Python Programming Projects for Beginners
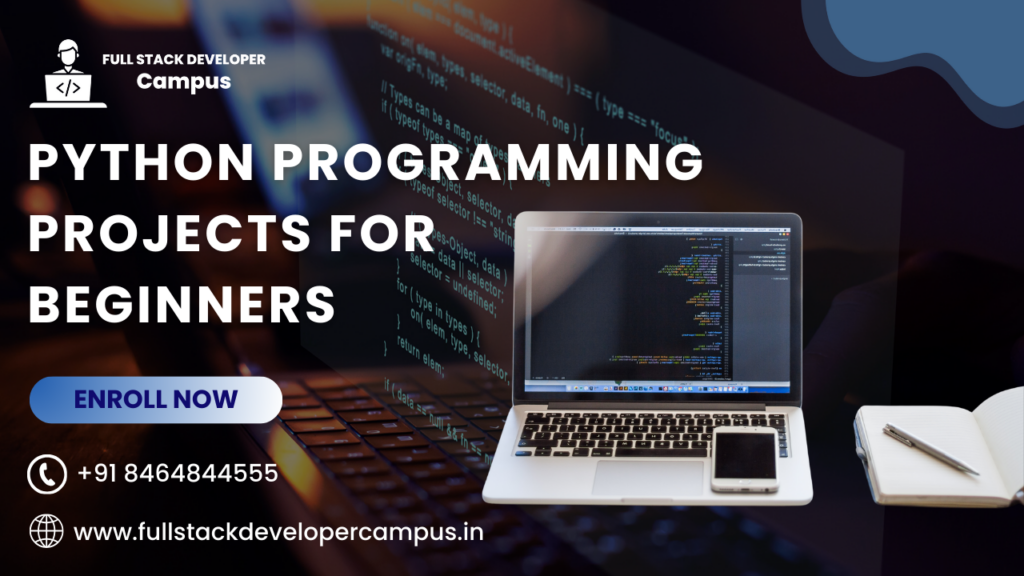
1: Introduction to Python Programming for Beginners
Python is among the most popular programming languages and is easy for beginners to learn. Whether you’re new to programming or transitioning from another language, Python provides a straightforward syntax and versatility that make it a top choice. This section will introduce you to Python’s importance in programming, its ease of use, and why it is perfect for beginners.
Python was developed in the late 1980s by Guido van Rossum with the aim to create a language that is simple, readable, and powerful. Its syntax is clear and intuitive, making it a great starting point for beginners who are learning to code.Python is also an open source language, which means it is free to use. It has a large community that helps improve and develop it.
For beginners, Python’s large standard library provides modules that allow for quick prototyping and problem-solving. Whether you want to build a web app, analyze data, or automate simple tasks, Python offers libraries and frameworks that make it easy to get started with these projects.
Why Python is Great for Beginners:
- Simple Syntax: Python uses indentation instead of curly braces or semicolons, which makes the code more readable and easier to follow.
- Versatile and Powerful: Python is not only useful for web development but also for data science, machine learning, automation, and more.
- Active Community: Python has one of the largest programming communities, meaning there are plenty of resources, tutorials, and frameworks to help you as you begin your learning journey.
2: Setting Up Python: A Step-by-Step Guide
You must install Python on your computer before you start writing any code.
This section will guide you through the installation process and the tools you’ll need to start coding in Python.
Step 1: Install Python
Start by downloading and installing Python on your computer.Follow these steps:
- Visit Python’s Official Website: Go to python.org and download the latest version of Python.
- Choose the Correct Version: For beginners, it’s recommended to download Python 3.x, as Python 2.x is outdated and no longer supported.
- Start the Installer: Once you have downloaded the installer, open it. Be sure to select the option that says “Add Python to PATH” before you click “Install Now.”
- Start the Installer: Once you have downloaded the installer, open it. Be sure to select the option that says “Add Python to PATH” before you click “Install Now.”
Step 2: Install a Code Editor
You’ll also need a text editor or Integrated Development Environment (IDE) to write your Python code. Some popular editors for Python are:
- VS Code: A lightweight but powerful code editor, highly recommended for beginners.
