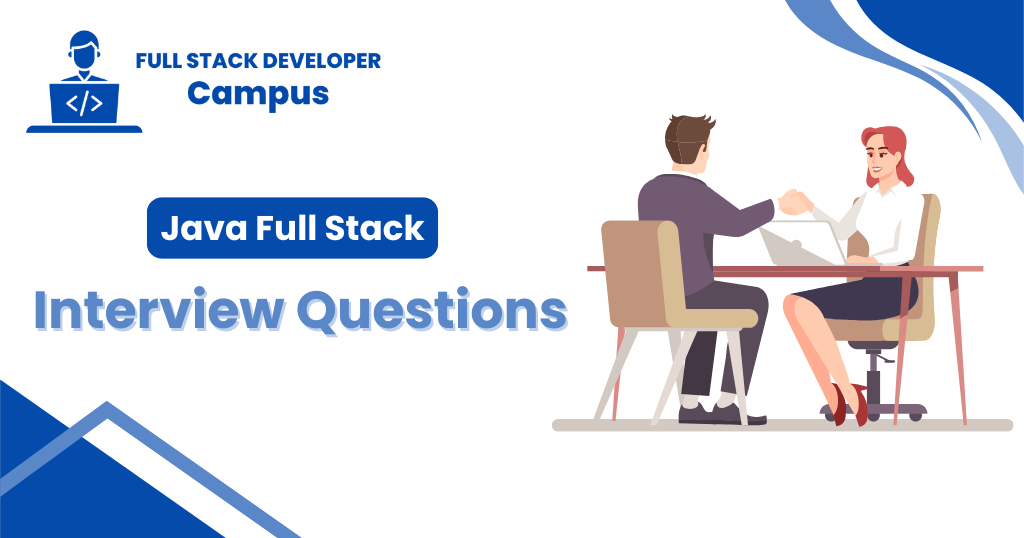
1. What is Java?
Java is a high-level, Object-Oriented, Platform Independent programming Language. It is widely used for building web applications, mobile apps, desktop applications, and enterprise applications.
2. What are JDK, JRE, and JVM in Java.
JDK – Java Development Kit
It’s a toolkit for developing Java applications.
It includes tools like compilers and debuggers to write, compile, and run Java programs.
Contains the JRE (Java Runtime Environment) and development tools.
JRE – Java Runtime Environment
It’s a part of the JDK.
Provides the libraries, Java Virtual Machine (JVM), and other components to run applications written in Java.
It doesn’t include development tools like compilers or debuggers.
JVM – Java Virtual Machine.
It’s the heart of the Java programming language.
Executes Java bytecode, making Java platform-independent.
Converts bytecode into machine code for the host system.
3. Tell me the main features of Java?
Java has several features which makes it stand ahead with other programming languages. They are:
Platform Independence: Any device with compatible JVM can run java code, without needing to be recompiled.
Object-Oriented: Java uses an object-oriented programming model, making it modular, flexible, and easy to maintain.
Robust: Java highlights early error checking, runtime checking, and has strong memory management capabilities, reducing the likelihood of errors and crashes.
Security: Java provides a secure platform with built-in security features like bytecode verification, and a runtime environment that can run untrusted code within a sandbox.
Multi-threaded: Java supports multi-threading, allowing multiple threads to run concurrently, improving the performance of applications.
High Performance: Through the use of Just-In-Time (JIT) compilers, Java achieves high performance by converting bytecode to native machine code at runtime.
Distributed: Java has strong networking capabilities, making it easy to develop distributed applications.
Dynamic: Java can adapt to an evolving environment, supporting dynamic linking and runtime changes.
4. Differentiate between Java and JavaScript?
Java:
Java is a Compiled language.
It is often used for building standalone applications, enterprise-level backend systems, and Android apps.
It runs on the Java Virtual Machine (JVM), which allows it to be platform-independent.
It is object-oriented and has strong syntax
It is generally faster due to being compiled to bytecode.
JavaScript:
It is an Interpreted language.
It is primarily used for adding interactive elements to web pages, but also for server-side development with environments like Node.js.
It runs in web browsers and on servers without the need for compilation.
It is prototype-based and dynamic
It is generally slower due to being interpreted, but modern engines and just-in-time compilation have significantly improved its speed.
5. Explain about an object-oriented programming language?
An object-oriented programming (OOP) language is designed around the concept of “objects”. In OOP, everything is organized into classes and objects, which makes it easier to design, manage, and scale complex applications. Here are the core concepts of OOP in Java :
Class: A blueprint for creating objects. It defines a set of properties and methods that the created objects will have.