- PyCharm: A full-featured IDE that is great for larger projects.
- Jupyter Notebooks: Ideal for interactive coding, especially for data science projects.
Download and install your preferred editor and set it up. Once set up, you can open your editor, create a new file with a.py extension, and start coding.
Step 3: Run Your First Python Program
You have Python installed, so it’s time to create your first Python program. Open your editor and type:
print(“Hello, World!”)
Save the file and run it using your editor’s run feature or via the command line by navigating to the folder where the file is saved and typing:
python yourfile.py
You should see “Hello, World!” printed on the screen. This simple program will help you confirm that your setup is working correctly.
Why Proper Setup is Essential
Setting up your environment properly ensures that you have everything you need to write and run Python code.It also helps you fix any problems fast.Python Programming Projects For Beginners Without the right tools, you might face errors that can be difficult for beginners to understand. By taking the time to properly install Python and choose the right editor, you’ll set yourself up for a smooth coding experience.
This step-by-step guide is crucial for beginners, as it removes any barriers to getting started with coding. Once you’ve successfully set up your environment, you’re ready to dive into writing more complex Python programs.
3: Understanding Variables and Data Types in Python
In Python, variables and data types are essential concepts you need to grasp early on. A variable is simply a container that holds data, and the data type determines the kind of value it can store. In this section, we’ll explore how to work with variables and the most common data types in Python.
Variables in Python
A variable in Python is made by giving a name a valueIn Python, you don’t have to state the variable type clearly like in other programming languages. Python figures out the type on its own based on the value you provide. Here’s how you can create variables:
name = “Laddu”
age = 25
height = 5.9
is_student = True
In the example above:
- name is a string variable.
- age is an integer.
- Height is a float (a decimal number).
- is_student is a true or false value.
Common Data Types in Python
Knowing the basic data types will assist you in storing and handling data efficiently.
- Whole numbers (int): numbers that can be positive or negative and do not have decimal points.
- Example: age = 25
- Floating-Point Numbers (float): Numbers with decimals.
- Example: height = 5.9
- Strings (str) are a series of characters that are surrounded by single or double quotation marks.
- Example: name = “Laddu”
- Booleans (bool) show one of two options: true or false.
- Example: is_student = True
- Lists are organized groups of items that can include any type, such as numbers or words.
- Example: fruits = [“apple”, “banana”, “cherry”]
- Tuples (tuples): Similar to lists but immutable (cannot be changed after creation).
- Example: coordinates = (10, 20)
- Dictionaries (dict): unordered collections of key-value pairs.
- Example: person = {“name”: “Laddu”, “age”: 25}
Type Conversion
You might need to change one type of data into another at times.Python has built-in functions to change data types.
- int(): Converts a value to an integer.
- float(): Converts a value to a float.
- str(): Converts a value to a string.
For example:
num = “10” # This is a string
num = int(num) # Convert it to an integer
Why Variables and Data Types Matter
Variables and data types are fundamental to programming. They allow you to store and manipulate data, making your programs dynamic and interactive. Understanding how to use them effectively will make coding much easier and will help you write cleaner, more efficient code.
Learning the basic data types is crucial because it forms the foundation of more advanced topics in Python. Once you’re comfortable with these, you can start using them to create your own projects and build more complex programs.
4: Writing Functions in Python: Reusable Code Blocks
Functions are among the strongest features of Python. They allow you to group related code into reusable blocks, making your programs more organized, efficient, and easier to maintain. In this section, we’ll cover the basics of creating and using functions in Python.
What is a function?
A function is a block of code that executes only when you invoke it.Functions can accept inputs, known as parameters, and give back outputs. By using functions, you can write code once and reuse it multiple times, making your programs much more efficient and concise.
Here’s how you define a function in Python:
def greet(name):
print(“Hi, ” + name + “!”)
- This keyword is used to create a function in Python.
- The function is called greet.
- Parameters: The function takes one parameter, name.
- The code that is indented within the function is what the function will run.
Calling a Function
Once a function is defined, you can call it by using its name and passing any necessary arguments. For example:
greet(“Laddu”)
This will output: Hello, Laddu!
Return Values
Functions can send back values by using the return keyword. A return statement allows the function to send a result back to the caller. Here’s a sample function that gives back a value.
def add_numbers(a, b):
return a + b
result = add_numbers(10, 5)
print(result) # Output: 15
The add_numbers function calculates the sum of a and b.We can capture that result in a variable and use it in other parts of our program.
Function Parameters and Arguments
Functions can accept several parameters and work with various kinds of data.
You can also define default parameters that will be used if no argument is provided when calling the function.
For example:
def greet(name=”Guest”):
print(“Hello, ” + name + “!”)
Here, if no name is provided, it will use “Guest” as the default.
Why Functions are Important
Functions allow you to write cleaner code by reducing redundancy. Instead of repeating the same code in multiple places, you can write it once in a function and call it whenever needed. This helps make your code simpler to read, test, and keep up with.
Functions also help you break down complex problems into smaller, manageable tasks, which is essential for writing efficient and understandable code.Python Programming Projects For Beginners By mastering functions, you’ll be able to write more modular, organized, and reusable code for any project you work on.
5: Working with Loops in Python: Automating Repetitive Tasks
Loops are an essential programming concept that allow you to repeat a set of instructions multiple times. This is useful for tasks like iterating through a list or performing an action until a certain condition is met.In Python, there are two main kinds of loops: for loops and while loops.
For Loops
A for loop is used when you want to iterate over a sequence, like a list, string, or range of numbers. Here’s how it works:
fruits = [“apple”, “banana”, “cherry”]
for fruit in fruits:
print(fruit)
In this example:
- Fruits is a list.
- The for loop goes through every item in the list and displays it.
You can use a for loop along with the range() function to go through a series of numbers.For example:
for i in range(5):
print(i)
This will display numbers from 0 to 4 (5 is not part of the range).
While Loops
A while loop keeps running as long as a certain condition is true. It’s particularly useful when you don’t know beforehand how many times the loop will need to run, but you want to keep repeating an action until a condition is met.
Here it is. an example of a while loop:
count = 0
while count < 5:
print(count)
count += 1
In this case, the loop continues to run until the count becomes 5. The count += 1 part ensures that the condition will eventually be met, preventing an infinite loop.
Break and Continue
Inside loops, you can use the break and continue statements to control the flow:
- When a condition is met, it stops the loop entirely.
- Moves past the current step and goes on to the next one.
For example:
for num in range(10):
If num == 5:
When num reaches 5, leave the loop.
print(num)
This will print numbers from 0 to 4 and then exit the loop when num equals 5.
Why Loops are Important
Loops are vital for automating repetitive tasks and efficiently handling large amounts of data. Without loops, you’d have to write repetitive code for every individual task, which can be time-consuming and error-prone.Python Programming Projects For Beginners With loops, you can iterate through lists, manipulate data, or repeatedly perform actions with just a few lines of code.
Understanding how to work with loops will greatly improve your problem-solving skills and make your programs much more dynamic and flexible.
6: Working with Conditionals in Python: Making Decisions in Code
Conditionals are a fundamental aspect of programming. They enable your program to make decisions and execute specific actions based on conditions or criteria. In Python, conditionals are primarily implemented using if, elif, and else statements, which help control the flow of a program depending on logical evaluations.
The if Statement
The if statement checks a condition to see if it is trueIf the condition is met, the related code block runs. For example, you can check if someone’s age is greater than or equal to 18 and display a message if the condition holds true.
The else Statement
The else statement is used when the if statement’s condition is not true.It provides an alternative action or output. This is helpful when you want to handle the opposite case of the if statement, like indicating when someone is under a certain age.
The elif Statement
The elif statement, which stands for “else if,” lets you evaluate several conditions one after another.If the initial if condition is false, Python will evaluate the conditions in elif statements one by one. If one of them is true, the associated code block will execute.