Object: An instance of a class. It represents a real-world entity with a state (attributes) and behavior (methods).
Encapsulation: Bundling the data (attributes) and methods (functions) that operate on the data into a single unit, or class. This hides the internal state of the object from the outside world.
Inheritance: A way to form new classes using classes that have already been defined. It helps in reusing code and establishing a subtype from an existing object.
Polymorphism: The ability of different objects to respond to the same message (or method call) in different ways, typically achieved through method overriding.
Abstraction: The concept of showing what a program does rather than how a program does . Here we hide complex implementation details and show only the necessary details. This helps in reducing programming complexity and effort.
6. What are the four pillars in object-oriented programming systems through Java?
The four pillars OOPS in java are:
Encapsulation
Inheritance
Polymorphism
Abstraction
7. Explain about class in Java?
A Class is a blueprint of an object. Objects are created here. It defines a set of properties and methods that the created objects will have. It is also defined as a collection of objects.
8. Explain about an object in Java?
An object is defined as an instance of a class. It represents a real-world entity with a state (attributes) and behavior (methods).
9. Explain, how do you create an object in Java?
Creating the object involve following steps:
Define a Class: Write a class that outlines the object’s structure and behavior.
public class Dog {
String name;
int age;
void bark() {
System.out.println(“Woof!”);
}
}
Instantiate the Object: Use the new keyword.
Dog myDog = new Dog();
Initialize the Object: Set its properties and call its methods.
myDog.name = “Buddy”;
myDog.age = 5;
myDog.bark(); // Outputs: Woof!
10. What is inheritance in Java? Explain types of inheritance.
Inheritance is a process of creating new classes using classes that have already been defined. It helps in reusing code and establishing a subtype from an existing object.
Types of inheritance:
In Java, there are five main types of inheritance:
Single Inheritance: A child class inherits from one parent class.
Multiple Inheritance (via Interfaces): A class can implement multiple parent classes. But classes do not perform multilevel inheritance. But Interfaces can.
Multilevel Inheritance: A child class inherits from a parent class which in turn inherits from another parent class.
Hierarchical Inheritance: Multiple classes inherit from a single parent class.
Hybrid Inheritance: A combination of two or more types of inheritance. Hybrid inheritance is not possible with a combination of multiple inheritance by classes.
Enhance Your Coding Career with Full Stack Development
11. Why is Multiple Inheritance not possible in Classes?
Multiple inheritance in classes is not allowed in Java to avoid ambiguity (confusion) when two parent classes have the same method. This can cause the “diamond problem,” where Java doesn’t know which method to use. Java solves this by allowing multiple inheritance through interfaces, which are simpler and don’t create this issue.
12. Tell me about the purpose of super keyword in Java?
The super keyword in Java is used to call methods and constructors of the parent class. It helps you reuse and extend the functionalities of a parent class in a child class.
13. What is polymorphism in Java?
Polymorphism allows methods or objects to behave differently based on the object they are acting upon. In Java, it’s achieved through method overloading (compile-time polymorphism) and method overriding (runtime polymorphism).
14. Why Interfaces perform multiple inheritance?
Interfaces allow multiple inheritance because a class can implement multiple interfaces. Interfaces only provide method declarations, without actual implementation.
15. What is encapsulation in Java?
Encapsulation is the concept of bundling data (fields) and methods that operate on the data into a single unit (class) and restricting direct access to some of the object’s components using access modifiers.
16. Why do we use access modifiers in Java?
Access modifiers control the visibility and accessibility of classes, methods, and variables, ensuring encapsulation, data protection, and controlled access within the program.
17. Explain the difference between public, private, protected, and default access modifiers in Java.
public: Accessible everywhere throughout the project.
private: Accessible only within the same class. And accessible when inherited
protected: Accessible within the same package and subclasses.
default (no keyword): Accessible within the same package only.
18. What is abstraction in Java?
The concept of showing what a program does rather than how a program does . Here we hide complex implementation details and show only the necessary details. This helps in reducing programming complexity and effort.
19. How do you achieve abstraction in Java?
Abstraction is achieved using abstract classes and interfaces. Abstract classes can have both abstract and concrete methods, while interfaces provide method declarations that must be implemented by the class.