Using Logical Operators
Logical operators like and, or, and not help combine multiple conditions in decision-making processes.
- It only gives true if both conditions are true.
- It gives a true result if at least one condition is met.
- not reverses the result of a condition.
Nested Conditionals
You can place if statements within each other to manage more complex decisions.This helps address situations where conditions depend on multiple factors, providing a structured way to manage complex logic.
Why Conditionals Are Important
Conditionals are vital for creating interactive and responsive programs.Python Programming Projects BeggBeginners They allow your application to handle varying inputs and scenarios, making it more dynamic. Whether it’s validating form data, responding to user input, or handling different states in an app, conditionals are key to building intelligent and flexible software.
7: Working with Lists in Python: Storing and Manipulating Data
Lists are among the most frequently used data structures in Python. They allow you to store a collection of items in an ordered manner, making it easy to manage multiple values in a single variable. In this section, we’ll explore how to create, manipulate, and work with lists in Python.
Creating Lists
A list is created by placing a comma-separated collection of items inside square brackets. These items can be any type of data, like numbers, text, or even other lists. Here’s an example:
fruits = [“apple”, “banana”, “cherry”]
The list of fruits includes three items. You can create a list of mixed data types as well:
person = [“Laddu”, 25, 5.9, True]
This list contains a string, an integer, a float, and a boolean value.
Accessing List Items
You can get specific items from a list using their index. Keep in mind that Python starts counting from zero, so the first item in the list is at index 0. Here’s how you can access an item from the list:
fruits = [“apple”, “banana”, “cherry”]
print(fruits[0]) # Output: apple
print(fruits[1]) # Output: banana
You can reach items from the end of the list by using negative indexing.
print(fruits[-1]) # Output: cherry
Modifying Lists
You can modify a list by changing its items, adding new items, or removing items.To modify the value of an item:
fruits[1] = “blueberry”
print(fruits) # Output: [‘apple’, ‘blueberry’, ‘cherry’]
To add something to the end of the list, use the append() method.
fruits.append(“orange”)
print(fruits) # Output: [‘apple’, ‘blueberry’, ‘cherry’, ‘orange’]
To add an item at a specific index, use the insert() method:
fruits.insert(1, “pear”)
print(fruits) # Shows: [‘apple’, ‘pear’, ‘blueberry’, ‘cherry’, ‘orange’].
To delete an item, you can use the remove() method.
fruits.remove(“blueberry”)
print(fruits) # Output: [‘apple’, ‘pear’, ‘cherry’, ‘orange’]
Looping Through Lists
You can easily loop through a list using a for loop. This is particularly useful when you want to perform an action for each item in the list:
for fruit in fruits:
print(fruit)
This will print each item in the list one by one.
List Slicing
You can slice a list to get a sublist (a portion of the list). For example:
fruits = [“apple”, “pear”, “cherry”, “orange”, “banana”]
print(fruits[1:4]) # Output: [‘pear’, ‘cherry’, ‘orange’]
Here, the slice fruits [1:4] returns a new list starting from index 1 and ending at index 3.
Why Lists are Important
Lists are incredibly useful for managing collections of data. They allow you to store multiple items in an ordered manner and provide easy ways to modify, access, and iterate over that data. Lists are versatile and can be used in a wide range of applications, from handling user inputs to storing data for analysis or processing.
Understanding how to manipulate lists is crucial for handling large datasets or when working with groups of related items. Mastering lists will help you write more efficient and organized code.
8. Introduction to Object-Oriented Programming (OOP) in Python
Object-Oriented Programming (OOP) is a programming paradigm that organizes software design by grouping related data and actions into objects. This approach helps manage complexity, especially in large programs, by promoting modularity, reusability, and maintainability. Python, with its simple syntax and rich features, is an excellent language to learn and implement OOP concepts. Mastering OOP allows developers to write efficient, clean, and scalable code.
Key Concepts of OOP
1. Classes and Objects
- Class: A class serves as a template or blueprint for creating objects.It describes the features (attributes) and functions (methods) that its objects will possess.
- An object is a specific example of a class.It represents a specific entity with unique values for its attributes.
For example, if a class is a blueprint for a “Car,” then an object is a specific car, such as a “2020 Toyota Corolla.”
2. Attributes and Methods
- Attributes are the features or qualities that define an object. They store information about the object, such as its condition or identity.
- Methods: Methods are actions or behaviors associated with an object.They explain the actions the object can perform or what can be done with it.
Think of a “dog” object that has attributes like name, breed, and age, and methods like “bark” or “fetch.”
3. Encapsulation
Encapsulation is the practice of hiding an object’s internal details and exposing only the necessary parts. This protects the data from unintended interference and misuse.
In Python, attributes can be made private to restrict direct access. Instead, controlled access is provided through specific methods. For example, a bank account might hide its balance but allow controlled deposits or withdrawals.
4. Inheritance
A class can inherit features and methods from another class.This promotes code reuse and allows for the extension of existing functionalities.
For example, if there is a generic “animal” class, a “dog” class can inherit its properties while adding its own specific features, like barking.
5. Polymorphism
Polymorphism allows different classes to use the same method name for defining specific behaviors. It supports flexibility in handling objects of different types in a uniform way.
For instance, both “dog” and “cat” classes can have a sound method, but the behavior of this method will differ for each class.
Why OOP is Essential
- Code Modularity: OOP organizes programs into manageable components, making them easier to understand and modify.
- Reusability: With features like inheritance, OOP facilitates the reuse of code, reducing redundancy.
- Ease of Maintenance: Encapsulation ensures that changes in one part of the code do not unintentionally affect other parts. This simplifies debugging and updating.
- Scalability: OOP supports building complex systems by breaking them down into smaller, reusable components, making it ideal for large-scale applications.
9. Handling Errors and Exceptions in Python: Writing Robust Code
Errors and exceptions are a common part of any programming language. In Python, exceptions occur when the interpreter encounters an error during the execution of a program. Understanding how to handle these exceptions is crucial for writing programs that are robust and can recover from unexpected situations. In this section, we’ll cover how to handle errors using try-except blocks and other related techniques in Python.
What Are Errors and Exceptions?
An error is a problem in the program that prevents it from running as expected. An exception is a specific type of error that can be caught and handled. For example, trying to divide by zero or accessing a non-existent file could result in exceptions.
Here’s an example of a program that raises an exception:
x = 10
y = 0
result = x / y # This will raise a zero-division error.
This will lead to a zero division error, which will make the program stop working.
Handling Exceptions with try-except
To prevent your program from crashing when an error occurs, you can handle exceptions using try-except blocks. Here’s an example:
try:
x = 10
y = 0
result = x / y
except ZeroDivisionError:
print(“You cannot divide by zero!”)
In this case, the except block catches the ZeroDivisionError and prints an error message instead of letting the program crash.
Catching Multiple Exceptions
You can catch multiple exceptions by using multiple except blocks:
try:
x = int(input(“Enter a number: “))
y = int(input(“Enter another number: “))
result = x / y
except ZeroDivisionError:
print(“You cannot divide by zero!”)
except ValueError:
print(“Invalid input! Please enter a valid number.”)
Here, the program handles both ZeroDivisionError (if the user tries to divide by zero) and ValueError (if the user enters a non-integer value).
Using else and finally
You can also use the else and finally blocks in error handling:
- The else block runs if no exception is raised in the try block.
- The finally block runs no matter what, even if an exception was raised or not.
Here’s an example:
try:
x = 10
y = 2
result = x / y
except ZeroDivisionError:
print(“You cannot divide by zero!”)
else:
print(“The division was successful. The result is: “Result.
finally:
print(“This block always runs.”)
In this example, the else block will execute because no exception occurred, and the finally block will always execute at the end.
Raising Exceptions
Sometimes, you may want to deliberately trigger an exception.This is done using the raise keyword. For example:
def check_age(age):
if age < 18:
raise ValueError(“You must be 18 or older.”)
else:
print(“Access granted!”)
try:
check_age(16)
except ValueError as e:
print(e) # Output: You must be 18 or older.