20. What is the use constructor in Java?
A constructor in Java is a special method used to initialize objects. It is called when an object is created in a class. The main purposes of constructors are:
Initialize Object State: Set initial values for the object’s attributes.
Allocate Resources: Set up any resources the object might need during its lifetime.
Ensure Proper Object Creation: Enforce constraints and ensure the object is in a valid state when it’s created.
Java Full Stack Interview Questions For Freshers
21. Explain the difference between default and parameterized constructors in Java.
Default Constructor:
- A constructor with no parameters.
- It is automatically provided by Java if no other constructors are defined.
- It initializes an object with default values.
Example:
public class Car {
// Default constructor
public Car() {
System.out.println(“Default constructor called”);
}
}
Parameterized Constructor:
- A constructor that takes one or more parameters.
- It is defined by the programmer to initialize an object with specific values.
- It allows setting initial values for object attributes.
Example:
public class Car {
String model;
int year;
// Parameterized constructor
public Car(String model, int year) {
this.model = model;
this.year = year;
}
22. Tell me about the purpose of the static keyword in Java?
The static keyword is used for methods or variables that belong to the class, not to any specific instance. Static members can be accessed without creating an instance of the class.
23. What is a static method in Java?
A static method in Java belongs to the class rather than instances of the class. This means you can call a static method without creating an object of the class. Static methods can access static variables and other static methods directly, but they cannot access instance variables or instance methods. You call a static method using the class name, like this:
class MathUtils {
public static int add(int a, int b) {
return a + b;
}
}
// Calling a static method
int sum = MathUtils.add(5, 3);
24. Differentiate between final, finally, and finalize in Java.
1. final:
The final keyword can be applied to variables, methods, or classes to indicate that they cannot be modified.
- final variable: Once assigned a value, it cannot be changed (constant).
- final method: Cannot be overridden by child classes .
- final class: Cannot be subclassed (no child classes can be created from it).
2. finally:
The finally block is used in exception handling. It contains code that will run no matter what, whether an exception is handled or not. This is often used for resource cleanup (e.g., closing a file or releasing a database connection).
3. finalize():
The finalize() method is part of Java’s garbage collection mechanism. It is called by the garbage collector before an object is destroyed. This method allows the object to perform any cleanup actions, like closing open resources. However, it is rarely used in modern programming as the garbage collector handles most memory management.
25. What is method overriding in Java?
Method overriding occurs when a subclass provides a specific implementation of a method that is already defined in its superclass, allowing runtime polymorphism.
26. What is method overloading in Java?
Method overloading is defining multiple methods with the same name but different parameter types or numbers within the same class, allowing compile-time polymorphism.
27. What is a package in Java?
A package is a namespace that organizes related classes and interfaces, preventing naming conflicts and improving code maintainability.
28. How do you import packages in Java?
You import packages using the import statement. For example: import java.util.Scanner;.
29. What is the purpose of the import statement in Java?
The import statement allows you to use classes and interfaces from other packages without needing to specify their full package name every time.
30. What is a Java interface?
An interface in Java is a reference type that contains abstract methods (method signatures without implementation) that must be implemented by a class.
Transform Your Future with Full Stack Development Skills
31. Explain the difference between abstract classes and interfaces in Java.
Abstract Class: Can have both abstract and concrete methods, constructors, and state (fields).
Interface: Can only declare abstract methods (Java 8+ allows default and static methods) and does not contain constructors or state.
32. Can you implement multiple interfaces in Java?
Yes, a class can implement multiple interfaces, allowing for multiple inheritance of method signatures.
33. What is a Java abstract class?
A Java abstract class is a class that cannot be instantiated (you cannot create objects of it). It is meant to be extended by other classes that will provide specific implementations for its abstract methods. An abstract class can contain both abstract methods (methods without a body) and concrete methods (methods with a body).
34. What is the purpose of the instanceof operator in Java?
The instanceof operator is used to check if an object is an instance of a specific class or interface. It returns true if the object is an instance of the specified type, and false otherwise. This is useful for type-checking before casting objects.
35. What are the different types of variables in Java?
Local Variables: Declared within a method or a block and accessible only within that scope.
Instance Variables: Declared inside a class but outside any method. They are unique to each instance of the class.
Class Variables (Static Variables): Declared with the static keyword inside a class and outside of any method. They are shared in the class among all instances.
36. What is the difference between local variables, instance variables, and class variables in Java?
Local Variables:
Scope: Within the method or block.
Lifetime: Exists during the execution of the method/block.