Here, we intentionally raise a ValueError if the user is under 18.
Why Exception Handling is Important
Exception handling allows your program to deal with unexpected situations gracefully, improving its stability and user experience. Without exception, handling errors could cause your program to crash or behave unpredictably. By catching exceptions, you can provide meaningful feedback to the user, log errors for debugging purposes, and ensure that your program continues to run smoothly even when issues arise.
Mastering exception handling is important because it makes your code more reliable and professional. You’ll be able to anticipate and handle potential problems, making your programs much more robust.
10: Working with Libraries and Modules in Python: Expanding Functionality
Python has a vast ecosystem of libraries and modules that extend its capabilities, allowing you to perform complex tasks without needing to write everything from scratch. In this section, we’ll cover the basics of importing and using libraries and modules, how to install third-party packages, and how to structure your own code into reusable modules.
What Are Libraries and Modules?
- A module is a file that holds Python code, which can include functions, classes, and variables. It can also include runnable code. You can create your own modules or you can use built-in modules or third-party libraries.
- Library: A library is a collection of modules bundled together to offer a wide range of functionality. For example, the math module is a library that includes functions for mathematical operations, and requests is a library for making HTTP requests.
Importing Built-in Modules
Python comes with many built-in modules that provide useful functions and tools. To use a module, you can import it using the import keyword.
You can use the math module to perform math operations.
import math
print(math.sqrt(16)) # Output: 4.0
Here, math.sqrt() calculates the square root of 16.
You can also import specific functions from a module to save space:
from math import sqrt
print(sqrt(25)) # Output: 5.0
Installing Third-Party Libraries
Python has a powerful package manager called pip that allows you to install third-party libraries from the Python Package Index (PyPI). For example, to install the requests library, which is commonly used for making HTTP requests, you can run the following command:
pip install requests
After installing, you can import and use it in your code:
import requests
response = requests.get(“https://api.github.com”)
print(response.status_code) # Output: 200 (for a successful request)
Creating Your Own Modules
You can create your own modules by simply saving your Python code in a.py file. For example, create a file called mymodule.py:
# mymodule.py
def greet(name):
print(f”Hello, {name}!”)
You can import and use this module in a different Python script.
import mymodule
mymodule.greet(“Laddu”) # Output: Hello, Laddu!
Using name to Make Code Reusable
To make your modules more flexible, you can use the if __name__ == “__main__”: statement. This allows you to run certain parts of your code only when the module is executed directly, not when it’s imported.
For example, in mymodule.py:
def greet(name):
print(f”Hello, {name}!”)
if __name__ == “__main__”:
greet(“World”)
Now, if you run mymodule.py directly, it will greet “World.” But if you import it into another script, it will not automatically greet anyone.
Why Libraries and Modules Are Important
Libraries and modules are essential for keeping your code clean, efficient, and easy to maintain. They allow you to avoid reinventing the wheel by providing pre-built functionality that you can leverage in your own programs. By using existing libraries, you can focus on solving the core problem of your application rather than dealing with low-level details.
Moreover, structuring your own code into reusable modules improves code organization and makes it easier to scale your projects. You can import these modules into other programs, leading to better modularity and reusability.
Learning how to use and create libraries and modules is key to writing more efficient, scalable, and maintainable Python programs.
Conclusion
In this blog, we’ve explored key concepts and essential skills that will help beginners get started with Python programming. From the basics of variables and data types to the more advanced topics of object-oriented programming, error handling, and working with libraries, each topic contributes to building a strong foundation for writing efficient and scalable Python code.
By mastering the fundamental concepts, such as working with conditionals, loops, and lists, you gain the ability to create more interactive and dynamic programs. Understanding the importance of object-oriented programming enables you to structure your code in a modular way, making it easier to maintain and extend. Meanwhile, learning how to handle exceptions ensures that your
Programs can gracefully handle errors and continue functioning under unexpected circumstances.