Default Value: Must be initialized before use.
Instance Variables:
Scope: Throughout the class.
Lifetime: Exists as long as the object exists.
Default Value: Initialized to default values (e.g., 0 for int, null for objects).
Class Variables:
Scope: Throughout the class and shared among all instances.
Lifetime: Exists as long as the class is loaded in memory.
Default Value: Initialized to default values.
37. How do you declare and initialize variables in Java?
Declaration: Specify the data type followed by the variable name.
int num;
Initialization: Assign a value to the variable.
num = 10;
Declaration and initialization together:
int num = 10;
38. What is the purpose of the volatile keyword in Java?
The volatile keyword ensures that a variable’s value is always read from and written to the main memory, making it visible to all threads. It is used in multithreading to avoid issues with caching.
39. What is the purpose of the transient keyword in Java?
The transient keyword prevents certain variables from being serialized when an object is converted to a byte stream. It is used when some fields of a class should not be saved.
40. What is a Java array?
An array in Java is a fixed-size collection of elements of the same type, stored in contiguous memory locations.
41. How do you declare and initialize arrays in Java?
Declaration:
int[] arr;
Initialization:
arr = new int[5]; // array of size 5
Declaration and initialization together:
int[] arr = {1, 2, 3, 4, 5};
42. What is the length of an array in Java?
The length of an array is the number of elements it can hold. It can be accessed using arrayName.length.
43. What is the difference between arrays and ArrayList in Java?
Array: Fixed in size, can hold elements of a single data type.
ArrayList: Dynamic in size, can grow or shrink, and can only hold objects (can’t hold primitives directly, but uses wrapper classes).
44. What is the purpose of the clone() method in Java?
The clone() method creates and returns a copy (clone) of the object. It performs a shallow copy unless explicitly overridden.
45. What is the purpose of the toString() method in Java?
The toString() method returns a string representation of the object. It is commonly overridden to provide meaningful output for an object.
46. What is the purpose of the equals() method in Java?
The equals() method checks if two objects are meaningfully equal (i.e., have the same content or state) rather than simply comparing their memory locations.
47. What is the purpose of the hashCode() method in Java?
The hashCode() method returns an integer (hash code) representing the object. It’s used for efficient storage and retrieval in collections like HashMap and HashSet.
48. What is the purpose of the getClass() method in Java?
The getClass() method returns the runtime class of an object, giving information about the object’s class.
49. What is exception handling in Java?
Exception handling in Java is a mechanism to handle runtime errors or exceptions in a controlled way using try, catch, finally, and throw blocks to prevent program crashes.
50. Speak about the try, catch, and finally blocks in Java.
Try Block: Contains the code that might throw an exception. Catch Block: Catches and handles the exception. Finally Block: Executes code, regardless of whether an exception was thrown. It’s often used for cleanup.
throw:
The throw keyword is used to explicitly throw an exception from a method or block of code. This is useful when you want to signal an error condition.
throws:
The throws keyword is used in a method declaration to indicate that the method might throw an exception. It informs the caller that they need to handle or declare the exception.
51. Differentiate between checked and unchecked exceptions in Java?
Checked Exceptions: Exceptions that are checked at compile-time. Methods must either handle these exceptions or declare them using the throws keyword.
Example: IOException, SQLException
Unchecked Exceptions: Exceptions that are checked at runtime. These include errors and runtime exceptions that don’t need explicit handling.
Example: NullPointerException, ArrayIndexOutOfBoundsException
52. What is a Java String?
A Java String is an object that represents a sequence of characters. Strings are immutable, meaning once created, their values cannot be changed.
53. How do you create and manipulate strings in Java?
Creating Strings:
String str = “Hello, World!”;
Manipulating Strings:
String upper = str.toUpperCase(); // “HELLO, WORLD!”
String lower = str.toLowerCase(); // “hello, world!”
int length = str.length();
54. What is the difference between String, StringBuilder, and StringBuffer in Java?
String: Immutable sequence of characters.
String Builder: Mutable sequence of characters, not thread-safe, faster for single-threaded operations.
String Buffer: Mutable sequence of characters, thread-safe, slightly slower due to synchronization.
55. What is a Java ArrayList?
An ArrayList is a resizable array implementation of the List interface in Java. It allows for dynamic arrays that can grow and shrink as needed.
56. How do you create and manipulate ArrayLists in Java?
Creation:
ArrayList<String> list = new ArrayList<>();
Manipulation:
Add elements:
list.add(“Apple”);
Access elements:
String fruit = list.get(0); // Access first element
Remove elements:
list.remove(“Apple”); // Remove by value
57. What is the purpose of the Iterator interface in Java?
The Iterator interface provides a way to traverse (iterate) through the elements of a collection (like ArrayList, HashSet, etc.) without exposing the underlying structure. It allows for safe removal of elements during iteration.
58. What is a Java HashMap?
A HashMap is a part of the Java Collections Framework that implements the Map interface. It stores key-value pairs, allowing for efficient retrieval of values based on keys.
59. How do you create and manipulate HashMaps in Java?
Creation:
HashMap<String, Integer> map = new HashMap<>();
Manipulation:
Put key-value pairs:
map.put(“Apple”, 1);
Get values by key:
int value = map.get(“Apple”); // Returns 1
Remove key-value pairs:
map.remove(“Apple”);
60. What is the purpose of the entrySet() method in Java HashMap?
The entrySet() method returns a set view of the mappings contained in the HashMap. It allows you to iterate through the key-value pairs in the map.
61. What is the purpose of the put() and get() methods in Java HashMap?
put(): Adds a key-value pair to the map or updates the value if the key already exists.
get(): Retrieves the value associated with a specified key.
62. What is the purpose of the remove() method in Java HashMap?
The remove() method removes the key-value pair associated with the specified key from the map.
63. What is a Java HashSet?
A HashSet is a collection that implements the Set interface. It stores unique elements and does not allow duplicates.
64. How do you create and manipulate HashSets in Java?
Creation:
HashSet<String> set = new HashSet<>();
Manipulation:
Add elements:
set.add(“Apple”);
Remove elements:
set.remove(“Apple”);
65. What is the purpose of the add() method in Java HashSet?
The add() method adds a specified element to the HashSet if it is not already present. If the element already exists, it does not change the set.
66. What is a Java LinkedList?
A LinkedList is a collection that implements the List and Deque interfaces. It consists of nodes where each node contains data and a reference to the next node, allowing for efficient insertions and deletions.
67. How do you create and manipulate LinkedLists in Java?
Creation:
LinkedList<String> list = new LinkedList<>();
Manipulation:
Add elements:
list.add(“Apple”);
list.addFirst(“Banana”); // Add at the beginning
Remove elements:
list.remove(“Apple”);
68. What is a Java TreeSet?
A TreeSet is a collection that implements the Set interface and stores elements in a sorted (natural order or according to a specified comparator) manner.
69. How do you create and manipulate TreeSets in Java?
Creation:
TreeSet<String> set = new TreeSet<>();
Manipulation:
Add elements:
set.add(“Apple”);
Remove elements:
set.remove(“Apple”);
70. What is the purpose of the contains() method in Java collections?
Purpose: Checks if a specified element is present in the collection.
Usage: Returns true if the collection contains the element; otherwise, returns false.
List<String> list = new ArrayList<>();
list.add(“apple”);
boolean hasApple = list.contains(“apple”); // true
71. What is the purpose of the isEmpty() method in Java collections?
Purpose: Checks if the collection is empty.
Usage: Returns true if the collection has no elements; otherwise, returns false.
List<String> list = new ArrayList<>();
boolean isListEmpty = list.isEmpty(); // true
72. What is the purpose of the size() method in Java collections?
Purpose: Returns the number of elements in the collection.
Usage: Provides the count of elements.
List<String> list = new ArrayList<>();
list.add(“apple”);
int size = list.size(); // 1
73. What is the purpose of the clear() method in Java collections?
Purpose: Removes all elements from the collection.
Usage: Empties the collection, making it like new.
List<String> list = new ArrayList<>();
list.add(“apple”);
list.clear();
boolean isListEmpty = list.isEmpty(); // true
74. What is the purpose of the toArray() method in Java collections?
Purpose: Converts the collection to an array.
Usage: Useful for obtaining a fixed-size array containing all elements.
List<String> list = new ArrayList<>();
list.add(“apple”);
Object[] array = list.toArray();
75. What is the purpose of the iterator() method in Java collections?
Purpose: Provides an iterator to traverse the elements in the collection.
Usage: Allows sequential access to elements.
List<String> list = new ArrayList<>();
list.add(“apple”);
Iterator<String> iterator = list.iterator();
while (iterator.hasNext()) {
System.out.println(iterator.next());
}
76. What is a Java Comparator?
A Comparator in Java is an interface used to define custom ordering for objects. It provides a compare method that you can implement to specify the sort logic.