Additionally, the ability to work with external libraries and modules opens the door to a wealth of functionality that can help you avoid reinventing the wheel and build more powerful applications. Python’s rich ecosystem of libraries allows you to quickly integrate solutions for tasks ranging from web scraping to data analysis and machine learning.
Remember that programming is a journey, and the best way to improve your skills is through practice. As you continue to work on real-world projects and solve problems using Python, you’ll gain a deeper understanding of its capabilities and develop your own unique coding style.
By applying the concepts covered in this blog, you’ll be well on your way to becoming a proficient Python programmer, ready to take on more complex projects and dive deeper into the world of software development.
FAQs
What is Python, and why should I learn it?
Python is a high-level, interpreted programming language known for its simplicity and readability. It’s beginner-friendly and widely used in web development, data analysis, machine learning, automation, and more. Learning Python gives you access to a large ecosystem of libraries and tools, making it a versatile language for various applications.
Is Python suitable for beginners?
Yes, Python is one of the most beginner-friendly languages due to its easy-to-understand syntax and vast community support. Its focus on readability makes it an excellent choice for those just starting their programming journey.
How long does it take to learn Python?
The time to learn Python depends on your prior experience and the depth of knowledge you wish to acquire. On average, a beginner can learn the basics in 1-2 months, while mastering advanced concepts may take 6 months to a year with consistent practice.
What are the basic concepts I should learn in Python?
Beginner Python concepts include:
- Variables and data types
- Operators (arithmetic, comparison, logical)
- Control flow (if, elif, else)
- Loops (for, while)
- Functions
- Lists, tuples, and dictionaries
- Error handling with exceptions
Can Python be used for web development?
Yes, Python can be used for web development with frameworks like Django, Flask, and FastAPI. These frameworks allow you to build powerful, scalable web applications efficiently.
What are Python libraries, and why are they important?
Python libraries are pre-written code packages that provide specific functionality. Examples include Numpy for numerical computing, Pandas for data analysis, and Matplotlib for data visualization. Libraries save time by providing solutions to common problems and tasks.
What is the difference between Python 2 and Python 3?
Python 3 is the latest version and includes several improvements and optimizations over Python 2. Python 2 is no longer supported, so it is recommended to use Python 3 for all new projects.
What is Object-Oriented Programming (OOP) in Python?
Object-Oriented Programming (OOP) is a programming paradigm that organizes code into classes and objects. Python supports OOP, allowing you to define classes, create objects, and use inheritance, polymorphism, and encapsulation to structure your programs.
How do I handle errors in Python?
Errors can be handled using try, except, else, and finally blocks. This allows you to catch exceptions and prevent your program from crashing. The raise keyword can also be used to manually trigger exceptions.
What are Python functions, and how do I create them?
A function is a reusable block of code that performs a specific task. You can create functions in Python using the def keyword, followed by the function name and parameters. Functions help break down complex tasks into smaller, manageable pieces of code.
How do I install external Python libraries?
You can install external Python libraries using the pip package manager. For example, to install the requests library, run pip install requests in your command prompt or terminal.
What is the purpose of lists in Python?
A list is a collection of items stored in an ordered sequence. Lists are flexible, allowing you to store different types of data and easily modify, access, or iterate over elements. They are one of Python’s most commonly used data structures.
What is the difference between a list and a tuple in Python?
The main difference between lists and tuples is that lists are mutable (can be changed), while tuples are immutable (cannot be changed). Use tuples when you need a fixed, unchangeable collection of items.
What are some real-world applications of Python?
Python is used in a wide range of industries.
- Web development (e.g., Django, Flask)
- Data science and analysis (e.g., pandas, numpy, matplotlib)
- Machine learning and AI (e.g., TensorFlow, scikit-learn)
- Automation and scripting (e.g., automating repetitive tasks)
- Game development (e.g., Pygame)
How can I practice and improve my Python skills?
To improve your Python skills, try the following:
- Solve coding challenges on platforms like LeetCode, HackerRank, or Codewars.
- Work on personal projects that interest you.
- Contribute to open-source projects on GitHub.
- Read Python books, blogs, and tutorials to deepen your understanding.
- Join Python communities to get support and feedback.