77. How do you implement custom sorting using a Comparator in Java?
You implement a Comparator by overriding its compare method, then pass it to a sorting function.
class AgeComparator implements Comparator<Person> {
public int compare(Person p1, Person p2) {
return p1.getAge() – p2.getAge();
}
}
Use it with:
Collections.sort(personList, new AgeComparator
78. What is the purpose of the compareTo() method in Java?
The compareTo method is part of the Comparable interface. It defines the natural ordering of objects, and is used by sorting methods to compare objects.
79. What is a Java thread?
A Java thread is a lightweight process that allows concurrent execution of code. Threads are used to perform multiple tasks simultaneously within a program.
80. How do you create and start a thread in Java?
You can create a thread by:
- Extending the Thread class
To start a thread, you call its start method.
class MyThread extends Thread {
public void run() {
System.out.println(“Thread is running”);
}
}
MyThread t = new MyThread();
- Implementing the Runnable interface
class MyRunnable implements Runnable {
public void run() {
System.out.println(“Thread is running”);
}
}
MyRunnable myRunnable = new MyRunnable();
Thread thread = new Thread(myRunnable);
thread.start();
81. What is the difference between a thread and a process in Java?
Thread: A lightweight unit of execution within a process. Shares the process’s memory and resources.
Process: An independent program with its own memory space. Heavier than threads and takes longer to switch between.
82. How do you implement multithreading in Java?
We Implement multithreading by creating multiple threads and running them concurrently. You can extend Thread or implement Runnable.
83. What is synchronization in Java?
Synchronization is a mechanism to control the access of multiple threads to shared resources. It prevents thread interference and consistency problems.
84. How do you achieve synchronization in Java?
You achieve synchronization using the synchronized keyword, which locks an object or a method to allow only one thread to execute at a time.
85. What is the purpose of the synchronized keyword in Java?
The synchronized keyword ensures that only one thread can access a resource at a time, maintaining data consistency and preventing race conditions.
86. What are the states of a thread in Java?
- New: Thread is created but not yet started.
- Runnable: Thread is ready to run and waiting for CPU time.
- Blocked: Thread is blocked, waiting for a monitor lock.
- Waiting: Thread is waiting indefinitely for another thread.
- Timed Waiting: Thread is waiting for a specified time.
- Terminated: Thread has finished execution.
87. What is deadlock in Java?
Deadlock occurs when two or more threads are blocked forever, waiting for each other to release resources.
88. How do you prevent deadlock in Java?
Prevent deadlock by:
- Avoiding nested locks.
- Using a timeout for lock attempts.
- Following a consistent locking order.
89. What is the purpose of the wait(), notify(), and notifyAll() methods in Java threading?
- wait(): Makes the current thread wait until another thread invokes notify() or notifyAll() on the same object.
- notify(): Wakes up a single thread waiting on the object’s monitor.
- notifyAll(): Wakes up all threads waiting on the object’s monitor.
90. What is Java Full Stack Development?
Java full stack development involves working on both the front-end (client-side) and back-end (server-side) parts of a web application. This includes using Java for server-side development and front-end technologies like HTML, CSS, and JavaScript for the user interface.
91. Explain the difference between front-end and back-end development.
Front-end Development: Focuses on what users see and interact with, such as the user interface (UI) and user experience (UX). Technologies include HTML, CSS, JavaScript, and frameworks like Angular or React.
Back-end Development: Deals with the server-side logic and database management. Technologies include Java, Python, Ruby, and frameworks like Spring Boot or Django.
92. What do you need to build a typical web application?
To build a typical web application, you need:
Front-end: HTML, CSS, JavaScript, and a front-end framework (e.g., React, Angular).
Back-end: A server-side language (e.g., Java, Python), a framework (e.g., Spring Boot, Django), and a database (e.g., MySQL, MongoDB).
93. What’s a servlet, and why is it used in Java web development?
A servlet is a Java program that runs on a server and handles client requests. It is used to create dynamic web content and manage server-side logic.
94. What is the Model-View-Controller (MVC) pattern?
The MVC pattern is an architectural pattern that separates an application into three components:
- Model: Manages the data and business logic.
- View: Displays the data to the user.
- Controller: Handles user input and updates the model and view accordingly.
95. What is JDBC, and why is it used?
JDBC is a Java API that enables Java applications to interact with databases. It provides methods to query and update data in relational databases using SQL. JDBC is used to connect to a database, send SQL statements, and process the results returned by the database.
96. What are the main components of JDBC architecture?
The main components of JDBC architecture are:
- Driver Manager: Manages a list of database drivers.
- Driver: A specific implementation for a database vendor.
- Connection: A session with a specific database.
- Statement: Used to execute SQL queries.
- ResultSet: Represents the result set of a query.
- SQLException: Handles database errors.
97. What are the different types of SQL joins?
- INNER JOIN: Returns records that are having matching values in both tables.
- LEFT (OUTER) JOIN: Returns all records from the left table and the matched records from the right table.
- RIGHT (OUTER) JOIN: Returns all records from the right table and the matched records from the left table.
- FULL (OUTER) JOIN: Returns all records when there is a match in either left or right table.
98. What is a servlet, and how does it work?
A servlet is a Java class that handles HTTP requests and responses in a web application. It extends the capabilities of servers and can respond to various types of requests, such as HTML form submissions. The servlet container (e.g., Tomcat) is responsible for managing servlet lifecycle methods like init(), service(), and destroy().
99. What is the servlet life cycle?
The servlet life cycle consists of:
- init(): Called when the servlet is first loaded.
- service(): Handles client requests. This method is called each time a request is made.
- destroy(): Called before the servlet is taken out of service.
100. What are the different types of advice in AOP?
The types of advice in AOP are:
- Before advice: Executed before a join point.
- After advice: Executed after a join point.
- Around advice: Surrounds a join point (before and after).
- After throwing advice: Runs when a method exits by throwing an exception.
After returning advice: Runs when a method completes normally.
101. What is Hibernate, and why is it used?
Hibernate is an Object-Relational Mapping (ORM) framework for Java. It simplifies data access by mapping Java objects to database tables and allows developers to interact with databases using high-level objects instead of SQL.
102. What are the main steps in a Hibernate configuration?
- Configure the Hibernate settings (in hibernate.cfg.xml or application.properties).
- Define the Java classes to map to database tables.
- Create the SessionFactory object.
- Obtain a Session object from the SessionFactory.
- Perform CRUD operations using Session.
103. What are the main features of Hibernate?
- Automatic table creation
- HQL (Hibernate Query Language)
- Transaction management
- Lazy initialization
- Caching
104. What are the main steps in a Hibernate configuration?
- Configure the Hibernate settings (in hibernate.cfg.xml or application.properties).
- Define the Java classes to map to database tables.
- Create the SessionFactory object.
- Obtain a Session object from the SessionFactory.
- Perform CRUD operations using Session.
105. What is JSP, and how is it different from servlets?
JSP is a server-side technology that allows you to create dynamic web content by embedding Java code in HTML. While servlets are Java classes with embedded HTML, JSPs are HTML pages with embedded Java code. JSP is translated into a servlet during runtime.
106. What are JSP directives?
JSP directives provide instructions to the container and are used to define page settings, include files, or declare tag libraries. The three main directives are:
- page: Defines page-dependent attributes like scripting language and error page.
- include: Includes a file at page translation time.
- taglib: Declares a tag library to be used in the page.
107. What is the Spring Framework?
Spring is a comprehensive framework for enterprise Java applications that provides infrastructure support, enabling developers to focus on the business logic. Spring core features include dependency injection, aspect-oriented programming, and transaction management.
108. What is dependency injection (DI) in Spring?
Dependency injection is a design pattern in which an object receives other objects (its dependencies) that it needs to operate. Spring supports constructor-based and setter-based dependency injection, which simplifies object creation and management.
109. What is Spring Boot, and why is it used?
Spring Boot is an extension of the Spring framework that simplifies the creation of production-ready Spring applications by reducing configuration. It provides pre-configured templates and embedded servers (like Tomcat or Jetty), making it easier to get a Spring application running quickly.
110. What is Spring MVC, and how does it work?
Spring MVC is a web framework that follows the Model-View-Controller design pattern. It separates application concerns: the model represents the data, the view displays the data, and the controller handles user input. Spring MVC also provides support for RESTful web services.
111. What is the role of @Controller and @RestController annotations in Spring MVC?
- Controller: Indicates that a class serves as a controller in Spring MVC and is used to return views.
- @RestController: Combines @Controller and @ResponseBody, indicating that the class handles RESTful requests and returns data directly as JSON or XML.
112. What is ORM, and why is it important?
ORM is a technique that maps object-oriented programming objects to relational database tables. ORM frameworks like Hibernate simplify database operations by allowing developers to work with objects rather than writing SQL queries.
113. How does Hibernate achieve ORM?
Hibernate achieves ORM by mapping Java classes to database tables through XML configuration files or annotations. It provides automatic CRUD operations, HQL (Hibernate Query Language), and session management to handle persistent data easily.
114. What is a ResultSet and how do you iterate through it?
A ResultSet is an object that holds the data retrieved from a database after executing a query. You can iterate through it using next()
while(rs.next()) {
System.out.println(rs.getString(“column_name”));
}
115. What is SQL and what are its main components?
SQL is a language used to communicate with databases. It includes:
- DDL (Data Definition Language): For creating and modifying structures (e.g., CREATE, ALTER).
- DML (Data Manipulation Language): For querying and modifying data (e.g., SELECT, INSERT, UPDATE).
- DCL (Data Control Language): For controlling access (e.g., GRANT, REVOKE).
- TCL (Transaction Control Language): For managing transactions (e.g., COMMIT, ROLLBACK).
116. Explain the difference between DDL, DML, DCL, and TCL.
- DDL: Defines and manages database schema (e.g., CREATE, ALTER).
- DML: Manipulates data in the database (e.g., SELECT, INSERT).
- DCL: Controls access to the data (e.g., GRANT, REVOKE).
- TCL: Manages transactions within the database (e.g., COMMIT, ROLLBACK).
117. How do you write a basic SELECT query?
A basic SELECT query:
SELECT column1, column2 FROM table_name WHERE condition;
118. Describe the use of SQL aggregate functions like COUNT, SUM, AVG, etc.
Aggregate functions perform calculations on a set of values and return a single value:
- COUNT(): Counts the number of rows.
- SUM(): Returns the total sum of a column.
- AVG(): Calculates the average value.
- MAX()/MIN(): Returns the largest/smallest value.
119. What is the difference between GET and POST methods?
GET: Used to retrieve data. Parameters are sent in the URL and are visible.
POST: Used to send data securely. Parameters are sent in the request body.
120. How do you handle cookies in a Servlet?
- Use HttpServletResponse.addCookie() to add cookies.
- HttpServletRequest.getCookies() to retrieve them.
121. What are the benefits of using AOP in a project?
AOP provides better code modularity, simplifies maintenance, and improves reusability by separating cross-cutting concerns from business logic.
122. Explain the difference between Session and Transaction in Hibernate.
- Session: Represents a single unit of work with the database and provides methods to perform CRUD operations.
- Transaction: Represents a unit of work that can be committed or rolled back.
123. How do you map Java classes to database tables using Hibernate?
Java classes can be mapped to database tables using XML configuration files or JPA annotations (@Entity, @Table, @Id, etc.).
124. What are the benefits of using Hibernate over JDBC?
Hibernate offers:
- Automatic table generation
- HQL for object-oriented queries
- Caching for better performance
- Lazy loading
- Simplified transaction handling
125. What is a JSP and how does it work?
JSP is a server-side technology that enables the creation of dynamic web content. It allows Java code to be embedded in HTML and is compiled into a servlet by the web server at runtime.
126. How do you embed Java code in a JSP page?
Java code is embedded in JSP using <% %> scriptlet tags or using expressions <%= %>.
127. What are the benefits of using JSP over Servlets?
JSP allows for easier creation and maintenance of web pages as it blends HTML with Java, while servlets require a lot of Java code to generate HTML.
128. What is Spring Core and why is it used?
Spring Core provides the foundation for Spring Framework, enabling dependency injection (DI) and inversion of control (IoC) for better modularity and easier testability.
129. Explain the concept of Inversion of Control (IoC).
IoC is a design principle where control over object creation is transferred from the application to the framework. In Spring, it is achieved through DI.
130. What are the benefits of using Spring Framework?
Spring provides:
- Loose coupling through DI.
- Modular architecture.
- AOP support.
- Simplified transaction management.
- Integration with various frameworks.
131. Explain the difference between ORM and JDBC.
ORM: Works with objects and automatically translates them into SQL queries. Offers caching and transaction management.
JDBC: Requires manual handling of SQL queries and result sets.
132. What are the benefits of using ORM in a project?
- Reduces boilerplate SQL code.
- Provides caching for performance.
- Simplifies transaction management.
- Supports object-oriented development with fewer manual SQL queries.